Building a RESTful API with Express and Sequelize
Building a RESTful API is a common task for modern web development, allowing applications to interact with data in a structured way. Express, a popular web framework for Node.js, combined with Sequelize, an ORM (Object-Relational Mapping) for Node.js, provides a powerful toolkit for creating APIs that handle complex data interactions efficiently. This blog will guide you through the process of building a RESTful API using Express and Sequelize, with practical examples and code snippets.
Understanding RESTful APIs
A RESTful API (Representational State Transfer) allows communication between clients and servers using standard HTTP methods. It facilitates CRUD (Create, Read, Update, Delete) operations and provides a scalable way to manage data.
Setting Up Your Environment
Before diving into code, you need to set up your environment. Ensure you have Node.js and npm (Node Package Manager) installed. You’ll also need to install Express and Sequelize along with their dependencies.
```bash npm install express sequelize pg pg-hstore ```
– Express: Web framework for Node.js.
– Sequelize: ORM for Node.js that supports multiple SQL databases.
– pg and pg-hstore: PostgreSQL and its serializer.
Building the API with Express and Sequelize
1. Setting Up the Express Server
First, create a basic Express server that listens for requests.
```javascript // server.js const express = require('express'); const app = express(); const port = 3000; app.use(express.json()); // Middleware to parse JSON bodies app.get('/', (req, res) => { res.send('Welcome to the API!'); }); app.listen(port, () => { console.log(`Server running at http://localhost:${port}/`); }); ```
2. Configuring Sequelize
Initialize Sequelize to connect to your database and define models.
```javascript // models/index.js const { Sequelize } = require('sequelize'); const sequelize = new Sequelize('database', 'username', 'password', { host: 'localhost', dialect: 'postgres', }); const User = sequelize.define('User', { name: { type: Sequelize.STRING, allowNull: false, }, email: { type: Sequelize.STRING, unique: true, allowNull: false, }, }); sequelize.sync(); // Sync models with the database module.exports = { sequelize, User }; ```
3. Implementing CRUD Operations
Add routes to your Express server to handle CRUD operations for the User model.
```javascript // routes/users.js const express = require('express'); const router = express.Router(); const { User } = require('../models'); // Create a new user router.post('/', async (req, res) => { try { const user = await User.create(req.body); res.status(201).json(user); } catch (error) { res.status(400).json({ error: error.message }); } }); // Get all users router.get('/', async (req, res) => { try { const users = await User.findAll(); res.status(200).json(users); } catch (error) { res.status(500).json({ error: error.message }); } }); // Get a user by ID router.get('/:id', async (req, res) => { try { const user = await User.findByPk(req.params.id); if (user) { res.status(200).json(user); } else { res.status(404).json({ error: 'User not found' }); } } catch (error) { res.status(500).json({ error: error.message }); } }); // Update a user by ID router.put('/:id', async (req, res) => { try { const user = await User.findByPk(req.params.id); if (user) { await user.update(req.body); res.status(200).json(user); } else { res.status(404).json({ error: 'User not found' }); } } catch (error) { res.status(400).json({ error: error.message }); } }); // Delete a user by ID router.delete('/:id', async (req, res) => { try { const user = await User.findByPk(req.params.id); if (user) { await user.destroy(); res.status(204).end(); } else { res.status(404).json({ error: 'User not found' }); } } catch (error) { res.status(500).json({ error: error.message }); } }); module.exports = router; ```
Integrate the routes into your Express server.
```javascript // server.js (continued) const userRoutes = require('./routes/users'); app.use('/users', userRoutes); ```
Testing Your API
Use tools like Postman or CURL to test the endpoints you’ve created. Check for successful creation, retrieval, updating, and deletion of user records.
Conclusion
Building a RESTful API with Express and Sequelize is a powerful way to manage data and provide a scalable service for your applications. Express offers a lightweight framework for handling requests, while Sequelize provides a robust ORM for interacting with your database. Together, they create a seamless environment for developing and managing APIs.
Further Reading:
Table of Contents
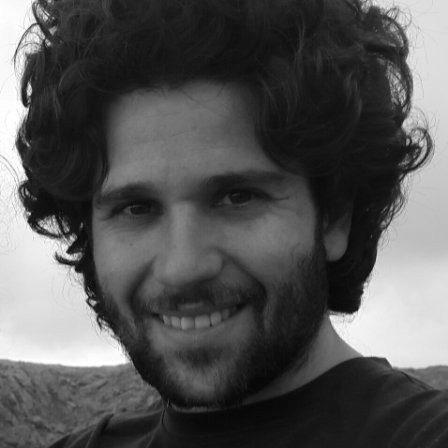
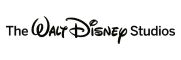