Building a Cryptocurrency Tracker App with Flutter: API Integration
Cryptocurrency tracking apps are essential tools for investors and enthusiasts who want to keep an eye on market trends. With Flutter, a powerful UI toolkit for building natively compiled applications for mobile, web, and desktop, you can create a responsive and visually appealing cryptocurrency tracker app. This blog post will guide you through the process of building a Flutter app that integrates with cryptocurrency APIs to fetch and display real-time data.
Understanding the Basics of Cryptocurrency APIs
Cryptocurrency APIs provide real-time data on market prices, trading volumes, and other relevant metrics. These APIs are vital for building apps that require up-to-date information about various cryptocurrencies. Understanding how to integrate these APIs with Flutter is crucial for developing a functional tracker app.
Setting Up Your Flutter Environment
Before diving into API integration, ensure that your Flutter environment is set up correctly. You’ll need to have Flutter installed on your system and be familiar with basic Flutter concepts such as widgets, state management, and navigation.
```bash $ flutter create crypto_tracker $ cd crypto_tracker $ flutter run ```
Integrating a Cryptocurrency API
To start, choose a cryptocurrency API provider that offers the data you need. For this example, we’ll use the CoinGecko API, which provides free access to a wide range of cryptocurrency data.
1. Adding HTTP Package
First, add the `http` package to your Flutter project to enable API calls.
```yaml dependencies: flutter: sdk: flutter http: ^0.13.3 ```
Run `flutter pub get` to install the package.
2. Fetching Cryptocurrency Data
Next, create a service class to handle API requests. This class will make a call to the CoinGecko API and return a list of cryptocurrencies with their current prices.
```dart import 'package:http/http.dart' as http; import 'dart:convert'; class CryptoService { final String apiUrl = "https://api.coingecko.com/api/v3/coins/markets"; Future<List<dynamic>> fetchCryptocurrencies() async { final response = await http.get(Uri.parse('$apiUrl?vs_currency=usd&order=market_cap_desc&per_page=10&page=1&sparkline=false')); if (response.statusCode == 200) { return json.decode(response.body); } else { throw Exception('Failed to load data'); } } } ```
Displaying Data in the Flutter UI
Once you have fetched the data, the next step is to display it in your app’s UI.
1. Creating a Cryptocurrency List Widget
Create a widget that displays a list of cryptocurrencies. This widget will use a `FutureBuilder` to fetch and display data asynchronously.
```dart import 'package:flutter/material.dart'; import 'crypto_service.dart'; class CryptoList extends StatelessWidget { final CryptoService cryptoService = CryptoService(); @override Widget build(BuildContext context) { return FutureBuilder<List<dynamic>>( future: cryptoService.fetchCryptocurrencies(), builder: (context, snapshot) { if (snapshot.connectionState == ConnectionState.waiting) { return CircularProgressIndicator(); } else if (snapshot.hasError) { return Text('Error: ${snapshot.error}'); } else { return ListView.builder( itemCount: snapshot.data?.length, itemBuilder: (context, index) { var crypto = snapshot.data?[index]; return ListTile( title: Text(crypto['name']), subtitle: Text('Price: $${crypto['current_price']}'), leading: Image.network(crypto['image']), ); }, ); } }, ); } } ```
2. Integrating the Widget into Your App
Modify your `main.dart` file to include the `CryptoList` widget in your app’s main screen.
```dart import 'package:flutter/material.dart'; import 'crypto_list.dart'; void main() => runApp(CryptoTrackerApp()); class CryptoTrackerApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Crypto Tracker', home: Scaffold( appBar: AppBar( title: Text('Crypto Tracker'), ), body: CryptoList(), ), ); } } ```
Adding Refresh Capability
A real-time tracker should allow users to refresh data. Implement a pull-to-refresh feature using the `RefreshIndicator` widget.
```dart class CryptoList extends StatefulWidget { @override _CryptoListState createState() => _CryptoListState(); } class _CryptoListState extends State<CryptoList> { final CryptoService cryptoService = CryptoService(); Future<void> _refreshCryptos() async { setState(() {}); } @override Widget build(BuildContext context) { return RefreshIndicator( onRefresh: _refreshCryptos, child: FutureBuilder<List<dynamic>>( future: cryptoService.fetchCryptocurrencies(), builder: (context, snapshot) { if (snapshot.connectionState == ConnectionState.waiting) { return CircularProgressIndicator(); } else if (snapshot.hasError) { return Text('Error: ${snapshot.error}'); } else { return ListView.builder( itemCount: snapshot.data?.length, itemBuilder: (context, index) { var crypto = snapshot.data?[index]; return ListTile( title: Text(crypto['name']), subtitle: Text('Price: $${crypto['current_price']}'), leading: Image.network(crypto['image']), ); }, ); } }, ), ); } } ```
Enhancing the User Interface
To make your app more visually appealing, consider customizing the design by adding icons, colors, and other UI elements that enhance user experience. You can also implement features like sorting and filtering cryptocurrencies based on user preferences.
Conclusion
Building a cryptocurrency tracker app with Flutter is a great way to learn API integration and UI development in Flutter. By following the steps outlined in this article, you can create a functional and visually appealing app that provides real-time cryptocurrency data to users. With further customization and enhancements, you can expand the app’s functionality and provide more value to your users.
Further Reading:
Table of Contents
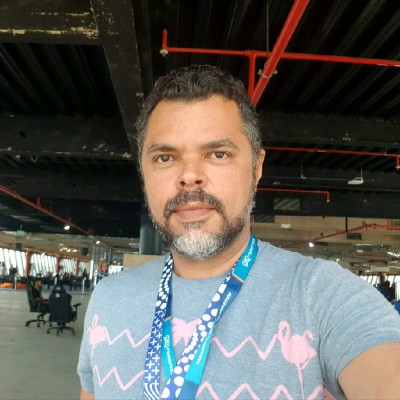
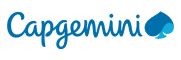