Building a Car Rental App with Flutter: Maps and Booking
In today’s fast-paced world, convenience is key, and that extends to transportation as well. Car rental apps have become an integral part of modern travel, providing users with the flexibility to rent a car on-the-go. If you’re looking to create your own car rental app, Flutter is an excellent choice. With its robust framework and versatile widget library, you can build a feature-rich car rental app that includes maps and booking capabilities. In this comprehensive guide, we’ll walk you through the process of building a car rental app with Flutter, covering everything from setting up the project to integrating maps and implementing booking functionality.
Table of Contents
1. Prerequisites
Before we dive into the development process, let’s make sure you have everything you need to get started:
1.1. Flutter Development Environment
Make sure you have Flutter installed on your system. If you haven’t already, follow the official installation guide on the Flutter website.
bash flutter --version
This command should display the installed Flutter version, confirming that you’re ready to proceed.
1.2. Code Editor
Choose a code editor that you’re comfortable with. Popular choices include Visual Studio Code, Android Studio, and IntelliJ IDEA. Ensure that you have the Flutter and Dart extensions installed for your chosen editor.
1.3. Google Maps API Key
To integrate maps into your app, you’ll need a Google Maps API Key. Follow Google’s documentation to obtain one.
1.4. Firebase Account
For user authentication and data storage, we’ll be using Firebase. Create a Firebase account and set up a new project.
Now that you have the necessary prerequisites in place, let’s begin building our car rental app with Flutter.
2. Setting Up the Flutter Project
Start by creating a new Flutter project using the following command:
bash flutter create car_rental_app
Navigate to your project directory:
bash cd car_rental_app
Open the project in your chosen code editor. You’re now ready to start developing your car rental app.
3. Designing the User Interface
3.1. Landing Page
Let’s begin by designing the landing page of your car rental app. This page should display a list of available cars and their details, along with a map showing the user’s current location.
Sample Landing Page Code:
dart import 'package:flutter/material.dart'; class LandingPage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Car Rental App'), ), body: Column( children: [ Text('Available Cars'), // Display a list of available cars here // Display a map showing user's current location ], ), ); } }
3.2. Car Details Page
Create a separate page for displaying detailed information about a selected car. Users should be able to view images, specifications, and book the car from this page.
Sample Car Details Page Code:
dart import 'package:flutter/material.dart'; class CarDetailsPage extends StatelessWidget { // Pass the car details as arguments to this page final Car car; CarDetailsPage({required this.car}); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(car.name), ), body: Column( children: [ Image.network(car.imageURL), Text(car.name), Text('Specifications: ${car.specifications}'), // Add a button to book the car ], ), ); } }
3.3. Booking Confirmation Page
Create a page where users can confirm their booking details before finalizing their reservation.
Sample Booking Confirmation Page Code:
dart import 'package:flutter/material.dart'; class BookingConfirmationPage extends StatelessWidget { // Pass booking details as arguments to this page final BookingDetails bookingDetails; BookingConfirmationPage({required this.bookingDetails}); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Booking Confirmation'), ), body: Column( children: [ Text('Confirmation Details'), Text('Car: ${bookingDetails.car.name}'), Text('Pickup Date: ${bookingDetails.pickupDate}'), Text('Return Date: ${bookingDetails.returnDate}'), // Add a button to confirm the booking ], ), ); } }
4. Integrating Google Maps
4.1. Installing Dependencies
To integrate Google Maps into your Flutter app, you’ll need to use the google_maps_flutter package. Add it to your pubspec.yaml file:
yaml dependencies: flutter: sdk: flutter google_maps_flutter: ^2.0.4 # Check for the latest version on pub.dev
Run ‘flutter pub get’ to fetch the package.
4.2. Setting Up Google Maps
Now, let’s integrate Google Maps into your app. Create a new file called maps.dart and add the following code:
maps.dart
dart import 'package:flutter/material.dart'; import 'package:google_maps_flutter/google_maps_flutter.dart'; class MapPage extends StatefulWidget { @override _MapPageState createState() => _MapPageState(); } class _MapPageState extends State<MapPage> { late GoogleMapController mapController; final LatLng _center = const LatLng(37.7749, -122.4194); // Default center final Set<Marker> _markers = {}; // Markers on the map void _onMapCreated(GoogleMapController controller) { mapController = controller; } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Maps'), ), body: GoogleMap( onMapCreated: _onMapCreated, initialCameraPosition: CameraPosition( target: _center, zoom: 10.0, ), markers: _markers, ), ); } }
4.3. Displaying Maps on Landing Page
Now, let’s integrate the MapPage into your landing page.
