Building a News Aggregator App with Flutter and RSS Feeds
In the fast-paced digital age, staying informed about the latest news and updates is more crucial than ever. What if you could create your own news aggregator app to curate and deliver news content from various sources directly to your users’ fingertips? In this comprehensive guide, we’ll explore how to build a News Aggregator App using Flutter and RSS Feeds. With Flutter’s flexibility and RSS Feeds’ vast source of information, you can create a powerful, personalized news app that keeps your users engaged and informed.
Table of Contents
1. Introduction to News Aggregator Apps and Technology Stack
1.1. Understanding News Aggregator Apps
News aggregator apps have become increasingly popular as they provide users with a single platform to access news and updates from multiple sources. These apps collect news articles, blog posts, and other content from various publishers and present it in an organized, user-friendly manner. Users can customize their content preferences, making it a convenient way to stay informed.
1.2. Advantages of Building a News Aggregator App
Why build a News Aggregator App? Here are some key advantages:
- Personalization: Users can tailor their news feed to their interests.
- Centralized Information: All news sources in one place.
- Efficient: Saves time compared to visiting multiple websites.
- Monetization: Opportunities for ad revenue and subscriptions.
- Learning Experience: Gain valuable skills in app development.
1.3. Technology Stack: Flutter and RSS Feeds
To build a robust and cross-platform News Aggregator App, we’ll use Flutter, a popular UI toolkit from Google. Flutter allows you to create beautiful, natively compiled applications for mobile, web, and desktop from a single codebase. It’s known for its fast development process and expressive UI.
Additionally, we’ll leverage RSS (Really Simple Syndication) feeds to aggregate news content. RSS feeds are XML files that contain structured information about articles, making them an ideal source for news aggregation.
Now that we have a clear understanding of our project’s goals and technology stack, let’s move on to setting up the development environment.
2. Setting Up the Development Environment
2.1. Installing Flutter and Dart
Before we start coding, you need to set up your development environment. Follow these steps:
- Visit the Flutter website and download the latest stable release for your operating system (Windows, macOS, or Linux).
- Extract the downloaded archive and add the Flutter binary to your system’s PATH.
- Open a terminal and run flutter doctor to check for any missing dependencies. Install any missing packages as prompted.
2.2. Setting Up an IDE
Choose your preferred Integrated Development Environment (IDE) for Flutter development. Two popular options are:
- Android Studio: Provides a full-featured Flutter plugin.
- Visual Studio Code (VS Code): Offers a lightweight, customizable Flutter experience with extensions.
Install your chosen IDE and configure it for Flutter development by installing relevant extensions and plugins.
2.3. Creating a New Flutter Project
With your development environment set up, create a new Flutter project:
- Open your terminal or IDE.
- Run the following command to create a new Flutter project:
bash flutter create news_aggregator_app
Navigate to your project directory:
bash cd news_aggregator_app
Now that we have our Flutter project ready, let’s move on to designing the user interface.
3. Designing the User Interface
3.1. Building the App’s Wireframe
Before diving into coding the user interface, it’s essential to plan the app’s layout and design. Sketch out the wireframe or create a digital prototype to visualize how your app will look and function.
Consider the following elements:
- News Feed: The main screen displaying news articles.
- Navigation Bar: Providing access to different sections or categories.
- Article Card: The template for displaying individual news articles.
3.2. Implementing the UI with Flutter Widgets
Flutter provides a wide range of widgets to design your app’s user interface. Here are some commonly used widgets for building a News Aggregator App:
- AppBar: Create the top app bar with navigation.
- ListView: Display a scrollable list of news articles.
- Card: Design the news article cards.
- Drawer: Implement a navigation drawer for user settings (if desired).
- BottomNavigationBar: Add navigation tabs (e.g., Home, Categories, Settings).
