Flutter for Game Development: Building Mobile Games
In the ever-evolving realm of mobile app development, Flutter has emerged as a versatile and powerful framework. While it’s primarily known for crafting beautiful and efficient user interfaces, it has also gained traction in the world of game development. In this blog, we will dive into the exciting world of Flutter for game development and explore how you can create captivating mobile games using this remarkable toolkit.
Table of Contents
1. Why Choose Flutter for Game Development?
Before we delve into the technical aspects of building games with Flutter, let’s address the most pressing question: Why use Flutter for game development? Here are some compelling reasons:
1.1. Cross-Platform Compatibility
Flutter’s cross-platform nature allows you to write code once and run it on both Android and iOS devices. This is a significant advantage as it saves time and effort compared to developing separate games for each platform. Moreover, Flutter provides a consistent user experience across different devices, ensuring your game looks and works the same on every platform.
1.2. Fast Development Cycle
Flutter’s hot reload feature is a game-changer for developers. It enables you to see the results of code changes almost instantly, making the development process faster and more efficient. This rapid feedback loop is invaluable when creating games, where quick iterations are often necessary to fine-tune gameplay and graphics.
1.3. Rich Set of Widgets
Flutter offers a wide range of customizable widgets, making it easy to create complex and interactive game interfaces. You can leverage these widgets to build everything from buttons and menus to in-game HUDs and scoreboards. With Flutter, you have the flexibility to design your game’s UI to match your creative vision.
1.4. Access to Native Features
While Flutter is primarily used for building user interfaces, it doesn’t limit your access to native features. You can still integrate device-specific functionalities such as sensors, cameras, and geolocation into your games. This allows you to create immersive and feature-rich gaming experiences.
Now that we’ve established the advantages of using Flutter for game development, let’s roll up our sleeves and start building a simple mobile game.
2. Setting Up Your Development Environment
Before we begin coding our game, make sure you have Flutter and Dart installed on your development machine. If you haven’t already, follow the official installation guide on the Flutter website.
2.1. Creating a New Flutter Project
Let’s create a new Flutter project for our game. Open your terminal and run the following commands:
bash flutter create my_game cd my_game
This will set up a new Flutter project named “my_game” for you to work on.
3. Designing the Game
Before we start writing code, it’s essential to have a clear plan for our game. Let’s outline the concept for our simple mobile game:
3.1. Game Concept
Our game will be a basic 2D platformer where the player controls a character that can jump to avoid obstacles. The objective is to reach the end of the level while collecting as many coins as possible. The game will have the following features:
- A player character that can move left, right, and jump.
- Platforms for the character to stand on.
- Obstacles that the character must avoid.
- Coins to collect for points.
- A scoring system to track the player’s progress.
With this concept in mind, let’s start implementing our game step by step.
4. Building the Game Interface
The first step in creating our game is to design the user interface (UI). We’ll use Flutter’s widgets to create the game’s visual elements, such as the player character, platforms, and coins.
4.1. Creating the Game Screen
In Flutter, the main game screen is just a widget like any other. Let’s create a simple game screen using the Container widget as the background and a CustomPaint widget to draw the game elements.
dart import 'package:flutter/material.dart'; class GameScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('My Game'), ), body: Container( decoration: BoxDecoration( image: DecorationImage( image: AssetImage('assets/background.png'), // Set your background image fit: BoxFit.cover, ), ), child: CustomPaint( size: Size( MediaQuery.of(context).size.width, MediaQuery.of(context).size.height, ), painter: GamePainter(), ), ), ); } }
In this code, we use the CustomPaint widget to create a canvas for drawing game elements. We’ll define the GamePainter class shortly to handle the drawing.
4.2. Creating the Game Painter
The GamePainter class is responsible for rendering the game elements on the screen. Here’s a basic implementation:
dart class GamePainter extends CustomPainter { @override void paint(Canvas canvas, Size size) { // TODO: Implement drawing game elements } @override bool shouldRepaint(covariant CustomPainter oldDelegate) { return false; } }
Inside the paint method, we’ll draw the player character, platforms, coins, and other game objects. We’ll continue building on this as we progress through the game development process.
