Building a Quiz App with Flutter: User Engagement and Scoring
In today’s fast-paced digital world, mobile applications have become an integral part of our lives. Among the multitude of app genres available, quiz apps are among the most popular due to their ability to entertain and educate simultaneously. In this tutorial, we will walk you through the process of building a captivating quiz app using Flutter. We will not only focus on the technical aspects but also delve into user engagement and scoring mechanisms to make your app stand out.
Table of Contents
1. Why Build a Quiz App with Flutter?
Before diving into the nitty-gritty of building our quiz app, let’s briefly discuss why Flutter is an excellent choice for this project.
1.1. Flutter: The Ideal Choice for Building Engaging Quiz Apps
Flutter, a framework developed by Google, has gained immense popularity in recent years for cross-platform app development. Here’s why it’s the perfect choice for building a quiz app:
- Cross-Platform Compatibility: Flutter allows you to write a single codebase that can be deployed on both iOS and Android platforms, saving time and effort.
- Rich UI Experience: Flutter provides a wide range of widgets and customization options, enabling you to create a visually appealing and engaging user interface.
- Fast Development: Hot reload feature in Flutter speeds up the development process, making it easier to experiment and iterate.
- Community and Plugins: Flutter has a thriving community and a vast collection of plugins, making it easy to implement various features and functionalities.
Now that we’ve established why Flutter is an excellent choice, let’s move on to the steps for building a user-engaging quiz app.
Step 1: Project Setup
The first step in building any Flutter app is setting up your development environment. Ensure you have Flutter installed, and you can check its version by running the following command in your terminal:
bash flutter --version
If you don’t have Flutter installed, refer to the official Flutter installation guide for your operating system.
Once Flutter is installed, create a new Flutter project by running:
bash flutter create quiz_app
This command will create a new directory named quiz_app with the basic project structure.
Step 2: Designing the User Interface
Building the Quiz Screen
Now, let’s design the quiz screen, which is the heart of our quiz app. We’ll use the flutter/material.dart package to create a visually appealing user interface.
dart import 'package:flutter/material.dart'; class QuizScreen extends StatefulWidget { @override _QuizScreenState createState() => _QuizScreenState(); } class _QuizScreenState extends State<QuizScreen> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Quiz App'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( 'Question 1', style: TextStyle(fontSize: 24), ), SizedBox(height: 20), ElevatedButton( onPressed: () { // Handle user's answer }, child: Text('Option 1'), ), ElevatedButton( onPressed: () { // Handle user's answer }, child: Text('Option 2'), ), ElevatedButton( onPressed: () { // Handle user's answer }, child: Text('Option 3'), ), ElevatedButton( onPressed: () { // Handle user's answer }, child: Text('Option 4'), ), ], ), ), ); } }
In this code sample, we’ve created a QuizScreen widget with a simple layout. The quiz question is displayed at the top, followed by four answer options as buttons. We will later replace the static question and options with dynamic data.
Adding Animation and Transitions
To make your quiz app more engaging, consider adding animations and transitions between questions. Flutter provides the AnimatedContainer and Hero widgets for this purpose.
Here’s an example of using the AnimatedContainer widget to animate the button’s background color when the user selects an answer:
dart AnimatedContainer( duration: Duration(milliseconds: 300), decoration: BoxDecoration( color: selectedAnswer == optionText ? Colors.green : Colors.blue, borderRadius: BorderRadius.circular(10), ), child: ElevatedButton( onPressed: () { // Handle user's answer setState(() { selectedAnswer = optionText; }); }, child: Text(optionText), ), )
In this code, we’re using the selectedAnswer variable to track the user’s choice and update the button’s background color accordingly.
Implementing Navigation
To navigate between questions, use the Navigator widget to push a new question screen onto the stack when the user answers a question. Here’s an example:
dart Navigator.of(context).push( MaterialPageRoute( builder: (context) => QuizScreen( questionIndex: questionIndex + 1, ), ), );
By pushing a new QuizScreen onto the stack with an updated questionIndex, you can display the next question when the user moves forward.
Step 3: Managing Questions and Answers
To create a dynamic quiz app, you need a way to manage questions and answers. You can store your quiz data in a structured format, such as JSON, and then load it into your app.
Creating a Quiz Data Model
Start by creating a Question class to represent a quiz question:
dart class Question { final String questionText; final List<String> options; final int correctOptionIndex; Question({ required this.questionText, required this.options, required this.correctOptionIndex, }); }
In this model, questionText represents the text of the question, options is a list of possible answers, and correctOptionIndex points to the correct answer in the options list.
