Building Real-Time Analytics with Elixir and InfluxDB
Real-time analytics is becoming increasingly important for businesses to make timely and informed decisions. Elixir, with its concurrency and fault-tolerance features, combined with InfluxDB’s powerful time-series database capabilities, provides a robust solution for building real-time analytics applications. This blog explores how Elixir and InfluxDB can be utilized together to create effective real-time analytics systems and includes practical examples to demonstrate their integration.
Understanding Real-Time Analytics
Real-time analytics involves processing and analyzing data as it is generated, enabling immediate insights and responses. This approach is crucial for applications that require up-to-the-minute data, such as monitoring systems, financial services, and IoT solutions.
Using Elixir for Real-Time Data Processing
Elixir is a dynamic, functional language designed for building scalable and maintainable applications. Its concurrency model, based on the Actor model and Erlang’s BEAM virtual machine, makes it ideal for real-time data processing.
1. Setting Up Elixir
To start with Elixir, ensure you have it installed on your system. You can find installation instructions on the [Elixir website](https://elixir-lang.org/install.html).
2. Creating a Real-Time Data Pipeline
In this example, we’ll build a simple data pipeline to ingest and process real-time data.
Example: Simple Real-Time Data Ingestion
```elixir defmodule RealTimeProcessor do def start_link(_) do Task.start_link(fn -> process_data() end) end defp process_data do IO.puts("Starting real-time data processing...") Simulate real-time data processing loop() end defp loop do :timer.sleep(1000) IO.puts("Processing data at {:os.system_time(:seconds)}") loop() end end In your application's supervision tree children = [ {RealTimeProcessor, []} ] ```
Integrating Elixir with InfluxDB
InfluxDB is a high-performance time-series database designed for real-time analytics. It stores data in a way that makes it easy to query and visualize time-based information.
1. Setting Up InfluxDB
Install InfluxDB by following the instructions on the [InfluxDB website](https://docs.influxdata.com/influxdb/v2.0/get-started/).
2. Writing Data to InfluxDB
You can use the `influxdb-elixir` library to interact with InfluxDB from Elixir.
Example: Writing Data Points to InfluxDB
```elixir defmodule InfluxDBWriter do use InfluxDB.Client @bucket "your_bucket_name" def write_data(measurement, fields, tags \\ %{}) do influxdb_url = "http://localhost:8086" client = InfluxDB.Client.new(url: influxdb_url, token: "your_token") point = %{ measurement: measurement, fields: fields, tags: tags } case InfluxDB.Client.write(client, @bucket, point) do :ok -> IO.puts("Data written successfully") {:error, reason} -> IO.puts("Failed to write data: {reason}") end end end ```
3. Querying Data from InfluxDB
You can query time-series data using InfluxDB’s query language.
Example: Querying Recent Data Points
```elixir defmodule InfluxDBReader do use InfluxDB.Client @bucket "your_bucket_name" def read_data(query) do influxdb_url = "http://localhost:8086" client = InfluxDB.Client.new(url: influxdb_url, token: "your_token") case InfluxDB.Client.query(client, @bucket, query) do {:ok, result} -> IO.inspect(result) {:error, reason} -> IO.puts("Failed to query data: {reason}") end end end Example query to get recent data InfluxDBReader.read_data("from(bucket:\"your_bucket_name\") |> range(start: -1h)") ```
Visualizing Real-Time Data
Visualization tools like Grafana can be integrated with InfluxDB to create real-time dashboards. Grafana provides powerful features to visualize time-series data and can be connected to your InfluxDB instance to display data trends and patterns.
Example: Configuring Grafana with InfluxDB
- Install Grafana by following the instructions on the [Grafana website](https://grafana.com/docs/grafana/latest/installation/).
- Add InfluxDB as a data source in Grafana.
- Create dashboards and visualizations based on your time-series data.
Conclusion
Elixir and InfluxDB together offer a powerful combination for building real-time analytics solutions. Elixir’s capabilities for handling concurrent tasks and real-time data processing, along with InfluxDB’s efficient time-series data storage and querying, enable the creation of scalable and responsive analytics systems. Leveraging these technologies effectively can provide valuable insights and enhance decision-making capabilities.
Further Reading:
Table of Contents
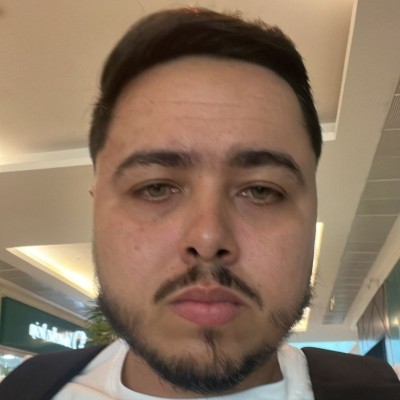
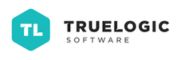