Scaling Express Applications: Techniques and Considerations
As your Express application gains popularity and user base, it’s essential to ensure that your app can handle increasing traffic and maintain optimal performance. Scaling an Express application involves implementing techniques and considering various factors to enhance its scalability, reliability, and efficiency. In this blog post, we will explore some valuable techniques and considerations for scaling Express applications, along with code samples to help you optimize your app effectively.
Load Balancing
Load balancing is a crucial technique to distribute incoming network traffic across multiple servers or processes. By distributing the workload, load balancing helps improve application availability, scalability, and reliability. One popular load balancing technique is using a reverse proxy server like Nginx or HAProxy. Here’s an example of an Nginx configuration for load balancing:
nginx http { upstream express_servers { server app1.example.com; server app2.example.com; server app3.example.com; } server { listen 80; server_name example.com; location / { proxy_pass http://express_servers; } } }
Horizontal Scaling
Horizontal scaling involves adding more servers or instances to your application infrastructure to handle increased traffic. Express applications can be horizontally scaled by deploying multiple instances behind a load balancer. Each instance can handle a portion of the incoming requests, allowing for better utilization of resources and improved performance. Consider using containerization technologies like Docker and orchestration tools like Kubernetes to manage the scaling process efficiently.
Caching
Caching is an effective technique to reduce the load on your Express application’s backend by storing frequently accessed data in a cache. Implementing a caching layer can significantly improve response times and reduce database or API calls. One popular caching solution is Redis, an in-memory data structure store. Here’s an example of using Redis as a cache with Express:
javascript const redis = require('redis'); const client = redis.createClient(); app.get('/data/:id', (req, res) => { const { id } = req.params; client.get(id, (err, data) => { if (data) { res.send(data); } else { // Fetch data from the database // Store it in Redis cache for future requests } }); });
Database Optimization
As traffic increases, database performance becomes crucial for the overall application scalability. Consider the following optimization techniques:
1. Indexing
Proper indexing of frequently queried fields in your database can significantly improve query performance. Analyze your application’s query patterns and add indexes to columns frequently used in WHERE, JOIN, or ORDER BY clauses.
2. Connection Pooling
Using connection pooling libraries like pg-pool (for PostgreSQL) or mysql2 (for MySQL) allows reusing database connections, eliminating the overhead of creating a new connection for each request.
javascript const { Pool } = require('pg'); const pool = new Pool(); app.get('/data', async (req, res) => { const client = await pool.connect(); try { // Perform database operations res.send(result); } finally { client.release(); } });
Asynchronous Operations
Leveraging asynchronous programming can improve your application’s responsiveness and scalability. By avoiding blocking operations, you can handle more concurrent requests efficiently. Use tools like async/await or Promises to handle asynchronous operations in Express.
javascript app.get('/data', async (req, res) => { try { const result = await fetchData(); res.send(result); } catch (error) { res.status(500).send('An error occurred'); } });
Performance Monitoring
Monitoring the performance of your Express application is essential to identify bottlenecks and optimize its performance. Utilize performance monitoring tools like New Relic, Datadog, or the built-in Node.js Performance API to track response times, CPU and memory usage, and other vital metrics.
Conclusion
Scaling an Express application involves implementing various techniques and considering factors such as load balancing, horizontal scaling, caching, database optimization, asynchronous operations, and performance monitoring. By following these techniques and best practices, you can ensure that your Express application handles increasing traffic with efficiency, maintains high availability, and provides a seamless user experience. Optimize your app using the code samples provided and keep monitoring its performance to continue improving scalability as your user base grows.
Table of Contents
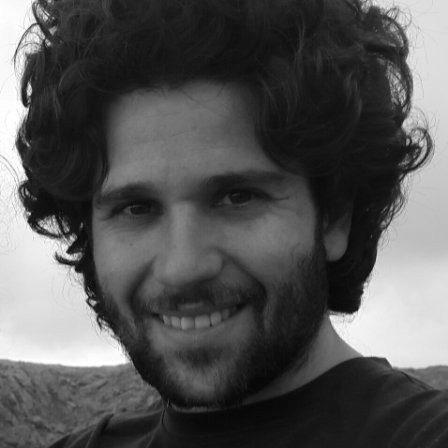
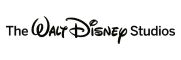