Express vs. Other Node.js Frameworks: Which One to Choose?
Choosing the right Node.js framework is crucial for building robust and scalable web applications. While Node.js itself provides a solid foundation, various frameworks add additional features and functionalities to streamline development. One of the most popular choices is Express, but there are other contenders in the Node.js ecosystem as well. In this blog post, we’ll compare Express with other notable Node.js frameworks, exploring their features, benefits, and code samples to help you make an informed decision.
Express.js: A Quick Overview
Express is a fast, minimal, and flexible Node.js web application framework that provides a robust set of features for building web applications and APIs. It offers an unopinionated approach, allowing developers to make their own architectural decisions. With a vast ecosystem of middleware, Express simplifies tasks like routing, handling HTTP requests and responses, managing sessions, and much more. Its simplicity and ease of use have made it the de facto standard for many Node.js developers.
Comparing Express with Other Node.js Frameworks
1. Koa.js
Koa.js is a lightweight Node.js framework developed by the same team behind Express. It focuses on modularity and extensibility, providing a more elegant and expressive API. Koa adopts modern JavaScript features like async/await, making it highly readable and maintaining a clean codebase. It offers advanced features like context passing, which facilitates request-specific state management. However, Koa’s ecosystem and community support are not as extensive as Express.
2. Hapi.js
Hapi.js is another popular Node.js framework known for its configuration-centric approach. It emphasizes code reusability and scalability, making it suitable for large-scale applications. Hapi.js offers features like input validation, authentication, caching, and plugin support out of the box. Its powerful routing system allows developers to define routes with ease. While Hapi.js provides excellent performance and extensive documentation, its learning curve might be steeper compared to Express.
3. Sails.js
Sails.js is a full-featured MVC framework built on top of Express. It follows the convention over configuration principle, aiming for a developer-friendly experience. Sails.js provides automatic route generation, database integration, and real-time capabilities through WebSockets. It promotes the use of Waterline, an ORM/ODM that supports multiple databases. Sails.js is suitable for building real-time applications and RESTful APIs but may introduce unnecessary complexity for smaller projects.
Performance and Scalability
When it comes to performance and scalability, Express has proven its worth over the years, with numerous optimizations and a vast community contributing to its efficiency. However, other frameworks like Koa, Hapi, and Sails also offer impressive performance benchmarks. The choice ultimately depends on the specific needs of your project, the expertise of your development team, and the scalability requirements.
Code Samples
1. Express.js Code Sample
javascript const express = require('express'); const app = express(); app.get('/', (req, res) => { res.send('Hello, World!'); }); app.listen(3000, () => { console.log('Express server listening on port 3000'); });
2. Koa.js Code Sample
javascript const Koa = require('koa'); const app = new Koa(); app.use(async (ctx) => { ctx.body = 'Hello, World!'; }); app.listen(3000, () => { console.log('Koa server listening on port 3000'); });
3. Hapi.js Code Sample
javascript const Hapi = require('@hapi/hapi'); const init = async () => { const server = Hapi.server({ port: 3000, host: 'localhost', }); server.route({ method: 'GET', path: '/', handler: (request, h) => { return 'Hello, World!'; }, }); await server.start(); console.log('Hapi server listening on port 3000'); }; init();
4. Sails.js Code Sample
javascript const sails = require('sails'); const http = require('http'); const app = sails(); app.lift(http.createServer(app), (err) => { if (err) { console.error(err); } else { console.log('Sails server listening on port ' + app.config.port); } });
Conclusion
Choosing the right Node.js framework depends on your project’s requirements, team expertise, and personal preferences. Express remains the go-to choice for many developers due to its simplicity, vast ecosystem, and community support. However, frameworks like Koa, Hapi, and Sails offer unique features and cater to different development styles. Consider factors such as modularity, extensibility, scalability, and learning curve when making your decision. Experiment with code samples, explore documentation, and gather insights from the Node.js community to select the framework that best fits your needs.
Table of Contents
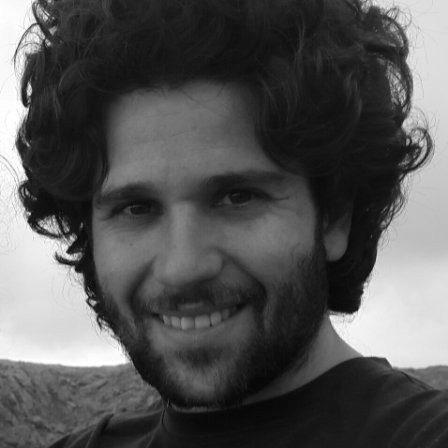
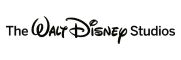