Building Real-Time Dashboards with Elixir and LiveView
In the ever-evolving landscape of web development, creating real-time dashboards that provide live updates and dynamic visualizations has become a necessity for modern applications. Elixir, known for its robust concurrency model, and LiveView, a cutting-edge feature of the Phoenix web framework, offer a potent combination for building such interactive and responsive dashboards. In this article, we’ll explore the process of building real-time dashboards using Elixir and LiveView, diving into key concepts, best practices, and hands-on code examples.
Table of Contents
1. Introduction to Real-Time Dashboards
Real-time dashboards provide users with instant access to important information and insights, making them an invaluable tool for monitoring data, analytics, and more. These dashboards need to reflect changes as they occur, offering a dynamic and interactive experience to users. Elixir, a functional programming language built for concurrency, and LiveView, a component of the Phoenix web framework, are an ideal combination for building real-time dashboards that provide these capabilities.
2. The Power of Elixir and Concurrency
At the heart of Elixir is the Erlang Virtual Machine (BEAM), which excels in managing massive numbers of lightweight processes concurrently. This architecture enables Elixir applications to handle many tasks simultaneously without the need for complex thread management. When constructing real-time dashboards, Elixir’s concurrency capabilities shine, allowing you to process and serve data to users without bottlenecks.
3. Getting Started with LiveView
3.1. Setting Up Your Project
To begin, create a new Elixir project and include the Phoenix framework. Phoenix provides the infrastructure for building web applications, and LiveView extends it with real-time capabilities.
bash # Create a new Phoenix project mix phx.new realtime_dashboard # Navigate to the project directory cd realtime_dashboard
3.2. Creating Your First LiveView Component
With your project set up, it’s time to create your first LiveView component. LiveViews represent dynamic parts of your application that update in response to server-side changes. Let’s create a basic counter component:
elixir # In lib/realtime_dashboard_web/live/counter_live.ex defmodule RealtimeDashboardWeb.CounterLive do use Phoenix.LiveView def render(assigns) do ~L""" <div> <h2>Counter: <%= @count %></h2> <button phx-click="increment">Increment</button> </div> """ end def mount(_params, session, socket) do {:ok, assign(socket, count: 0)} end def handle_event("increment", _, socket) do {:noreply, update(socket, :count, &(&1 + 1))} end end
In this example, the LiveView component displays a counter value that users can increment with the “Increment” button. The mount/3 function initializes the LiveView’s state, and the handle_event/3 function responds to user interactions.
4. Dynamic Data Integration
To create a truly useful real-time dashboard, you’ll need to integrate dynamic data. This might involve fetching data from external APIs, databases, or other sources and updating the dashboard in real time.
4.1. Fetching and Updating Data
Elixir’s built-in HTTP client libraries, such as HTTPoison, make it easy to fetch data from external sources. For example, to fetch weather data and display it in a LiveView, you might do:
elixir # In lib/realtime_dashboard_web/live/weather_live.ex defmodule RealtimeDashboardWeb.WeatherLive do use Phoenix.LiveView alias HTTPoison def render(assigns) do ~L""" <div> <h2>Weather: <%= @temperature %>°C</h2> <p>Condition: <%= @condition %></p> </div> """ end def mount(_params, _session, socket) do {:ok, socket} end def handle_info(:update_weather, socket) do case fetch_weather_data() do {:ok, data} -> temperature = data["temperature"] condition = data["condition"] {:noreply, assign(socket, temperature: temperature, condition: condition)} {:error, _} -> {:noreply, socket} end end defp fetch_weather_data do # Use HTTPoison or another HTTP client to fetch weather data end end
In this example, the handle_info/2 function fetches weather data and updates the LiveView’s state accordingly. The data is then rendered in the LiveView template.
4.2. Displaying Data in Real Time
LiveView’s automatic two-way data binding and LiveView hooks enable seamless updates. When the server-side state changes, LiveView efficiently updates the corresponding parts of the HTML, providing real-time data visualization without writing explicit JavaScript.