5. Implementing Game Logic
With the game interface in place, it’s time to add some interactivity and game logic. We’ll start by defining the player character and enabling basic movement.
5.1. Creating the Player Character
We’ll create a simple player character class that can move left, right, and jump. Here’s the initial implementation:
dart class PlayerCharacter { double x; // X-coordinate of the player double y; // Y-coordinate of the player double velocityX = 0; // Horizontal velocity double velocityY = 0; // Vertical velocity double speed = 5.0; // Movement speed double jumpStrength = -10.0; // Jump strength double gravity = 0.5; // Gravity strength bool isJumping = false; // Flag to track if the player is jumping PlayerCharacter({required this.x, required this.y}); void moveLeft() { velocityX = -speed; } void moveRight() { velocityX = speed; } void jump() { if (!isJumping) { velocityY = jumpStrength; isJumping = true; } } void update() { // Apply gravity velocityY += gravity; // Update position x += velocityX; y += velocityY; // Check for collisions with platforms // TODO: Implement collision detection // Reset jumping flag if the player is on the ground if (y >= groundY) { y = groundY; isJumping = false; } } }
In this code, we define a PlayerCharacter class with properties for position, velocity, speed, jump strength, and gravity. The moveLeft, moveRight, and jump methods allow the player character to move and jump. The update method is responsible for updating the player’s position and handling collisions (which we’ll implement later).
5.2. Handling Player Input
To control the player character, we need to capture user input. Flutter makes this easy with the GestureDetector widget. Let’s add it to our game screen:
dart class GameScreen extends StatelessWidget { final PlayerCharacter player = PlayerCharacter(x: 100, y: groundY); // Initialize player @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('My Game'), ), body: GestureDetector( onTap: () { player.jump(); }, child: Container( decoration: BoxDecoration( image: DecorationImage( image: AssetImage('assets/background.png'), fit: BoxFit.cover, ), ), child: CustomPaint( size: Size( MediaQuery.of(context).size.width, MediaQuery.of(context).size.height, ), painter: GamePainter(player: player), ), ), ), ); } }
In this code, we wrap our game screen with a GestureDetector to capture taps and trigger the player’s jump when the user taps the screen.
5.3. Updating Game State
To keep the game running, we need to update the game state continuously. We can achieve this using a Ticker from Flutter’s flame package. First, add the flame package to your pubspec.yaml file:
yaml dependencies: flutter: sdk: flutter flame: ^1.0.0
Then, create a game loop in your main.dart file:
dart import 'package:flutter/material.dart'; import 'package:flame/game.dart'; import 'package:flame/flame.dart'; void main() async { WidgetsFlutterBinding.ensureInitialized(); await Flame.util.fullScreen(); await Flame.util.setLandscape(); runApp(MyGame().widget); } class MyGame extends BaseGame { @override void render(Canvas canvas) { // TODO: Render game elements } @override void update(double t) { // TODO: Update game logic } }
Now, our game is set up with a game loop. The update method will be called on each frame, allowing us to update the game logic and handle player input.
6. Drawing Game Elements
With the game logic in place, let’s work on rendering the game elements. We’ll start by drawing the player character on the screen.
6.1. Rendering the Player Character
To draw the player character, we’ll extend the BaseGame class from the flame package and use the render method to draw the player sprite. First, create a sprite for the player character by adding an image to your assets folder (e.g., assets/player.png).
dart class MyGame extends BaseGame { late Sprite playerSprite; @override Future<void> onLoad() async { playerSprite = await Sprite.load('player.png'); } @override void render(Canvas canvas) { super.render(canvas); playerSprite.renderRect(canvas, Rect.fromCenter(center: Offset(100, 100), width: 48, height: 48)); // Adjust the position and size } @override void update(double t) { // TODO: Update game logic } }
In this code, we load the player sprite image in the onLoad method and render it on the canvas in the render method. You can adjust the position and size of the player character as needed.
