Express 101: Getting Started with the Fast and Minimalist Web Application Framework
In today’s fast-paced web development landscape, having a lightweight and efficient framework is crucial. Enter Express, a popular Node.js framework that simplifies the process of building web applications. In this article, we will explore Express and guide you through the process of getting started with this powerful framework. With code samples and step-by-step instructions, you’ll be well on your way to creating robust web applications in no time.
What is Express?
Express is a minimal and flexible web application framework for Node.js. It provides a set of features for building web applications, including routing, middleware, and template engine support. Express has gained immense popularity due to its simplicity, performance, and extensive community support.
Setting Up Express
To get started with Express, make sure you have Node.js installed on your system. You can create a new project directory and initialize it using npm. Open your terminal and run the following commands:
bash mkdir my-express-app cd my-express-app npm init -y
Next, install Express as a project dependency:
bash npm install express
Creating Your First Express Application
Now that you have Express installed, it’s time to create your first application. Create a new file named app.js and add the following code:
javascript const express = require('express'); const app = express(); app.get('/', (req, res) => { res.send('Hello, Express!'); }); const port = 3000; app.listen(port, () => { console.log(`Server running on http://localhost:${port}`); });
Save the file and run the application using the following command:
bash node app.js
You should see a message in the console indicating that the server is running. Open your web browser and navigate to http://localhost:3000. Voila! You have your first Express application up and running.
Routing in Express
Express provides a simple and intuitive way to handle different routes in your application. Let’s modify our previous example to handle different routes:
javascript app.get('/', (req, res) => { res.send('Hello, Express!'); }); app.get('/about', (req, res) => { res.send('About Page'); }); app.get('/contact', (req, res) => { res.send('Contact Page'); });
Middleware in Express
Express middleware functions are essential for processing requests before they reach the route handler. You can use middleware for tasks such as authentication, logging, error handling, and more. Let’s create a simple logging middleware:
javascript app.use((req, res, next) => { console.log(`[${new Date().toISOString()}] ${req.method} ${req.url}`); next(); });
This middleware logs the timestamp, HTTP method, and URL for every incoming request. You can place this middleware before the route definitions to ensure it gets executed for all routes.
Templating Engines in Express
Express supports various templating engines, such as EJS, Pug (formerly Jade), and Handlebars. These engines allow you to generate dynamic HTML pages. Let’s use EJS as an example:
Bash npm install ejs
In your app.js file, add the following code to set EJS as the view engine and create a simple template:
javascript app.set('view engine', 'ejs'); app.get('/hello/:name', (req, res) => { const name = req.params.name; res.render('hello', { name }); });
Create a new file named hello.ejs in a folder named views and add the following code:
html <!DOCTYPE html> <html> <head> <title>Hello, <%= name %>!</title> </head> <body> <h1>Hello, <%= name %>!</h1> </body> </html>
Now, when you navigate to http://localhost:3000/hello/John, you will see a personalized greeting.
Conclusion
Express is a powerful and versatile web application framework that allows you to build efficient and scalable web applications with ease. In this article, we covered the basics of getting started with Express, including setting up a project, creating routes, using middleware, and utilizing templating engines. Armed with this knowledge, you can now embark on your journey to create robust and feature-rich web applications using Express. Happy coding!
Table of Contents
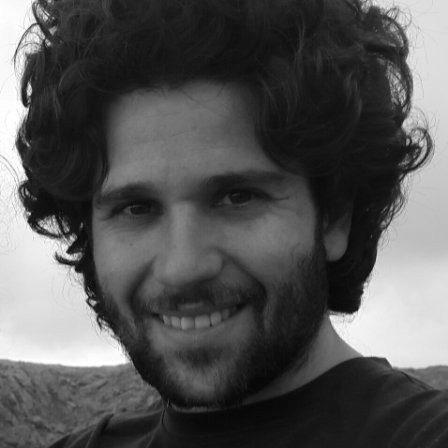
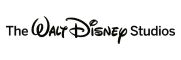