Let’s take a look at an example code snippet to build a simple app interface:
dart import 'package:flutter/material.dart'; void main() { runApp(NewsAggregatorApp()); } class NewsAggregatorApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('News Aggregator'), ), body: ListView.builder( itemCount: 10, // Replace with the actual number of articles. itemBuilder: (context, index) { return Card( child: ListTile( title: Text('News Article Title'), subtitle: Text('Source: News Outlet'), onTap: () { // Handle article tap. }, ), ); }, ), ), ); } }
This code creates a basic app with an app bar and a list of news articles. You can customize it further according to your wireframe and design preferences.
3.3. Adding Navigation and Layout
To enhance user experience, add navigation and layout elements to your app. You can implement:
- A navigation drawer for easy access to categories or settings.
- Bottom navigation tabs to switch between sections.
- Customized layouts for article cards, allowing images, summaries, and more.
With the UI in place, we’re ready to fetch and parse RSS feeds for news content.
4. Fetching and Parsing RSS Feeds
4.1. Understanding RSS Feeds
RSS (Really Simple Syndication) is a standardized format for distributing web content, including news articles, blogs, and podcasts. It allows publishers to share structured data that can be easily consumed by applications like ours.
RSS feeds typically contain metadata such as the article title, publication date, author, and a link to the full article. This structured format makes it ideal for aggregating news content.
4.2. Using Dart Packages for RSS Parsing
To fetch and parse RSS feeds in your Flutter app, you can use Dart packages like http for making HTTP requests and webfeed for parsing RSS data. Here’s how to add these packages to your pubspec.yaml file:
yaml dependencies: flutter: sdk: flutter http: ^0.13.3 webfeed: ^2.0.0
Run ‘flutter pub get’ to fetch the packages.
4.3. Fetching Data from RSS Feeds
Now that you have the necessary packages, you can fetch and parse RSS feeds. Here’s a simplified example:
dart import 'package:http/http.dart' as http; import 'package:webfeed/webfeed.dart'; Future<List<RssItem>> fetchNews() async { final response = await http.get('https://example.com/news-feed.xml'); if (response.statusCode == 200) { final feed = RssFeed.parse(response.body); return feed.items; } else { throw Exception('Failed to load news'); } }
This code snippet fetches news from an RSS feed and parses it into a list of RssItem objects. Replace the URL with the actual RSS feed you want to use.
With the ability to fetch news data, let’s move on to displaying news articles in your app.
5. Displaying News Articles
5.1. Creating News Article Models
Before rendering news articles, create a model class to represent a news article. This class should include fields for the article’s title, description, publication date, source, and link. Here’s a sample model class:
dart class NewsArticle { final String title; final String description; final DateTime pubDate; final String source; final String link; NewsArticle({ required this.title, required this.description, required this.pubDate, required this.source, required this.link, }); }
5.2. Rendering News Articles
Now that you have a model class, you can display news articles using Flutter widgets. Here’s an exampl
5.3. Rendering News Articles
Now that you have a model class, you can display news articles using Flutter widgets. Here’s an example of how to create a list of news articles:
dart ListView.builder( itemCount: articles.length, // Replace with your list of articles. itemBuilder: (context, index) { final article = articles[index]; return Card( child: ListTile( title: Text(article.title), subtitle: Text('${article.source} - ${article.pubDate}'), onTap: () { // Handle article tap (open the full article). }, ), ); }, )
This code creates a list view of news articles, and when a user taps an article, you can implement logic to open the full article in a web view or within your app.
5.4. Designing a Custom News Card
To make your news aggregator app visually appealing, consider designing custom news cards with images, summaries, and other relevant information. Customize the Card widget to include these elements and style them according to your app’s design guidelines.
With news articles displayed, you can optionally implement user authentication to personalize news content.
6. User Authentication (Optional)
6.1. Implementing User Registration and Login
To provide users with personalized news feeds and the ability to save articles, you can implement user authentication. Popular authentication solutions for Flutter include Firebase Authentication and OAuth2.
Here’s a high-level overview of the process:
- Integrate an authentication package into your app (e.g., Firebase Authentication).
- Implement registration and login screens.
- Secure user data and preferences.
6.2. Personalizing News Content
Once users are authenticated, you can customize their news feed based on their preferences, saved articles, and behavior within the app. Consider implementing features like:
- Saving favorite articles.
