Can you customize the app’s theme in Flutter?
In Flutter, customizing the app’s theme is a crucial aspect of creating a visually appealing and consistent user interface. The theme in Flutter encompasses various design elements such as colors, fonts, and shapes, providing a unified look and feel across the entire application.
To customize the app’s theme in Flutter, you can utilize the `Theme` class. This class allows you to define a set of visual properties that can be applied globally. For instance, you can specify the primary and accent colors, typography, and other style-related attributes.
Example:
```dart import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( theme: ThemeData( primaryColor: Colors.blue, // Set the primary color accentColor: Colors.greenAccent, // Set the accent color fontFamily: 'Roboto', // Set the default font family // Add more theme customization options as needed ), home: MyHomePage(), ); } } class MyHomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Custom Theme Example'), ), body: Center( child: Text( 'Welcome to Flutter!', style: Theme.of(context).textTheme.headline1, ), ), ); } } ```
In this example, the custom theme is applied to the entire `MaterialApp`, influencing various UI elements, such as the `AppBar` and `Text` widget. The `Theme.of(context)` allows you to access the current theme properties within the widget tree.
Key points to remember when customizing the app’s theme in Flutter include defining the theme in the `MaterialApp`, specifying primary and accent colors, setting typography, and using the defined theme properties throughout the app for a consistent and aesthetically pleasing user experience.
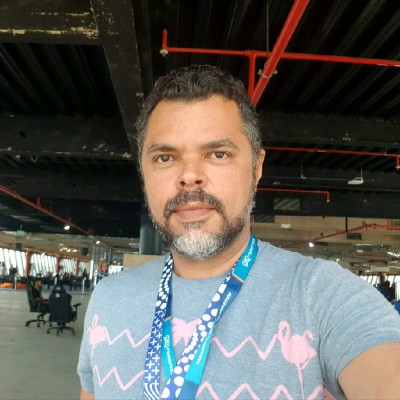
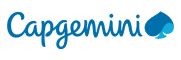