Express and MongoDB Atlas: Building Cloud-Native Applications
Cloud-native applications are designed to leverage cloud infrastructure and services, ensuring scalability, flexibility, and resilience. By using technologies like Express.js for the backend and MongoDB Atlas for database management, developers can create robust cloud-native applications that scale effortlessly.
This blog will explore how to build cloud-native applications using Express.js and MongoDB Atlas, providing practical examples and insights.
Why Choose Express.js and MongoDB Atlas?
– Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features to build single-page, multi-page, and hybrid web applications.
– MongoDB Atlas is a fully-managed cloud database service that offers global clusters, automatic scaling, and data distribution, making it an ideal choice for cloud-native applications.
Together, these tools offer an excellent foundation for developing modern, scalable applications.
Setting Up Your Environment
Before diving into coding, ensure that you have Node.js, npm (Node Package Manager), and a MongoDB Atlas account set up. You can install Express.js using npm and create a new project with the following commands:
```bash npm install express --save ```
Once installed, you can create a basic Express application structure.
1. Building a RESTful API with Express.js
Express.js simplifies the creation of RESTful APIs, which are integral to modern cloud-native applications. Here’s how you can set up a basic API endpoint using Express.js:
Example: Creating a Basic API Endpoint
```javascript const express = require('express'); const app = express(); app.use(express.json()); app.get('/api/v1/hello', (req, res) => { res.json({ message: "Hello, World!" }); }); app.listen(3000, () => { console.log('Server running on port 3000'); }); ```
This code sets up a simple API endpoint that returns a JSON response. The API can be extended to include more complex logic and routes.
2. Connecting to MongoDB Atlas
MongoDB Atlas simplifies database management in the cloud by providing automated backups, real-time analytics, and more. You can connect your Express.js application to MongoDB Atlas using the `mongoose` library.
Example: Connecting to MongoDB Atlas
```javascript const mongoose = require('mongoose'); mongoose.connect('mongodb+srv://<username>:<password>@cluster.mongodb.net/myFirstDatabase?retryWrites=true&w=majority', { useNewUrlParser: true, useUnifiedTopology: true, }); const db = mongoose.connection; db.on('error', console.error.bind(console, 'connection error:')); db.once('open', () => { console.log('Connected to MongoDB Atlas'); }); ```
Replace `<username>`, `<password>`, and `myFirstDatabase` with your MongoDB Atlas credentials and database name.
3. Defining Mongoose Models
Mongoose is an ODM (Object Data Modeling) library for MongoDB and Node.js, providing a straightforward schema-based solution to model your application data.
Example: Defining a Mongoose Schema and Model
```javascript const mongoose = require('mongoose'); const userSchema = new mongoose.Schema({ name: String, email: String, createdAt: { type: Date, default: Date.now } }); const User = mongoose.model('User', userSchema); ```
This example defines a simple `User` model with a name, email, and creation date.
4. CRUD Operations with Express and MongoDB Atlas
Once the model is defined, you can easily implement CRUD (Create, Read, Update, Delete) operations in your Express.js application.
Example: Implementing CRUD Operations
```javascript // Create a new user app.post('/api/v1/users', async (req, res) => { const user = new User(req.body); await user.save(); res.status(201).json(user); }); // Get all users app.get('/api/v1/users', async (req, res) => { const users = await User.find(); res.json(users); }); // Update a user by ID app.put('/api/v1/users/:id', async (req, res) => { const user = await User.findByIdAndUpdate(req.params.id, req.body, { new: true }); res.json(user); }); // Delete a user by ID app.delete('/api/v1/users/:id', async (req, res) => { await User.findByIdAndDelete(req.params.id); res.status(204).send(); }); ```
These endpoints allow you to create, retrieve, update, and delete user data stored in MongoDB Atlas.
5. Deploying Your Cloud-Native Application
After building your Express.js application, the final step is deploying it to the cloud. Platforms like Heroku, AWS, and Azure offer easy integration with MongoDB Atlas, making deployment seamless.
Example: Deploying to Heroku
- Install the Heroku CLI and log in:
```bash heroku login ```
- Create a new Heroku app:
```bash Heroku create ```
- Deploy your application:
```bash git push heroku main ```
Heroku will automatically detect your Node.js application and deploy it, connecting it to MongoDB Atlas.
Conclusion
Building cloud-native applications with Express.js and MongoDB Atlas allows developers to create scalable, resilient, and flexible solutions. By leveraging these powerful tools, you can focus on writing code while the cloud takes care of the infrastructure.
With the examples provided, you can start building your own cloud-native applications that are ready to scale.
Further Reading:
Table of Contents
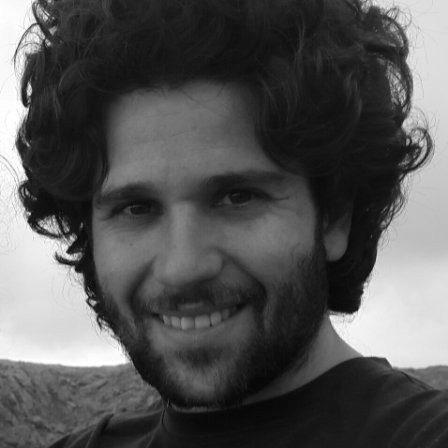
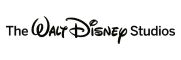