6.2. Rendering Platforms and Obstacles
To complete our game’s visuals, we also need to render platforms and obstacles. You can create sprites for these elements and load them in a similar manner to the player sprite. Then, use the render method to draw them on the canvas at the appropriate positions.
dart class MyGame extends BaseGame { late Sprite playerSprite; late Sprite platformSprite; late Sprite obstacleSprite; @override Future<void> onLoad() async { playerSprite = await Sprite.load('player.png'); platformSprite = await Sprite.load('platform.png'); obstacleSprite = await Sprite.load('obstacle.png'); } @override void render(Canvas canvas) { super.render(canvas); // Render platforms for (var platform in platforms) { platformSprite.renderRect(canvas, platform.rect); } // Render obstacles for (var obstacle in obstacles) { obstacleSprite.renderRect(canvas, obstacle.rect); } // Render the player character playerSprite.renderRect(canvas, player.rect); } @override void update(double t) { // TODO: Update game logic } }
In this code, we assume that platforms and obstacles are lists containing objects representing the platforms and obstacles in the game. Each object should have a rect property specifying its position and size.
7. Implementing Collision Detection
One of the essential aspects of game development is collision detection, which ensures that game objects interact correctly with each other. In our game, we need to check for collisions between the player character and platforms or obstacles.
7.1. Implementing Collision Detection for Platforms
To detect collisions with platforms, we can use Flutter’s built-in Rect class and the overlaps method. Here’s how you can implement collision detection for platforms:
dart bool isCollidingWithPlatform(Rect playerRect, List<Rect> platformRects) { for (var platformRect in platformRects) { if (playerRect.overlaps(platformRect)) { return true; } } return false; }
In this function, playerRect represents the bounding box of the player character, and platformRects is a list of bounding boxes for the platforms. The function checks if there’s any overlap between the player’s bounding box and the platforms’ bounding boxes. If there is an overlap, it returns true, indicating a collision.
7.2. Implementing Collision Detection for Obstacles
Detecting collisions with obstacles is similar to detecting collisions with platforms. You can use the same isCollidingWithPlatform function, but this time, check it against the list of obstacle rectangles.
dart bool isCollidingWithObstacle(Rect playerRect, List<Rect> obstacleRects) { for (var obstacleRect in obstacleRects) { if (playerRect.overlaps(obstacleRect)) { return true; } } return false; }
Now that we have collision detection in place, let’s integrate it into the game logic.
7.3. Updating the Game Logic with Collision Detection
In the update method of our game, we’ll check for collisions between the player character and platforms or obstacles. If a collision is detected, we can respond accordingly, such as stopping the player’s upward motion when hitting the ground or ending the game when colliding with an obstacle.
dart class MyGame extends BaseGame { // ... (previous code) @override void update(double t) { super.update(t); // Update player logic player.update(); // Check for collisions with platforms if (isCollidingWithPlatform(player.rect, platformRects)) { player.velocityY = 0; // Stop vertical motion when colliding with a platform player.isJumping = false; // Reset jumping flag } // Check for collisions with obstacles if (isCollidingWithObstacle(player.rect, obstacleRects)) { // Handle collision with obstacles (e.g., end the game) gameOver = true; } } }
In this code, platformRects and obstacleRects represent lists of bounding boxes for the platforms and obstacles, respectively. When a collision with a platform is detected, we stop the player’s upward motion and reset the jumping flag. When a collision with an obstacle occurs, we can trigger game-over logic, such as displaying a game-over screen or resetting the game.
8. Adding Scoring and Collectibles
To make our game more engaging, let’s implement a scoring system and add collectible coins that the player can pick up.
