Building a Blog with Express: From Database Integration to User Authentication
Building a blog from scratch is an excellent way to learn the fundamentals of web development. Express.js, a minimal and flexible Node.js web application framework, is perfect for creating such a project. In this article, we’ll walk through the process of building a blog with Express, focusing on integrating a database and implementing user authentication. By the end of this guide, you’ll have a fully functional blog application that you can extend and customize as needed.
Setting Up the Express Application
Before diving into database integration and user authentication, you’ll need to set up the Express application.
Step 1: Initialize the Project
Start by creating a new directory for your project and initializing it with `npm`.
```bash mkdir express-blog cd express-blog npm init -y ```
Step 2: Install Dependencies
Install Express along with other necessary dependencies like `body-parser`, `mongoose`, and `express-session`.
```bash npm install express body-parser mongoose express-session ```
Step 3: Basic Server Setup
Create an `index.js` file to set up a basic Express server.
```javascript const express = require('express'); const bodyParser = require('body-parser'); const mongoose = require('mongoose'); const app = express(); app.use(bodyParser.urlencoded({ extended: true })); // MongoDB connection setup mongoose.connect('mongodb://localhost:27017/blogDB', { useNewUrlParser: true, useUnifiedTopology: true }); // Basic route app.get('/', (req, res) => { res.send('Welcome to the Express Blog!'); }); // Start the server app.listen(3000, () => { console.log('Server is running on port 3000'); }); ```
Integrating the Database
Next, you’ll integrate MongoDB, a NoSQL database, to store blog posts and user data.
Step 4: Define the Blog Schema
Create a `models` directory and define the schema for your blog posts in a new file called `Post.js`.
```javascript const mongoose = require('mongoose'); const postSchema = new mongoose.Schema({ title: String, content: String, date: { type: Date, default: Date.now } }); module.exports = mongoose.model('Post', postSchema); ```
Step 5: Create Routes for CRUD Operations
Set up routes to create, read, update, and delete blog posts.
```javascript const Post = require('./models/Post'); app.post('/posts', (req, res) => { const newPost = new Post({ title: req.body.title, content: req.body.content }); newPost.save((err) => { if (!err) { res.send('Successfully added a new post.'); } else { res.send(err); } }); }); app.get('/posts', (req, res) => { Post.find({}, (err, posts) => { if (!err) { res.json(posts); } else { res.send(err); } }); }); app.put('/posts/:id', (req, res) => { Post.updateOne( { _id: req.params.id }, { title: req.body.title, content: req.body.content }, (err) => { if (!err) { res.send('Successfully updated the post.'); } else { res.send(err); } } ); }); app.delete('/posts/:id', (req, res) => { Post.deleteOne({ _id: req.params.id }, (err) => { if (!err) { res.send('Successfully deleted the post.'); } else { res.send(err); } }); }); ```
Implementing User Authentication
User authentication is crucial for allowing users to sign in, create posts, and manage their content.
Step 6: Set Up User Authentication with Passport.js
Install Passport.js and related packages for authentication.
```bash npm install passport passport-local passport-local-mongoose express-session ```
Step 7: Define the User Schema
In the `models` directory, create a `User.js` file and define the schema for user authentication.
```javascript const mongoose = require('mongoose'); const passportLocalMongoose = require('passport-local-mongoose'); const userSchema = new mongoose.Schema({ username: String, password: String }); userSchema.plugin(passportLocalMongoose); module.exports = mongoose.model('User', userSchema); ```
Step 8: Configure Passport.js
Configure Passport.js for user authentication in your `index.js` file.
```javascript const session = require('express-session'); const passport = require('passport'); const User = require('./models/User'); app.use(session({ secret: 'yourSecretKey', resave: false, saveUninitialized: false })); app.use(passport.initialize()); app.use(passport.session()); passport.use(User.createStrategy()); passport.serializeUser(User.serializeUser()); passport.deserializeUser(User.deserializeUser()); ```
Step 9: Create Routes for User Registration and Login
Add routes to handle user registration and login.
```javascript app.post('/register', (req, res) => { User.register({ username: req.body.username }, req.body.password, (err, user) => { if (err) { res.send(err); } else { passport.authenticate('local')(req, res, () => { res.send('Successfully registered and logged in!'); }); } }); }); app.post('/login', passport.authenticate('local'), (req, res) => { res.send('Successfully logged in!'); }); app.get('/logout', (req, res) => { req.logout((err) => { if (err) { return next(err); } res.send('Successfully logged out!'); }); }); ```
Deploying the Blog
Once your blog is complete, you’ll want to deploy it to a platform like Heroku or Vercel.
Step 10: Prepare for Deployment
Ensure your application is ready for deployment by setting up a `Procfile`, configuring environment variables, and using a production database.
```bash echo "web: node index.js" > Procfile ```
Update your MongoDB connection string to use an environment variable for the database URL:
```javascript mongoose.connect(process.env.DATABASE_URL, { useNewUrlParser: true, useUnifiedTopology: true }); ```
Conclusion
Building a blog with Express.js involves integrating a database, implementing user authentication, and deploying the application. By following this guide, you should now have a functional blog that can be customized and expanded according to your needs. Express.js provides the flexibility and scalability required to develop robust web applications, making it an excellent choice for projects like this.
Further Reading:
Table of Contents
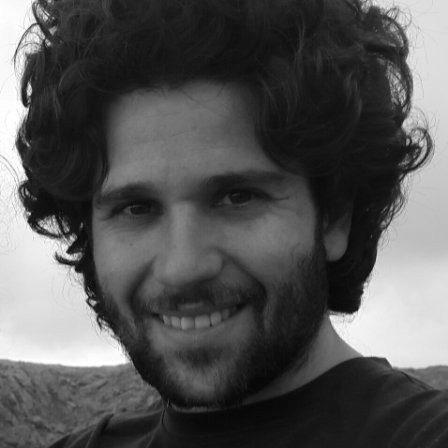
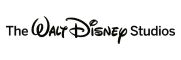