Loading Quiz Data
You can load your quiz data from a JSON file or an API. For simplicity, let’s create a list of questions directly in your Dart code:
dart List<Question> quizQuestions = [ Question( questionText: 'What is the capital of France?', options: ['Berlin', 'London', 'Madrid', 'Paris'], correctOptionIndex: 3, ), Question( questionText: 'Which planet is known as the Red Planet?', options: ['Mars', 'Venus', 'Earth', 'Jupiter'], correctOptionIndex: 0, ), // Add more questions here ];
Displaying Questions Dynamically
Now, you can modify your QuizScreen widget to display questions and options dynamically:
dart class QuizScreen extends StatefulWidget { final List<Question> questions; final int questionIndex; QuizScreen({ required this.questions, required this.questionIndex, }); @override _QuizScreenState createState() => _QuizScreenState(); } class _QuizScreenState extends State<QuizScreen> { @override Widget build(BuildContext context) { Question currentQuestion = widget.questions[widget.questionIndex]; return Scaffold( appBar: AppBar( title: Text('Quiz App'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( currentQuestion.questionText, style: TextStyle(fontSize: 24), ), SizedBox(height: 20), ...currentQuestion.options.map((optionText) { return AnimatedContainer( duration: Duration(milliseconds: 300), decoration: BoxDecoration( color: selectedAnswer == optionText ? Colors.green : Colors.blue, borderRadius: BorderRadius.circular(10), ), child: ElevatedButton( onPressed: () { // Handle user's answer setState(() { selectedAnswer = optionText; }); }, child: Text(optionText), ), ); }).toList(), ], ), ), ); } }
With this modification, your QuizScreen widget can display different questions based on the questionIndex.
Step 4: Scoring Mechanism
To keep users engaged and motivated, implement a scoring mechanism in your quiz app. Users should be able to track their progress and see how well they’re doing.
Tracking User Scores
Start by creating a score variable to keep track of the user’s score:
dart int score = 0;
Scoring Questions
When a user selects an answer, check if it’s the correct one and update the score accordingly:
dart ElevatedButton( onPressed: () { // Handle user's answer setState(() { if (selectedAnswer == currentQuestion.options[currentQuestion.correctOptionIndex]) { score++; } }); // Move to the next question moveToNextQuestion(); }, child: Text(optionText), )
Here, moveToNextQuestion is a function you can create to navigate to the next question.
Displaying the Score
To display the user’s score, you can add a score widget to your app’s UI:
dart Text( 'Score: $score/${widget.questions.length}', style: TextStyle(fontSize: 20), )
This text widget shows the user’s current score out of the total number of questions in the quiz.
Step 5: User Engagement Strategies
Building a quiz app is not just about coding; it’s also about engaging your users effectively. Here are some user engagement strategies you can implement:
1. Timer
Add a timer to each question to create a sense of urgency. Users will be more engaged as they race against the clock to answer questions.
dart int _timer = 15; // Initial timer value in seconds Timer.periodic(Duration(seconds: 1), (timer) { setState(() { if (_timer > 0) { _timer--; } else { // Time's up, move to the next question moveToNextQuestion(); _timer = 15; // Reset the timer for the next question } }); });
2. Lifelines
Incorporate lifelines such as “50-50” or “Ask a Friend” to make the game more interactive. Users can use these lifelines to eliminate wrong answers or get help from others.
3. Leaderboards
Implement a global or local leaderboard to encourage healthy competition among users. Displaying high scores can motivate players to improve their performance.
4. Rewards and Achievements
Reward users with virtual currency or badges for completing quizzes or achieving high scores. These rewards can keep users engaged and coming back for more.
Step 6: Testing and Debugging
Before releasing your quiz app to the public, thorough testing and debugging are essential. Ensure that questions are displayed correctly, the scoring system works as expected, and there are no crashes or unexpected behavior.
Use Flutter’s debugging tools, such as print statements and the DevTools package, to identify and fix any issues.
Step 7: Deployment
Once your quiz app is polished and bug-free, it’s time to deploy it to app stores. Follow the official Flutter documentation to package and publish your app for both Android and iOS platforms.
Conclusion
In this tutorial, we’ve covered the steps to build a captivating quiz app with Flutter. We explored designing the user interface, managing questions and answers, implementing a scoring mechanism, and discussed user engagement strategies. With Flutter’s flexibility and a focus on user engagement, you’re well-equipped to create an entertaining and educational quiz app that keeps users hooked. Start building your quiz app today and watch your users engage and compete for the top spot on the leaderboard!
Table of Contents
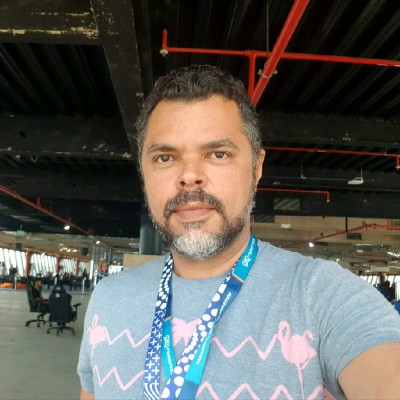
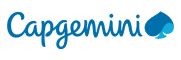