Mastering Express: A Comprehensive Guide to the Popular Node.js Framework
Express.js is a widely adopted Node.js framework that simplifies the process of building web applications. It provides a robust set of features and a flexible architecture that allows developers to create scalable and efficient server-side applications. In this comprehensive guide, we will delve into the world of Express, exploring its core concepts, advanced features, and best practices to help you become a master of this powerful framework.
Introduction to Express.js
Express.js is a minimalist web application framework for Node.js that provides a thin layer of fundamental web application functionality, allowing developers to build robust web applications quickly. It follows the middleware pattern, where incoming requests pass through a series of functions, known as middleware, before reaching the final handler.
Getting Started with Express
To get started with Express, you need to install it using npm, the Node.js package manager. Open your terminal and run the following command:
shell npm install express
Once installed, you can create an Express application by requiring the module and initializing it:
javascript const express = require('express'); const app = express();
Routing and Middleware
Express provides a powerful routing system that allows you to define routes and handle HTTP requests. You can define routes for various HTTP methods such as GET, POST, PUT, and DELETE. Here’s an example of a simple route:
javascript app.get('/users', (req, res) => { // Handle GET request for '/users' res.send('List of users'); });
Middleware functions play a crucial role in Express applications. They can be used to perform operations on the request or response objects, modify data, or handle errors. Here’s an example of a middleware function:
javascript app.use((req, res, next) => { console.log('Request received'); next(); // Call the next middleware or route handler });
Request and Response Handling
Express provides an intuitive way to handle requests and send responses. You can access request parameters, query strings, headers, and the request body. Here’s an example that demonstrates how to handle a POST request and send a JSON response:
javascript app.post('/users', (req, res) => { const user = req.body; // Save the user to the database res.json({ message: 'User created successfully' }); });
Template Engines and Views
Express supports various template engines, such as EJS and Handlebars, to generate dynamic HTML. These engines allow you to render HTML templates with embedded JavaScript code. Here’s an example of rendering an EJS template:
javascript app.set('view engine', 'ejs'); app.get('/users', (req, res) => { const users = ['John', 'Jane', 'Bob']; res.render('users', { users }); });
Working with Databases
Express can be seamlessly integrated with various databases and ORMs. You can use libraries like Sequelize or Mongoose to interact with relational or NoSQL databases, respectively.
```javascript const express = require('express'); const app = express(); // Example using Sequelize with a MySQL database const { Sequelize, DataTypes } = require('sequelize'); const sequelize = new Sequelize('database', 'username', 'password', { host: 'localhost', dialect: 'mysql', }); const User = sequelize.define('User', { name: { type: DataTypes.STRING, allowNull: false, }, }); // Example route to fetch users from the database app.get('/users', async (req, res) => { try { const users = await User.findAll(); res.json(users); } catch (error) { console.error(error); res.status(500).json({ message: 'Internal Server Error' }); } });
Error Handling and Debugging
Express provides error handling mechanisms to catch and handle errors that occur during request processing. You can use middleware functions specifically designed to handle errors and respond with appropriate error messages. Here’s an example:
```javascript app.use((err, req, res, next) => { console.error(err); res.status(500).json({ message: 'Internal Server Error' }); });
Authentication and Authorization
Securing your Express applications is crucial. You can use middleware and libraries like Passport.js to implement authentication and authorization mechanisms. Passport.js supports various authentication strategies, including local authentication, OAuth, and JWT. Here’s an example using Passport.js and local authentication:
javascript const passport = require('passport'); const LocalStrategy = require('passport-local').Strategy; passport.use( new LocalStrategy((username, password, done) => { // Check username and password against the database if (username === 'admin' && password === 'password') { return done(null, { id: 1, username: 'admin' }); } else { return done(null, false, { message: 'Invalid credentials' }); } }) ); app.post('/login', passport.authenticate('local'), (req, res) => { res.json({ message: 'Login successful' }); });
Testing Express Applications
Testing is an essential part of building reliable applications. Express applications can be tested using frameworks like Mocha and Chai. You can write unit tests, integration tests, and end-to-end tests to ensure the correctness of your application. Here’s an example of a basic unit test using Mocha and Chai:
javascript const chai = require('chai'); const chaiHttp = require('chai-http'); const expect = chai.expect; chai.use(chaiHttp); describe('GET /users', () => { it('should return a list of users', (done) => { chai .request(app) .get('/users') .end((err, res) => { expect(res).to.have.status(200); expect(res.body).to.be.an('array'); expect(res.body).to.have.length.above(0); done(); }); }); });
Scaling Express Applications
As your application grows, you might need to scale it to handle increased traffic and load. Express applications can be scaled horizontally by running multiple instances behind a load balancer. Additionally, caching mechanisms like Redis or implementing a CDN can improve performance. Monitoring and profiling tools can help identify bottlenecks and optimize performance.
Best Practices and Performance Optimization
To ensure high-quality code and optimal performance, it’s essential to follow best practices when developing Express applications. Some best practices include modularizing your code, using environment variables, implementing logging, and applying security measures. Additionally, optimizing database queries, using caching strategies,and minimizing external dependencies can greatly improve the performance of your Express application.
Conclusion
Express.js is a powerful and popular Node.js framework that simplifies web application development. In this comprehensive guide, we explored the core concepts of Express, including routing, middleware, request, and response handling. We also covered advanced topics like working with databases, authentication, error handling, testing, scaling, and performance optimization.
By mastering Express, you’ll be equipped with the knowledge and skills to build robust and scalable web applications using Node.js. Remember to follow best practices, stay updated with the latest features and developments, and continue exploring the vast ecosystem of libraries and tools that integrate seamlessly with Express.
Start your journey to becoming an Express expert today, and unleash the full potential of Node.js for web development!
Note: This blog post is intended as a comprehensive overview of Express and its features. For detailed implementation and code examples, please refer to the official Express documentation and other reliable resources.
Table of Contents
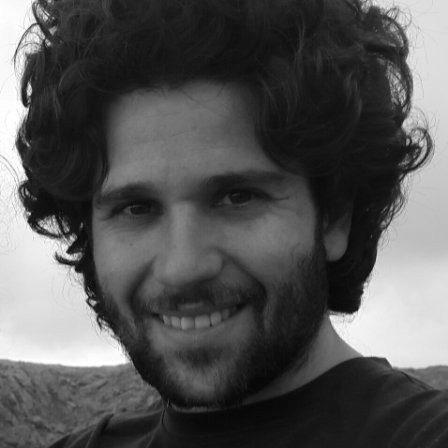
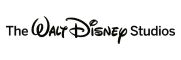