5. Interactive Visualizations
Adding interactive visualizations, such as charts and graphs, enhances the dashboard’s usability. You can integrate popular charting libraries like Chart.js or Google Charts.
5.1. Integrating Charting Libraries
To integrate a charting library like Chart.js, include the necessary JavaScript and CSS in your LiveView layout. Then, create a LiveView component to display the chart:
elixir # In lib/realtime_dashboard_web/live/chart_live.ex defmodule RealtimeDashboardWeb.ChartLive do use Phoenix.LiveView def render(assigns) do ~L""" <div> <canvas id="myChart" width="400" height="400"></canvas> </div> """ end def mount(_params, _session, socket) do # Initialize the chart using JavaScript, e.g., Chart.js {:ok, socket} end end
5.2. Real-Time Chart Updates with LiveView
By combining LiveView’s real-time capabilities with JavaScript charting libraries, you can achieve dynamic chart updates. When the underlying data changes, your LiveView can trigger JavaScript updates to reflect those changes in the chart.
6. User Authentication and Authorization
For many dashboards, user authentication and authorization are crucial. You want to ensure that only authorized users can access and interact with the dashboard’s sensitive information.
6.1. Securing Your Dashboard
Phoenix provides robust authentication solutions through libraries like phx_gen_auth. These libraries offer user registration, authentication, and session management, giving you a solid foundation for securing your dashboard.
6.2. Implementing User Access Control
After setting up authentication, implement access control to determine what data and features each user can access. LiveView makes it easy to conditionally render content based on user roles and permissions.
7. Optimizing for Performance
Real-time dashboards must be performant, especially when dealing with large data streams and multiple users. Here are a few tips for optimizing your dashboard’s performance:
7.1. Handling Large Data Streams
When dealing with substantial data streams, consider using Elixir’s built-in GenStage library to manage data flow efficiently. GenStage enables back pressure, ensuring that your dashboard can handle high volumes of data without overwhelming the system.
7.2. Caching and State Management
Utilize caching mechanisms to store frequently accessed data, reducing the need to fetch data from external sources repeatedly. Techniques like ETS (Erlang Term Storage) and caching libraries like Cachex can significantly improve performance.
8. Deployment and Scaling Considerations
Deploying and scaling a real-time dashboard built with Elixir and LiveView requires careful planning.
8.1. Deploying Your Dashboard
For deployment, you can use platforms like Gigalixir, which specialize in hosting Phoenix applications. Properly configure your production environment, including database connections, assets serving, and error monitoring.
8.2. Scaling with Elixir’s Fault-Tolerant Architecture
Elixir’s fault-tolerant design and process-based concurrency make it well-suited for scaling. By distributing work across multiple processes, you can ensure that your dashboard remains responsive even under heavy loads.
Conclusion
Building real-time dashboards with Elixir and LiveView opens up a world of possibilities for creating interactive, data-driven applications. Elixir’s concurrency model and LiveView’s real-time capabilities provide a solid foundation for developing powerful dashboards that offer dynamic updates, interactive visualizations, and user-friendly experiences. As you embark on your journey to create real-time dashboards, keep exploring the rich ecosystem of Elixir and Phoenix to unlock even more potential for your applications. Happy coding!
Table of Contents
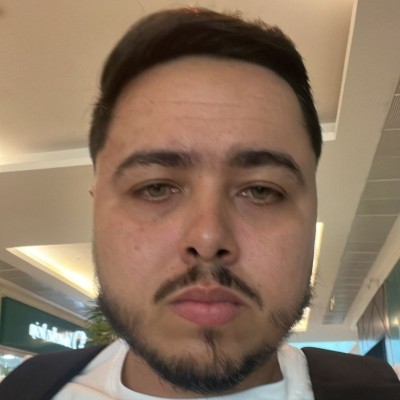
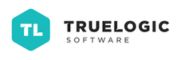