Express and SQL: Working with Relational Databases
Relational databases form the backbone of many modern web applications, and knowing how to work with them effectively is essential for any developer. Express.js, a popular web framework for Node.js, combined with SQL, offers a powerful way to manage and manipulate relational data. This blog explores how Express.js can be used to interact with SQL databases, providing practical examples and best practices.
Understanding Relational Databases
Relational databases store data in tables, where each table is a collection of rows, and each row contains fields corresponding to the columns of the table. SQL (Structured Query Language) is the standard language used to query and manipulate this data. Common relational databases include MySQL, PostgreSQL, and SQLite.
Using Express.js for Database Management
Express.js is a flexible and minimal web framework for Node.js that simplifies the creation of web applications and APIs. When combined with SQL, Express.js can be used to build full-featured web applications with robust database interactions. Below are some key concepts and examples demonstrating how Express.js can work with SQL databases.
1. Setting Up the Database Connection
The first step in working with SQL in an Express.js application is to set up the database connection. This can be done using a database client such as `pg` for PostgreSQL, `mysql` for MySQL, or `sqlite3` for SQLite.
Example: Connecting to a PostgreSQL Database
```javascript const express = require('express'); const { Pool } = require('pg'); const app = express(); const pool = new Pool({ user: 'dbuser', host: 'localhost', database: 'mydatabase', password: 'password', port: 5432, }); app.get('/', async (req, res) => { try { const result = await pool.query('SELECT FROM mytable'); res.json(result.rows); } catch (err) { console.error(err); res.status(500).send('Server Error'); } }); app.listen(3000, () => { console.log('Server running on port 3000'); }); ```
2. Querying the Database
Once the connection is established, you can start querying the database. SQL queries allow you to retrieve, insert, update, and delete data from your tables.
Example: Retrieving Data from the Database
```javascript app.get('/users', async (req, res) => { try { const result = await pool.query('SELECT FROM users'); res.json(result.rows); } catch (err) { console.error(err); res.status(500).send('Error retrieving users'); } }); ```
Example: Inserting Data into the Database
```javascript app.post('/users', async (req, res) => { const { name, email } = req.body; try { const result = await pool.query( 'INSERT INTO users (name, email) VALUES ($1, $2) RETURNING ', [name, email] ); res.json(result.rows[0]); } catch (err) { console.error(err); res.status(500).send('Error inserting user'); } }); ```
3. Handling Complex Queries
In real-world applications, you’ll often need to perform complex queries involving joins, aggregations, and subqueries.
Example: Joining Tables
```javascript app.get('/orders', async (req, res) => { try { const result = await pool.query(` SELECT orders.id, users.name, products.title FROM orders JOIN users ON orders.user_id = users.id JOIN products ON orders.product_id = products.id `); res.json(result.rows); } catch (err) { console.error(err); res.status(500).send('Error retrieving orders'); } }); ```
4. Using ORM for Simplified Database Interaction
Object-Relational Mapping (ORM) libraries like `Sequelize` or `TypeORM` can further simplify database operations by allowing you to interact with your database using JavaScript objects instead of raw SQL queries.
Example: Using Sequelize with Express
```javascript const { Sequelize, DataTypes } = require('sequelize'); const sequelize = new Sequelize('sqlite::memory:'); const User = sequelize.define('User', { name: { type: DataTypes.STRING, allowNull: false, }, email: { type: DataTypes.STRING, allowNull: false, }, }); app.get('/users', async (req, res) => { try { const users = await User.findAll(); res.json(users); } catch (err) { console.error(err); res.status(500).send('Error retrieving users'); } }); ```
5. Error Handling and Security Considerations
When working with databases, it’s crucial to handle errors properly and secure your application against common vulnerabilities such as SQL injection.
Example: Preventing SQL Injection
Using parameterized queries, as shown in the previous examples, is one way to protect against SQL injection. This approach ensures that user inputs are treated as data, not executable code.
Conclusion
Integrating Express.js with SQL databases allows developers to build robust web applications with efficient data management capabilities. Whether you’re working with raw SQL queries or leveraging ORM libraries, understanding how to interact with relational databases is a key skill in modern web development. By following best practices and securing your application against common threats, you can ensure the integrity and performance of your database-driven applications.
Further Reading:
Table of Contents
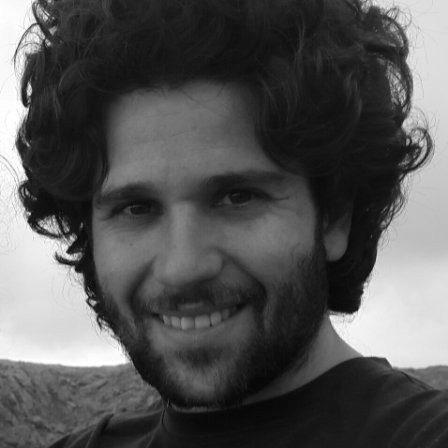
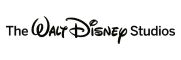