Creating Custom Animations in Flutter: A Step-by-Step Guide
Animations are a vital part of creating engaging and visually appealing mobile apps. In Flutter, Google’s open-source UI software development toolkit, you have a powerful framework at your disposal for creating custom animations that can breathe life into your user interface. Whether you want to add subtle transitions or create complex, eye-catching animations, Flutter has got you covered.
Table of Contents
This step-by-step guide will walk you through the process of creating custom animations in Flutter. We’ll cover essential concepts, Flutter’s animation framework, and provide code samples to help you get started.
1. Understanding Animations in Flutter
1.1. Why Use Custom Animations?
Animations are more than just eye candy. They can enhance user experience, provide feedback, and make your app feel responsive. Custom animations are particularly useful when standard widgets and transitions don’t meet your design requirements. By creating custom animations, you have full control over how your UI elements move, fade, or transform, enabling you to create unique user experiences.
1.2. Key Animation Concepts
Before we dive into the code, it’s essential to understand some fundamental animation concepts in Flutter:
- Animation: An animation in Flutter represents a changing value over time. It can be as simple as changing opacity or as complex as a full-page transition.
- Tween: A Tween defines a range of values that an animation should interpolate between. For example, you can use a Tween to define the starting and ending values for an animation.
- Controller: An AnimationController is responsible for managing the animation’s state. It controls the animation’s playback, duration, and whether it runs forwards or in reverse.
2. Flutter’s Animation Framework
Flutter provides a robust animation framework that simplifies the process of creating animations. Here are some essential components of Flutter’s animation framework:
2.1. Animation Controllers
An AnimationController controls the timing and playback of an animation. It allows you to specify the duration, curve (easing function), and whether the animation should loop or reverse. Here’s an example of creating an AnimationController:
dart AnimationController controller = AnimationController( duration: Duration(seconds: 2), vsync: this, // TickerProvider (e.g., State or TickerProviderStateMixin) );
2.2. Tween Animation
A Tween Animation defines the range of values that an animation should interpolate between. For instance, to animate a widget’s position from one point to another, you can use a Tween<Offset>:
dart Animation<Offset> positionAnimation = Tween<Offset>( begin: Offset(0, 0), end: Offset(100, 100), ).animate(controller);
2.3. AnimatedBuilder
The AnimatedBuilder widget is a handy tool for building widgets that depend on an animation. It allows you to rebuild a part of your UI tree whenever the animation value changes. Here’s a basic example of how to use AnimatedBuilder:
dart AnimatedBuilder( animation: positionAnimation, builder: (context, child) { return Positioned( left: positionAnimation.value.dx, top: positionAnimation.value.dy, child: child, ); }, child: MyWidget(), )
3. Creating Custom Animations
Now that we’ve covered the basics of Flutter’s animation framework, let’s create some custom animations.
3.1. Simple Opacity Animation
Let’s start with a straightforward example: fading a widget in and out using an opacity animation. In this case, we’ll use a Tween<double> to interpolate the opacity value.
dart class OpacityAnimationExample extends StatefulWidget { @override _OpacityAnimationExampleState createState() => _OpacityAnimationExampleState(); } class _OpacityAnimationExampleState extends State<OpacityAnimationExample> with SingleTickerProviderStateMixin { late AnimationController controller; late Animation<double> opacityAnimation; @override void initState() { super.initState(); controller = AnimationController( duration: Duration(seconds: 2), vsync: this, ); opacityAnimation = Tween<double>( begin: 0.0, end: 1.0, ).animate(controller); controller.forward(); // Start the animation } @override void dispose() { controller.dispose(); // Clean up the controller super.dispose(); } @override Widget build(BuildContext context) { return Center( child: AnimatedBuilder( animation: opacityAnimation, builder: (context, child) { return Opacity( opacity: opacityAnimation.value, child: Container( width: 200, height: 200, color: Colors.blue, ), ); }, ), ); } }
In this example, we create an opacity animation that starts from 0.0 and ends at 1.0, making a blue container fade in. The ‘AnimatedBuilder’ widget rebuilds the container whenever the animation value changes.
3.2. Animating Widget Properties
Flutter allows you to animate various widget properties, such as position, size, and rotation. Let’s explore how to animate a widget’s position using the Transform widget:
dart class PositionAnimationExample extends StatefulWidget { @override _PositionAnimationExampleState createState() => _PositionAnimationExampleState(); } class _PositionAnimationExampleState extends State<PositionAnimationExample> with SingleTickerProviderStateMixin { late AnimationController controller; late Animation<Offset> positionAnimation; @override void initState() { super.initState(); controller = AnimationController( duration: Duration(seconds: 2), vsync: this, ); positionAnimation = Tween<Offset>( begin: Offset(0, 0), end: Offset(100, 100), ).animate(controller); controller.forward(); // Start the animation } @override void dispose() { controller.dispose(); // Clean up the controller super.dispose(); } @override Widget build(BuildContext context) { return Center( child: AnimatedBuilder( animation: positionAnimation, builder: (context, child) { return Transform.translate( offset: positionAnimation.value, child: Container( width: 100, height: 100, color: Colors.red, ), ); }, ), ); } }
In this example, we use the ‘Transform.translate’ widget to move the red container from its initial position to a new position defined by the ‘positionAnimation’.
