What is the purpose of the FutureBuilder widget in Flutter?
The `FutureBuilder` widget in Flutter serves a crucial role in managing asynchronous operations, specifically dealing with `Future` objects. Its primary purpose is to build a UI based on the result of a `Future`, ensuring a seamless and responsive user experience while handling data that takes time to fetch or compute.
- Asynchronous Operation Handling:`FutureBuilder` is designed to handle asynchronous tasks, such as fetching data from a network or performing time-consuming computations. It efficiently manages the UI by displaying a loading indicator while waiting for the `Future` to complete.
- Dynamic UI Building: The widget dynamically constructs the UI based on the state of the `Future`. It accommodates scenarios where the data might not be available immediately.
- States of FutureBuilder:
– Waiting: Displays a loading indicator while the `Future` is still in progress.
– Error: Handles any errors that may occur during the asynchronous operation.
– Data: Builds the UI with the result from the completed `Future`.
- Example:
```dart Future<int> fetchData() async { // Simulating an asynchronous operation await Future.delayed(Duration(seconds: 2)); return 42; } FutureBuilder<int>( future: fetchData(), builder: (context, snapshot) { switch (snapshot.connectionState) { case ConnectionState.waiting: return CircularProgressIndicator(); case ConnectionState.done: if (snapshot.hasError) { return Text('Error: ${snapshot.error}'); } return Text('Data: ${snapshot.data}'); default: return Container(); // Placeholder for other connection states } }, ) ```
The `FutureBuilder` widget simplifies the implementation of asynchronous workflows in Flutter, allowing developers to create responsive and dynamic user interfaces based on the outcomes of asynchronous operations. By encapsulating the logic for handling different states, it promotes clean and efficient code when dealing with asynchronous tasks in Flutter applications.
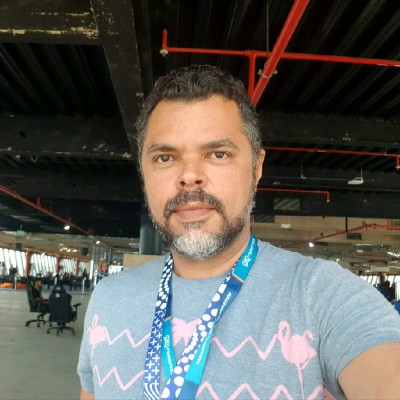
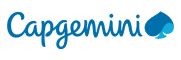