How do you implement a custom transition between screens in Flutter?
Implementing a custom transition between screens in Flutter involves leveraging the `PageRouteBuilder` class to define a custom page route with a specified transition animation. Here’s a step-by-step guide:
- Create a Custom Page Route:
Start by creating a custom page route using the `PageRouteBuilder` class. Define the `pageBuilder` to return the widget for the new screen and set the `transitionsBuilder` to handle the animation between screens.
```dart PageRouteBuilder( pageBuilder: (context, animation, secondaryAnimation) => MyCustomScreen(), transitionsBuilder: (context, animation, secondaryAnimation, child) { const begin = Offset(1.0, 0.0); const end = Offset.zero; const curve = Curves.easeInOut; var tween = Tween(begin: begin, end: end).chain(CurveTween(curve: curve)); var offsetAnimation = animation.drive(tween); return SlideTransition(position: offsetAnimation, child: child); }, ); ```
- Define Custom Screen:
Create the custom screen, in this case, `MyCustomScreen`, which will be displayed when the transition occurs.
```dart class MyCustomScreen extends StatelessWidget { // Your custom screen implementation } ```
- Specify Transition Parameters:
Customize the transition parameters, such as the offset, curve, and duration, based on your design preferences. These parameters determine the direction and style of the transition animation.
```dart const begin = Offset(1.0, 0.0); const end = Offset.zero; const curve = Curves.easeInOut; var tween = Tween(begin: begin, end: end).chain(CurveTween(curve: curve)); ```
- Apply Transition:
Apply the transition to the child widget using the `SlideTransition` widget. This widget animates the position of the child based on the specified transition animation.
```dart return SlideTransition(position: offsetAnimation, child: child); ```
- Integrate in Navigator:
Finally, use the custom page route when navigating to the new screen using the `Navigator`.
```dart Navigator.of(context).push( PageRouteBuilder( pageBuilder: (context, animation, secondaryAnimation) => MyCustomScreen(), transitionsBuilder: (context, animation, secondaryAnimation, child) { // ... (as shown in the first step) }, ), ); ```
By following these steps, you can implement a custom transition effect that adds a unique visual appeal to the navigation between screens in your Flutter application.
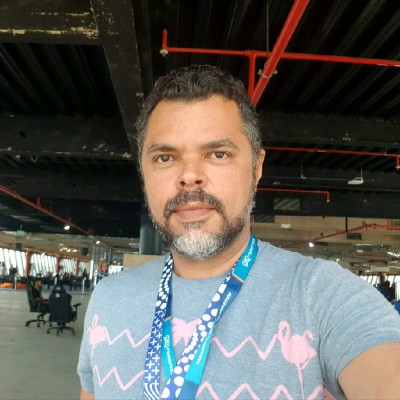
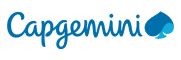