Building RESTful APIs with Elixir and Phoenix
In the ever-evolving landscape of web development, creating robust and scalable APIs is crucial for building modern applications. With the rise of functional programming languages, Elixir has emerged as a powerful choice for developing highly concurrent and fault-tolerant systems. When coupled with the Phoenix framework, developers can craft RESTful APIs that are both efficient and maintainable. In this guide, we’ll explore the process of building RESTful APIs with Elixir and Phoenix, along with some examples to illustrate key concepts.
Introduction to Elixir and Phoenix
Elixir is a dynamic, functional programming language designed for building scalable and maintainable applications. It runs on the Erlang VM (BEAM), which provides built-in support for concurrency and fault tolerance. Phoenix, on the other hand, is a web framework built with Elixir that follows the principles of performance, reliability, and developer productivity.
Getting Started with Phoenix
To begin building RESTful APIs with Elixir and Phoenix, you’ll first need to set up a new Phoenix project. You can do this using the `mix` tool, which comes bundled with Elixir. Navigate to your desired directory and run the following command:
```bash mix phx.new my_api --no-html --no-webpack ```
This command creates a new Phoenix project called `my_api` without the default HTML and webpack configurations, as we’ll be focusing solely on building APIs.
Defining Routes and Controllers
Once your project is set up, you can define routes and controllers to handle incoming HTTP requests. Phoenix uses a router to map URLs to controller actions, making it easy to define RESTful endpoints. Let’s create a simple route for a resource called `users`:
```elixir # In lib/my_api_web/router.ex defmodule MyApiWeb.Router do use MyApiWeb, :router scope "/api", MyApiWeb do pipe_through :api resources "/users", UserController end end ```
Next, we’ll create a corresponding controller to handle CRUD operations for users:
```elixir # In lib/my_api_web/controllers/user_controller.ex defmodule MyApiWeb.UserController do use MyApiWeb, :controller alias MyApi.Users def index(conn, _params) do users = Users.list_users() render(conn, "index.json", users: users) end # Implement other actions like show, create, update, and delete end ```
Serializing Data with Jason
To serialize data into JSON format, we can use the Jason library, which provides fast and flexible JSON encoding and decoding capabilities. Add Jason as a dependency in your `mix.exs` file:
```elixir # In mix.exs defp deps do [ {:phoenix, "~> 1.6.0"}, {:jason, "~> 1.2"} ] end ```
Then, run `mix deps.get` to fetch the new dependency.
Example: Creating a User Resource
Let’s walk through an example of creating a user resource using our RESTful API. Assume we have a `User` schema defined in our application. We can use HTTPie, a command-line HTTP client, to interact with our API:
```bash # Create a new user http POST http://localhost:4000/api/users name="John Doe" email="john@example.com" # Retrieve all users http GET http://localhost:4000/api/users ```
Conclusion
Building RESTful APIs with Elixir and Phoenix offers a powerful combination of performance, scalability, and developer productivity. By following the principles of functional programming and leveraging the capabilities of the Phoenix framework, developers can create APIs that are both efficient and maintainable. With Elixir’s built-in support for concurrency and fault tolerance, you can confidently build scalable applications that can handle high loads with ease.
External Resources:
- [Phoenix Framework Documentation](https://hexdocs.pm/phoenix/overview.html)
- [Elixir Lang Documentation](https://elixir-lang.org/getting-started/introduction.html)
- [Jason Library Documentation](https://hexdocs.pm/jason/readme.html)
Start building your next API with Elixir and Phoenix, and experience the benefits of functional programming in web development. Happy coding!
Table of Contents
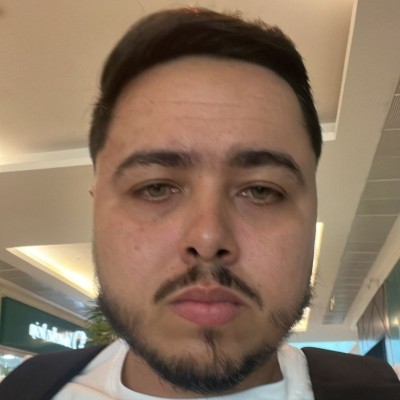
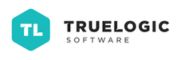