Landing Page Code (Updated):
dart import 'package:flutter/material.dart'; class LandingPage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Car Rental App'), ), body: Column( children: [ Text('Available Cars'), // Display a list of available cars here ElevatedButton( onPressed: () { Navigator.push( context, MaterialPageRoute(builder: (context) => MapPage()), ); }, child: Text('View Map'), ), ], ), ); } }
With this setup, users can now click the “View Map” button to access the map page.
5. Implementing Booking Functionality
To complete your car rental app, you need to implement booking functionality. We’ll use Firebase to store user data and booking information.
5.1. Firebase Setup
Add Firebase to your project by following these steps:
- Go to the Firebase Console (https://console.firebase.google.com/).
- Click “Add Project” and follow the setup wizard.
- Once your project is created, click “Add app” and select the appropriate platform (iOS or Android).
- Follow the instructions to download the configuration files and add them to your Flutter project.
5.2. User Authentication
Implement user authentication with Firebase. You can use Firebase Authentication to allow users to sign up and log in to your app.
dart import 'package:firebase_auth/firebase_auth.dart'; // Sign up a user Future<void> signUp(String email, String password) async { try { await FirebaseAuth.instance.createUserWithEmailAndPassword( email: email, password: password, ); } catch (e) { print('Error signing up: $e'); } } // Log in a user Future<void> logIn(String email, String password) async { try { await FirebaseAuth.instance.signInWithEmailAndPassword( email: email, password: password, ); } catch (e) { print('Error logging in: $e'); } }
5.3. Firestore Database
Set up Firebase Firestore to store car data and user bookings. Create a Firestore collection for cars and another for bookings.
5.3.1. Car Data Model:
dart class Car { final String id; final String name; final String imageURL; final String specifications; Car({ required this.id, required this.name, required this.imageURL, required this.specifications, }); }
5.3.2. Booking Data Model:
dart class BookingDetails { final Car car; final DateTime pickupDate; final DateTime returnDate; BookingDetails({ required this.car, required this.pickupDate, required this.returnDate, }); }
5.3.3. Adding a Car to Firestore:
dart import 'package:cloud_firestore/cloud_firestore.dart'; Future<void> addCar(Car car) async { await FirebaseFirestore.instance.collection('cars').doc(car.id).set({ 'name': car.name, 'imageURL': car.imageURL, 'specifications': car.specifications, }); }
5.3.4. Booking a Car and Storing Details in Firestore:
dart Future<void> bookCar(BookingDetails bookingDetails) async { await FirebaseFirestore.instance.collection('bookings').add({ 'car_id': bookingDetails.car.id, 'pickup_date': bookingDetails.pickupDate, 'return_date': bookingDetails.returnDate, // Add user ID here to associate the booking with the user }); }
With these Firebase services in place, you can now enable users to sign up, log in, view available cars, book a car, and store their booking details securely.
Conclusion
Congratulations! You’ve learned how to build a car rental app with Flutter, complete with maps and booking functionality. This comprehensive guide covered setting up your Flutter project, designing the user interface, integrating Google Maps, and implementing booking functionality using Firebase. With the right features and a user-friendly interface, your car rental app has the potential to provide a convenient and efficient solution for travelers. As you continue to develop and refine your app, consider adding additional features such as payment processing and user reviews to enhance the user experience further. Happy coding!
In the ever-evolving world of mobile app development, Flutter remains a versatile and powerful framework for building cross-platform applications. Whether you’re a seasoned developer or just getting started, Flutter provides a user-friendly and efficient platform for turning your app ideas into reality. So, go ahead and embark on your journey to create a cutting-edge car rental app and bring convenience to travelers worldwide.
Table of Contents
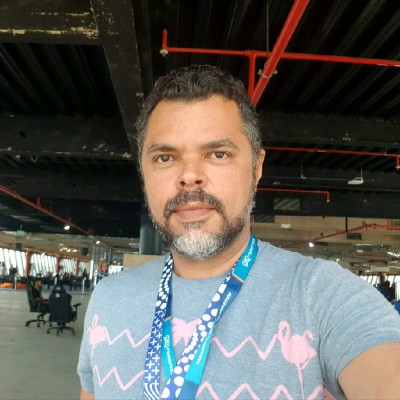
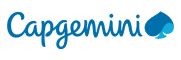