How do you handle background tasks in Flutter?
Handling background tasks in Flutter involves executing tasks that don’t interfere with the main thread, ensuring a smooth user experience. One common approach is to use the `flutter_background_service` package to manage background execution.
To implement background tasks, you first define a background task handler using the package. This handler typically extends the `BackgroundService` class and overrides the `onStart`, `onStop`, and `onBackgroundTask` methods. The `onBackgroundTask` method contains the code to be executed in the background.
Example:
```dart import 'package:flutter_background_service/flutter_background_service.dart'; class MyBackgroundTask extends BackgroundService { @override Future<void> onStart() async { print('Background task started'); // Initialization code if needed } @override Future<void> onStop() async { print('Background task stopped'); // Cleanup code if needed } @override void onBackgroundTask() { print('Executing background task'); // Your background task logic here } } void main() { WidgetsFlutterBinding.ensureInitialized(); BackgroundService.init(); BackgroundService().start(MyBackgroundTask()); runApp(MyApp()); } ```
In this example, `MyBackgroundTask` defines the background task logic in the `onBackgroundTask` method. The background task starts when the app initializes, and you can customize it to perform tasks like fetching data, processing updates, or handling notifications.
Remember to include the necessary permissions and configurations in the AndroidManifest.xml and Info.plist files for Android and iOS, respectively.
This approach allows Flutter apps to execute tasks in the background efficiently, enhancing the overall user experience by preventing the main thread from being blocked during resource-intensive operations.
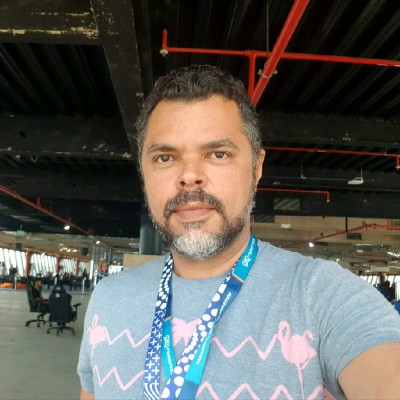
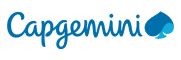