Using Flutter with GraphQL: Efficient Data Fetching in Apps
In today’s fast-paced digital world, mobile app users demand snappy and responsive experiences. Slow and inefficient data fetching can lead to user frustration and abandonment of your app. To keep your users engaged, it’s essential to optimize data fetching in your Flutter apps. One powerful tool for achieving this is GraphQL.
Table of Contents
In this blog post, we’ll explore how to use Flutter with GraphQL to efficiently fetch data in your apps. We’ll cover the basics of GraphQL, setting up a GraphQL server, integrating it with your Flutter app, and best practices for optimizing data fetching. By the end, you’ll be equipped to build highly responsive and performant mobile apps.
1. What is GraphQL?
1.1. GraphQL vs. REST
Before diving into how to use GraphQL with Flutter, let’s understand what GraphQL is and how it differs from REST.
GraphQL is a query language for your API, designed to provide a more efficient, powerful, and flexible alternative to REST APIs. In a REST API, you typically have multiple endpoints for different resources, and each endpoint may return more data than needed. This can lead to over-fetching or under-fetching of data, resulting in performance bottlenecks or wasted bandwidth.
In contrast, GraphQL allows clients to request precisely the data they need, nothing more and nothing less, in a single request. This eliminates over-fetching and under-fetching issues and results in more efficient data fetching.
1.2. GraphQL Queries and Mutations
In GraphQL, clients define their data requirements using queries and can also modify data on the server using mutations. Here’s a basic example of a GraphQL query:
graphql query { getUser(id: 1) { id name email } }
In this query, the client is asking for the user’s id, name, and email fields. The server responds with only the requested data.
Now, let’s look at a mutation:
graphql mutation { updateUser(id: 1, name: "John Doe") { id name } }
This mutation updates the user’s name and returns the updated id and name.
With GraphQL, you have fine-grained control over your data, making it an excellent choice for mobile app development.
2. Setting Up a GraphQL Server
To use GraphQL in your Flutter app, you’ll first need to set up a GraphQL server. There are various tools and libraries available for creating GraphQL servers, including Apollo Server, Express GraphQL, and more. Choose the one that best fits your backend technology stack.
Here’s a simplified example of setting up a GraphQL server using Apollo Server in Node.js:
javascript const { ApolloServer, gql } = require('apollo-server'); const typeDefs = gql` type User { id: ID! name: String! email: String! } type Query { getUser(id: ID!): User } type Mutation { updateUser(id: ID!, name: String!): User } `; const users = [ { id: '1', name: 'Alice', email: 'alice@example.com' }, { id: '2', name: 'Bob', email: 'bob@example.com' }, ]; const resolvers = { Query: { getUser: (_, { id }) => users.find(user => user.id === id), }, Mutation: { updateUser: (_, { id, name }) => { const user = users.find(user => user.id === id); if (!user) throw new Error('User not found'); user.name = name; return user; }, }, }; const server = new ApolloServer({ typeDefs, resolvers }); server.listen().then(({ url }) => { console.log(`Server running at ${url}`); });
In this example, we define a simple schema with a User type, a Query type for fetching user data, and a Mutation type for updating user data. The resolver functions handle the actual data retrieval and manipulation.
Once you have your GraphQL server up and running, it’s time to integrate it with your Flutter app.
3. Integrating GraphQL with Flutter
3.1. Installing Dependencies
To use GraphQL in your Flutter app, you’ll need some packages to handle GraphQL queries and mutations. The most popular package for this purpose is graphql_flutter. You can add it to your pubspec.yaml file like this:
yaml dependencies: graphql_flutter: ^5.0.0
After adding the dependency, don’t forget to run ‘flutter pub ge’t to fetch the package.
3.2. Writing GraphQL Queries
With the graphql_flutter package installed, you can start writing GraphQL queries in your Flutter app. Here’s how you can define a simple query:
dart import 'package:graphql_flutter/graphql_flutter.dart'; final String getUserQuery = ''' query GetUser($id: ID!) { getUser(id: $id) { id name email } } ''';
In this example, we define a query named GetUser that takes an id as a variable. This query fetches the id, name, and email fields of a user.
3.3. Making GraphQL Requests
To make GraphQL requests in Flutter, you’ll need to set up a GraphQLClient. You’ll also need a Cache for caching GraphQL responses, which we’ll discuss in more detail later.
