What is the syntax for defining modules in Elixir?
Defining modules in Elixir is a fundamental part of the language’s structure and organization. Modules provide a way to encapsulate functions, data, and other code elements, helping maintain a clean and structured codebase. Here’s the syntax for defining modules in Elixir:
- Module Declaration: To define a module in Elixir, you use the `defmodule` keyword followed by the module name. The module name should start with an uppercase letter and typically follows the CamelCase naming convention. For example:
```elixir defmodule MyModule do # Module contents go here end ```
In this example, we’ve defined a module named `MyModule`.
- Module Contents: The module contents are enclosed within the `do…end` block following the module declaration. This is where you define functions, variables, and other code elements that belong to the module.
- Function Definition: Most commonly, you’ll define functions within the module. To do this, use the `def` keyword followed by the function name and its arguments. The function body follows, enclosed in a `do…end` block. For example:
```elixir defmodule MathModule do def add(a, b) do a + b end end ```
In this code, we’ve defined a module named `MathModule` with an `add/2` function that takes two arguments and returns their sum.
- Exporting Functions: By default, functions defined in a module are private and can only be accessed within the module itself. To make a function accessible from outside the module, you can use the `@spec` attribute, which specifies the function’s signature and is often followed by the `@doc` attribute for documentation purposes.
```elixir defmodule MathModule do @spec add(number :: number, number :: number) :: number def add(a, b) do a + b end end ```
In this example, we’ve specified the function signature using `@spec`, allowing other modules to use the `add/2` function.
That’s the basic syntax for defining modules in Elixir. Modules play a crucial role in organizing your code, supporting encapsulation, and providing a clear structure to your Elixir applications.
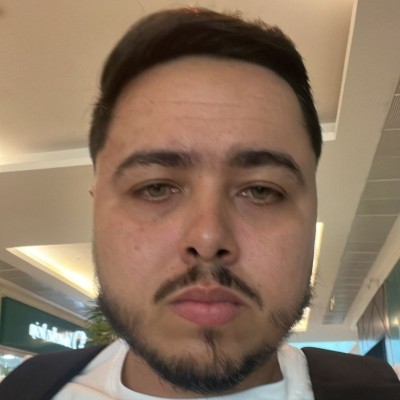
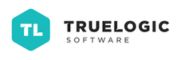