3.3. Complex Custom Animations
Custom animations can get as complex as your imagination. You can combine multiple animations, use different curves, and create intricate animations to match your app’s design. Here’s a simplified example of a complex custom animation:
dart class ComplexAnimationExample extends StatefulWidget { @override _ComplexAnimationExampleState createState() => _ComplexAnimationExampleState(); } class _ComplexAnimationExampleState extends State<ComplexAnimationExample> with SingleTickerProviderStateMixin { late AnimationController controller; late Animation<Offset> positionAnimation; late Animation<double> opacityAnimation; @override void initState() { super.initState(); controller = AnimationController( duration: Duration(seconds: 2), vsync: this, ); positionAnimation = Tween<Offset>( begin: Offset(0, 0), end: Offset(100, 100), ).animate(CurvedAnimation( parent: controller, curve: Curves.easeInOut, )); opacityAnimation = Tween<double>( begin: 0.0, end: 1.0, ).animate(controller); controller.forward(); // Start the animation } @override void dispose() { controller.dispose(); // Clean up the controller super.dispose(); } @override Widget build(BuildContext context) { return Center( child: AnimatedBuilder( animation: controller, builder: (context, child) { return Transform.translate( offset: positionAnimation.value, child: Opacity( opacity: opacityAnimation.value, child: Container( width: 150, height: 150, color: Colors.green, ), ), ); }, ), ); } }
In this example, we combine both position and opacity animations to create a more intricate animation effect.
4. Interactivity and Gestures
Creating animations that respond to user interactions is a crucial aspect of mobile app development. Flutter makes it relatively straightforward to add interactivity to your custom animations.
4.1. Triggering Animations
You can trigger animations in response to user actions, such as button presses or gestures. For example, to animate a widget when a button is pressed, you can use the following code:
dart GestureDetector( onTap: () { controller.forward(); // Start the animation }, child: MyButtonWidget(), )
4.2. Gesture-Based Animations
Flutter provides various gesture detectors like GestureDetector, Draggable, and GestureDetector, which you can use to create interactive animations. For instance, you can create a draggable widget that animates when dragged:
dart class DraggableAnimationExample extends StatefulWidget { @override _DraggableAnimationExampleState createState() => _DraggableAnimationExampleState(); } class _DraggableAnimationExampleState extends State<DraggableAnimationExample> { late AnimationController controller; late Animation<Offset> positionAnimation; @override void initState() { super.initState(); controller = AnimationController( duration: Duration(seconds: 1), vsync: this, ); positionAnimation = Tween<Offset>( begin: Offset(0, 0), end: Offset(0, 100), ).animate(controller); } @override void dispose() { controller.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return Center( child: GestureDetector( onVerticalDragUpdate: (details) { // Update the animation value based on drag controller.value -= details.primaryDelta! / 100.0; }, onVerticalDragEnd: (details) { // Determine whether to complete or reverse the animation if (details.velocity.pixelsPerSecond.dy > 200) { controller.reverse(); } else { controller.forward(); } }, child: AnimatedBuilder( animation: controller, builder: (context, child) { return Transform.translate( offset: positionAnimation.value, child: Container( width: 100, height: 100, color: Colors.orange, ), ); }, ), ), ); } }
In this example, the widget can be vertically dragged up or down, and the animation responds to the drag gestures. The onVerticalDragUpdate and onVerticalDragEnd callbacks control the animation based on the user’s actions.
5. Optimizing Animations
Optimizing animations is crucial to ensure smooth performance in your Flutter app. Here are some tips to enhance animation performance:
5.1. Performance Considerations
- Minimize the Rebuild Area: Use the AnimatedBuilder widget or similar techniques to rebuild only the parts of the UI that depend on the animation. This reduces the number of widgets that need to be rebuilt and improves performance.
- Use const Constructors: Whenever possible, use const constructors for your widgets to reduce widget tree changes during animations.
- Avoid Excessive Widgets: Limit the number of widgets in your UI tree, especially when animating complex layouts. Deep widget trees can slow down animations.
5.2. Using AnimatedContainer and AnimatedOpacity
Flutter provides specialized widgets like AnimatedContainer and AnimatedOpacity that simplify common animations by handling the underlying animation logic for you. These widgets automatically animate changes to their properties, such as size and opacity.
dart AnimatedContainer( duration: Duration(seconds: 1), width: _isExpanded ? 200.0 : 50.0, height: _isExpanded ? 200.0 : 50.0, color: _isExpanded ? Colors.blue : Colors.red, )
In this example, the container’s size and color will animate smoothly when _isExpanded changes.
5.3. Hero Animations
For seamless transitions between screens, consider using Hero animations. Hero animations smoothly interpolate the transition of a widget from one screen to another. This can create visually pleasing effects when navigating between different parts of your app.
Conclusion
Creating custom animations in Flutter allows you to add a touch of creativity and interactivity to your mobile app. With Flutter’s animation framework and the flexibility it offers, you can bring your app’s user interface to life in ways that captivate and engage your users.
Remember to start with the basics, understanding key animation concepts and Flutter’s animation framework. Experiment with simple animations and gradually progress to more complex ones as you become more comfortable with the framework.
Flutter’s focus on performance and developer productivity makes it an excellent choice for building animations that are not only visually appealing but also responsive and smooth. So, dive into the world of custom animations in Flutter, and let your imagination run wild as you create delightful user experiences. Happy animating!
Table of Contents
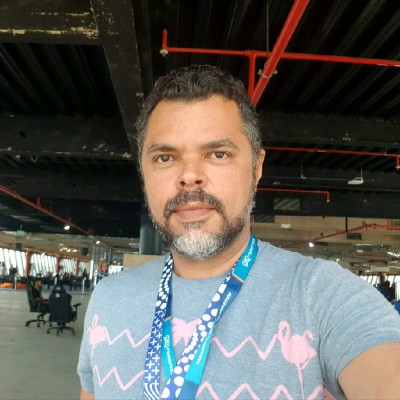
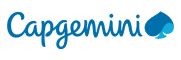