8.1. Implementing Scoring
To keep track of the player’s score, we can add a score variable to our game and increase it whenever the player collects a coin. Here’s how you can implement scoring:
dart class MyGame extends BaseGame { int score = 0; // Initialize the score @override void render(Canvas canvas) { super.render(canvas); // Render the player character playerSprite.renderRect(canvas, player.rect); // Render collectible coins for (var coin in coins) { coinSprite.renderRect(canvas, coin.rect); } // Render the score final textStyle = TextStyle( color: Colors.white, fontSize: 24.0, ); final textSpan = TextSpan( text: 'Score: $score', style: textStyle, ); final textPainter = TextPainter( text: textSpan, textDirection: TextDirection.ltr, ); textPainter.layout(); textPainter.paint(canvas, Offset(16.0, 16.0)); } @override void update(double t) { super.update(t); // Update player logic player.update(); // Check for collisions with coins final collectedCoins = coins.where((coin) => player.rect.overlaps(coin.rect)).toList(); for (var coin in collectedCoins) { coins.remove(coin); // Remove the collected coin score += 10; // Increase the score } } }
In this code, we maintain a score variable and update it when the player collects coins. We also render the score on the game screen using Flutter’s TextPainter and TextStyle.
8.2. Adding Collectible Coins
To add collectible coins to the game, create a sprite for the coin and generate random positions for them. Here’s how you can implement coin generation:
dart class MyGame extends BaseGame { // ... (previous code) late Sprite coinSprite; final List<Rect> coins = []; // List to store coin rectangles @override Future<void> onLoad() async { playerSprite = await Sprite.load('player.png'); platformSprite = await Sprite.load('platform.png'); obstacleSprite = await Sprite.load('obstacle.png'); coinSprite = await Sprite.load('coin.png'); // Load the coin sprite // Generate initial coin positions generateCoins(); } void generateCoins() { // Generate coins at random positions for (var i = 0; i < 10; i++) { // Generate 10 coins final randomX = Random().nextInt(300) + 50; // Random X-coordinate final randomY = Random().nextInt(400) + 100; // Random Y-coordinate final coinRect = Rect.fromLTWH(randomX.toDouble(), randomY.toDouble(), 24.0, 24.0); // Adjust the size coins.add(coinRect); // Add the coin to the list } } @override void update(double t) { super.update(t); // Update player logic player.update(); // Check for collisions with coins final collectedCoins = coins.where((coin) => player.rect.overlaps(coin)).toList(); for (var coin in collectedCoins) { coins.remove(coin); // Remove the collected coin score += 10; // Increase the score } // Check if all coins are collected if (coins.isEmpty) { generateCoins(); // Generate new coins } } }
In this code, we load the coin sprite in the onLoad method and generate initial coin positions using the generateCoins function. We also update the game logic to remove collected coins from the list and generate new coins when all coins are collected.
Conclusion
Congratulations! You’ve just embarked on the journey of game development with Flutter. In this blog, we covered the essential steps to get started with creating a simple mobile game using Flutter:
- Setting up your development environment: We ensured that you have Flutter and Dart installed and created a new Flutter project.
- Designing the game: We outlined the concept for our simple 2D platformer game, including player controls, platforms, obstacles, coins, and scoring.
- Building the game interface: We designed the game screen and implemented the GamePainter class to render game elements on the screen.
- Implementing game logic: We created the player character, handled player input, and updated the game state using a game loop.
- Drawing game elements: We rendered the player character, platforms, obstacles, and collectible coins on the screen.
- Implementing collision detection: We implemented collision detection for platforms and obstacles, ensuring that the player character interacts correctly with the game environment.
- Adding scoring and collectibles: We added a scoring system and collectible coins to make the game more engaging and rewarding for players.
As you continue your journey into game development with Flutter, you can expand on these concepts to create more complex and exciting games. Experiment with different game mechanics, graphics, and levels to unleash your creativity and deliver unique gaming experiences to your players.
Now, it’s your turn to take this foundation and build your own incredible mobile games with Flutter. Happy game development!
Table of Contents
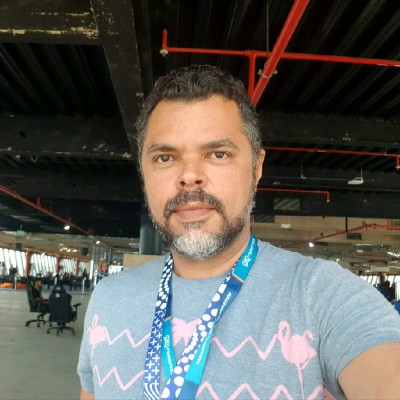
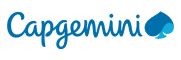