Flutter for E-commerce Apps: Essential Features and Functionality
In today’s digital era, E-commerce has become an integral part of our lives. With the growing popularity of online shopping, businesses need to adapt and provide users with seamless shopping experiences. Flutter, a versatile and powerful framework developed by Google, has emerged as a game-changer for E-commerce app development. In this blog, we’ll explore how Flutter can be leveraged to create feature-rich E-commerce apps, discussing essential features, functionalities, and providing code samples to get you started.
Table of Contents
1. Why Choose Flutter for E-commerce Apps?
Before we delve into the essential features and functionalities, let’s understand why Flutter is an excellent choice for developing E-commerce apps:
1.1. Cross-Platform Compatibility
Flutter allows you to develop apps that work seamlessly on both Android and iOS platforms with a single codebase. This significantly reduces development time and effort, ensuring a consistent user experience across devices.
1.2. Fast Development
Flutter’s hot reload feature enables developers to see real-time changes in the app, making the development process faster and more efficient. This rapid development cycle is crucial for E-commerce apps, where quick updates are often necessary.
1.3. Native-Like Performance
Flutter uses the Dart programming language and compiles to native ARM code, resulting in high-performance apps. E-commerce apps demand smooth animations and quick loading times, which Flutter delivers effortlessly.
1.4. Rich UI Components
Flutter provides a wide range of customizable widgets that can be used to create stunning and intuitive user interfaces. This is essential for E-commerce apps to engage users and drive conversions.
1.5. Strong Community and Support
Flutter has a thriving community of developers and extensive documentation, making it easy to find solutions to common issues and stay up-to-date with best practices.
Now that we understand why Flutter is a top choice, let’s explore the essential features and functionalities for E-commerce apps:
2. Essential Features for E-commerce Apps
To create a successful E-commerce app, you need to incorporate key features that enhance the user experience and drive sales. Here are the essential features to consider:
2.1. User Authentication
Implement secure user authentication with options like email, social media logins, or OTP verification. Flutter offers various packages for handling authentication, such as Firebase Authentication.
dart import 'package:firebase_auth/firebase_auth.dart'; final FirebaseAuth _auth = FirebaseAuth.instance; Future<void> signInWithGoogle() async { try { final GoogleSignInAccount googleUser = await GoogleSignIn().signIn(); final GoogleSignInAuthentication googleAuth = await googleUser.authentication; final AuthCredential credential = GoogleAuthProvider.credential( accessToken: googleAuth.accessToken, idToken: googleAuth.idToken, ); await _auth.signInWithCredential(credential); } catch (e) { print("Error during Google Sign-In: $e"); } }
2.2. Product Catalog
Display your product catalog with high-quality images, detailed descriptions, prices, and ratings. Consider using packages like flutter_swiper for creating product carousels.
dart import 'package:flutter_swiper/flutter_swiper.dart'; Swiper( itemBuilder: (BuildContext context, int index) { return Image.network( 'https://example.com/product$imageIndex.jpg', fit: BoxFit.fill, ); }, itemCount: products.length, pagination: SwiperPagination(), control: SwiperControl(), )
2.3. Search and Filters
Integrate a robust search functionality that allows users to find products easily. Implement filters by category, price, brand, and other attributes to help users refine their search.
dart class ProductFilter { String category; double minPrice; double maxPrice; // Other filter attributes }
2.4. Shopping Cart
Enable users to add and remove items from their shopping cart. Use Flutter’s state management solutions like Provider or Riverpod to manage the cart’s state.
dart class ShoppingCart { List<CartItem> items; double totalPrice; void addItem(Product product, int quantity) { // Add item logic } void removeItem(Product product) { // Remove item logic } }
2.5. Checkout and Payment
Implement a secure and user-friendly checkout process. Integrate popular payment gateways like Stripe or PayPal to ensure seamless transactions.
dart class Order { List<CartItem> items; double totalPrice; Address shippingAddress; // Payment details }
2.6. User Reviews and Ratings
Allow users to leave reviews and ratings for products. Display these reviews to build trust and credibility.
dart class ProductReview { String userId; String text; double rating; // Date and time }
2.7. Push Notifications
Send personalized push notifications to inform users about new products, discounts, or order updates. Firebase Cloud Messaging (FCM) is a popular choice for implementing push notifications in Flutter.
dart import 'package:firebase_messaging/firebase_messaging.dart'; final FirebaseMessaging _firebaseMessaging = FirebaseMessaging(); void configurePushNotifications() { _firebaseMessaging.subscribeToTopic('promotions'); }
2.8. Analytics and Insights
Integrate analytics tools like Google Analytics or Firebase Analytics to track user behavior, conversion rates, and other important metrics. Use this data to make informed decisions for your E-commerce app.
dart import 'package:firebase_analytics/firebase_analytics.dart'; final FirebaseAnalytics analytics = FirebaseAnalytics(); void logProductView(Product product) { analytics.logViewItem( itemId: product.id, itemName: product.name, itemCategory: product.category, ); }
3. Advanced Functionalities
Apart from the essential features, consider implementing advanced functionalities to make your E-commerce app stand out:
3.1. Augmented Reality (AR) Shopping
Integrate AR features that allow users to visualize products in their real-world environment. This can boost user engagement and reduce product return rates.
dart // AR integration code goes here
3.2. Personalized Recommendations
Use machine learning algorithms to provide personalized product recommendations based on user preferences and browsing history.
dart // Machine learning integration code goes here
3.3. Multi-Language and Localization
Expand your app’s reach by offering multiple language options and localized content for different regions.
dart // Localization code goes here
3.4. Offline Mode
Implement offline capabilities so users can browse products and access their shopping cart even when they have a poor internet connection.
dart // Offline mode logic goes here
3.5. Social Sharing
Enable users to share their favorite products on social media platforms, increasing your app’s visibility.
dart // Social sharing code goes here
4. Testing and Quality Assurance
Quality assurance is paramount in E-commerce app development. Perform thorough testing, including unit testing, integration testing, and user testing, to ensure your app functions flawlessly. Flutter provides excellent testing support, allowing you to create test cases easily.
dart // Unit testing code samples go here
Conclusion
Flutter empowers developers to build feature-rich E-commerce apps that deliver outstanding user experiences. By incorporating essential features like user authentication, product catalogs, shopping carts, and advanced functionalities like AR shopping and personalized recommendations, you can create a competitive and successful E-commerce app. Remember to prioritize testing and quality assurance to guarantee the reliability and performance of your app. With Flutter, you have the tools and support you need to make your mark in the world of E-commerce.
So, are you ready to take your E-commerce business to the next level with Flutter?
Table of Contents
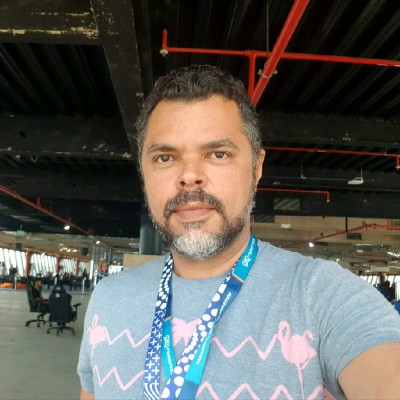
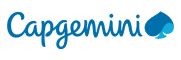