Here’s how you can set up a GraphQLClient and make a query using the previously defined getUserQuery:
dart import 'package:graphql_flutter/graphql_flutter.dart'; final HttpLink httpLink = HttpLink( uri: 'http://localhost:4000/graphql', // Replace with your GraphQL server URI ); final AuthLink authLink = AuthLink( getToken: () async => 'Bearer $yourAuthToken', // Replace with your authentication logic ); final Link link = authLink.concat(httpLink); final ValueNotifier<GraphQLClient> client = ValueNotifier( GraphQLClient( cache: GraphQLCache(store: InMemoryStore()), // Initialize cache link: link, // Set the link ), ); final GraphQLClient graphQLClient = GraphQLClient( cache: GraphQLCache(store: InMemoryStore()), link: link, ); final QueryOptions options = QueryOptions( document: gql(getUserQuery), variables: <String, dynamic>{'id': '1'}, // Pass variables ); final QueryResult result = await graphQLClient.query(options); if (result.hasException) { print(result.exception.toString()); } else { print(result.data.toString()); }
In this code, we create an HttpLink to connect to our GraphQL server, an AuthLink for handling authentication (replace yourAuthToken with your actual authentication logic), and then combine these links into a single Link. We initialize a GraphQLClient with the cache and link, and finally, we execute a query using the query method.
This is a basic setup for making GraphQL requests in Flutter. However, to create a robust and efficient data fetching system, there are several optimization techniques you should consider.
4. Optimizing Data Fetching
Efficient data fetching is crucial for a smooth user experience in your Flutter app. Here are some optimization techniques to consider when using GraphQL:
4.1. Batch Requests
One of the advantages of GraphQL is the ability to batch multiple queries into a single request. This reduces the overhead of multiple HTTP requests, improving performance. To batch requests in Flutter, you can use the multi operation:
dart final QueryOptions options1 = QueryOptions(document: gql(query1), variables: variables1); final QueryOptions options2 = QueryOptions(document: gql(query2), variables: variables2); final List<QueryOptions> allOptions = [options1, options2]; final QueryResultBatch batchResult = await graphQLClient.queryMulti(allOptions); for (var result in batchResult) { if (result.hasException) { print(result.exception.toString()); } else { print(result.data.toString()); } }
4.2. Caching
Caching is essential for reducing redundant network requests and speeding up data retrieval. The graphql_flutter package provides an in-memory cache by default, but you can also use more advanced caching libraries like Hive or Apollo Client for Flutter.
To implement caching, you’ll need to define a cache key for each GraphQL operation and check the cache before making a network request.
4.3. Pagination
When dealing with large datasets, consider implementing pagination to fetch data in smaller, manageable chunks. This prevents your app from loading excessive data upfront and keeps it responsive.
GraphQL supports pagination through arguments like first, after, last, and before. You can use these arguments in your queries to request specific portions of data.
graphql query { getPosts(first: 10, after: "cursor") { edges { node { id title } } pageInfo { hasNextPage endCursor } } }
By incorporating pagination into your app, you ensure that it remains performant even when dealing with extensive datasets.
Conclusion
Efficient data fetching is a critical aspect of mobile app development, and GraphQL provides a powerful solution to this challenge. In this blog post, we’ve explored the fundamentals of GraphQL, setting up a GraphQL server, integrating it with a Flutter app, and optimizing data fetching.
By using GraphQL with Flutter and implementing techniques like batch requests, caching, and pagination, you can create mobile apps that deliver a responsive and seamless user experience. Stay up to date with the latest GraphQL and Flutter developments to continuously improve your app’s performance and user satisfaction.
Remember that while GraphQL offers numerous advantages, it’s essential to tailor its use to your specific app requirements and keep an eye on performance metrics to ensure your app remains fast and efficient. Happy coding!
Incorporating GraphQL into your Flutter app can greatly improve data fetching efficiency. Here’s a guide on how to set it up and optimize for a better user experience.
Table of Contents
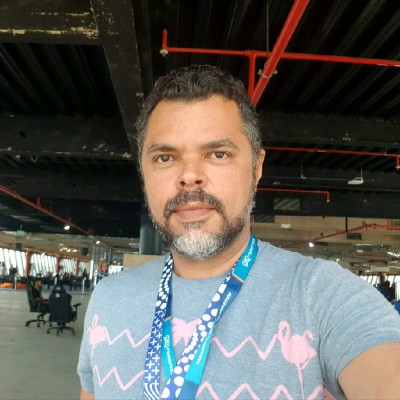
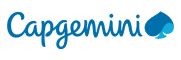