- Recommending articles based on user interests.
- Allowing users to follow specific news sources or categories.
Now that you have a personalized news aggregator app, let’s explore how to manage and cache news for offline access.
7. Managing and Caching News
7.1. Implementing Offline Access with Local Storage
To ensure users can access news even when offline, implement local storage to cache news articles. You can use packages like shared_preferences or SQLite for this purpose.
Here’s an example of how to cache and retrieve news articles:
dart import 'package:shared_preferences/shared_preferences.dart'; Future<void> cacheNews(List<NewsArticle> articles) async { final prefs = await SharedPreferences.getInstance(); final articleList = articles.map((article) => article.toJson()).toList(); await prefs.setStringList('cached_articles', articleList); } Future<List<NewsArticle>> getCachedNews() async { final prefs = await SharedPreferences.getInstance(); final cachedArticles = prefs.getStringList('cached_articles') ?? []; return cachedArticles .map((jsonString) => NewsArticle.fromJson(jsonString)) .toList(); }
This code demonstrates how to cache and retrieve news articles using shared_preferences. Make sure to add appropriate error handling and data synchronization logic.
Additionally, consider implementing a mechanism to refresh news data periodically.
8. Push Notifications (Optional)
8.1. Integrating Push Notification Services
To keep users engaged and informed, you can integrate push notification services like Firebase Cloud Messaging (FCM) or OneSignal. Push notifications allow you to send real-time updates and personalized news alerts to your users.
Here’s a high-level overview of integrating push notifications:
- Set up a push notification service (e.g., FCM).
- Configure your Flutter app to receive notifications.
- Implement logic to send notifications for breaking news or user-specific updates.
By integrating push notifications, you can increase user engagement and retention.
9. Testing and Debugging
9.1. Emulator and Device Testing
Before releasing your News Aggregator App, thoroughly test it on emulators and physical devices. Test for various screen sizes, orientations, and Android/iOS versions to ensure compatibility.
Use Flutter’s debugging tools to identify and fix any issues. Common debugging techniques include setting breakpoints, inspecting the widget tree, and using Flutter DevTools.
10. Deployment and Distribution
10.1. Preparing the App for Release
Before deploying your app to app stores, make sure to:
- Optimize app performance and user experience.
- Remove any debugging code and dependencies.
- Create app icons and branding assets.
- Set up an appropriate privacy policy.
- Test the app on actual devices for final verification.
10.2. Publishing to App Stores
To publish your News Aggregator App on app stores (Google Play Store and Apple App Store), follow these steps:
- Register as a developer on the respective app stores.
- Generate signing keys for Android and iOS apps.
- Configure your app for release mode.
- Build the release version of your app.
- Submit your app to the app stores, following their guidelines.
Congratulations! Your News Aggregator App is now available for users to download and enjoy.
Conclusion
Building a News Aggregator App with Flutter and RSS Feeds is a rewarding endeavor that allows you to create a personalized news experience for your users. In this guide, we’ve covered the entire development process, from setting up the development environment to deploying your app to app stores.
Remember to continually update and improve your app to provide the best news aggregation experience possible. Stay up to date with Flutter and RSS feed technologies to adapt to evolving user needs and preferences.
Now it’s your turn to start building your News Aggregator App and make a significant impact in the world of news delivery. Happy coding!
Table of Contents
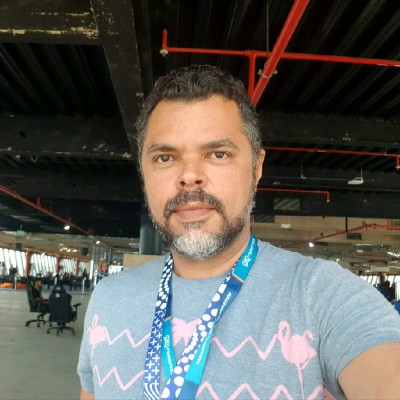
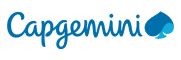