Flutter and Maps: Integrating Geolocation Services in Apps
In today’s mobile app landscape, location-based features have become indispensable. Whether you’re building a fitness app, a delivery service, or a travel guide, integrating geolocation services can elevate the user experience to a whole new level. Flutter, Google’s open-source UI toolkit, has gained immense popularity for its cross-platform capabilities, and when combined with Google Maps, it becomes a powerful tool for incorporating geolocation services into your apps.
Table of Contents
This blog post will guide you through the process of integrating geolocation services into your Flutter app using Google Maps. We’ll explore everything from setting up the project to handling user permissions and displaying interactive maps. So, let’s dive in!
1. Getting Started with Flutter and Google Maps
1.1. Setting up a Flutter Project
If you haven’t already, install Flutter on your development machine and create a new Flutter project:
bash flutter create my_maps_app cd my_maps_app
1.2. Adding Google Maps Dependencies
To integrate Google Maps into your Flutter project, add the following dependencies to your ‘pubspec.yaml’ file:
yaml dependencies: flutter: sdk: flutter google_maps_flutter: ^2.0.8
Don’t forget to run flutter pub get to fetch the newly added dependencies.
2. Obtaining API Keys
To use Google Maps services, you’ll need API keys. Here’s how you can obtain them:
- Creating a Google Cloud Project
- Go to the Google Cloud Console.
- Create a new project or select an existing one.
- Enabling the Maps SDK
- In the Cloud Console, navigate to the “APIs & Services” > “Library” section.
- Search for “Maps SDK for Android” and “Maps SDK for iOS” and enable them for your project.
- Go to “APIs & Services” > “Credentials” and create an API key.
3. Requesting Location Permissions
3.1. Android Permissions
In your AndroidManifest.xml file, add the following permissions:
xml <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
3.2. iOS Permissions
For iOS, open your Info.plist file and add the following keys:
xml <key>NSLocationWhenInUseUsageDescription</key> <string>We need your location for...</string> <key>NSLocationAlwaysUsageDescription</key> <string>We need your location for...</string>
Make sure to provide appropriate descriptions explaining why your app needs access to the user’s location.
4. Displaying a Map
4.1. Initializing Google Maps
In your Flutter code, initialize Google Maps by adding a GoogleMap widget:
dart import 'package:google_maps_flutter/google_maps_flutter.dart'; GoogleMap( initialCameraPosition: CameraPosition( target: LatLng(37.7749, -122.4194), // San Francisco coordinates zoom: 13.0, ), onMapCreated: (GoogleMapController controller) { // You can interact with the map controller here. }, )
This code sets up a basic Google Map centered on San Francisco with an initial zoom level of 13. You can customize the target and zoom parameters as per your requirements.
4.2. Customizing the Map
You can customize the map’s appearance using various options. For instance, to change the map type to satellite view:
dart GoogleMap( mapType: MapType.satellite, // Change map type to satellite // ... )
5. Adding Markers and User Location
5.1. Placing Markers on the Map
Markers are essential for highlighting specific locations on the map. Here’s how to add a marker:
dart Marker( markerId: MarkerId('marker_1'), position: LatLng(37.7749, -122.4194), // Marker's coordinates infoWindow: InfoWindow( title: 'San Francisco', snippet: 'A beautiful city by the bay.', ), )
You can add multiple markers to pinpoint various locations on the map.
5.2. Displaying User’s Current Location
To display the user’s current location on the map, use the MyLocationButton widget:
dart GoogleMap( myLocationEnabled: true, // Show the my location button myLocationButtonEnabled: true, // Enable the my location button // ... )
6. Handling Map Interactions
6.1. Listening to Map Events
You can listen to various map events, such as when the user taps on the map or when the camera position changes:
dart GoogleMap( onMapCreated: (GoogleMapController controller) { // Add event listeners here controller.onMarkerTapped.add((marker) { // Handle marker tap event }); controller.onCameraMove.add((position) { // Handle camera position change event }); }, // ... )
6.2. Geocoding and Reverse Geocoding
With the help of geocoding and reverse geocoding, you can convert between addresses and coordinates. Here’s a sample code for geocoding:
dart final GeocodingResponse response = await geocoding.searchByLocation( Location(latitude: 37.7749, longitude: -122.4194), ); if (response.status == GeocodingStatus.OK) { final first = response.results.first; print("Formatted Address: ${first.formattedAddress}"); }
And for reverse geocoding:
dart final ReverseGeocodingResponse response = await geocoding.searchByAddress('San Francisco'); if (response.status == GeocodingStatus.OK) { final first = response.results.first; print("Coordinates: ${first.geometry.location.lat}, ${first.geometry.location.lng}"); }
7. Best Practices for Geolocation Services in Flutter
- Battery Optimization: Be mindful of location updates’ frequency to conserve device battery.
- User Privacy: Always request location permissions with a clear explanation of why they are needed.
- Error Handling: Implement error handling for scenarios where location services are unavailable or permission is denied.
- Testing: Thoroughly test your app on different devices and scenarios to ensure reliable location functionality.
Conclusion
In conclusion, integrating geolocation services into your Flutter app using Google Maps can greatly enhance its functionality and user experience. By following the steps outlined in this guide and adhering to best practices, you can create location-aware apps that provide valuable services to your users.
Whether you’re building a navigation app, a location-based game, or a delivery tracking system, the combination of Flutter and Google Maps offers a robust platform for creating innovative and engaging applications. So, go ahead and give it a try, and watch your app take on new dimensions with geolocation services!
Remember, the world is at your fingertips with Flutter and Google Maps. Happy coding!
Table of Contents
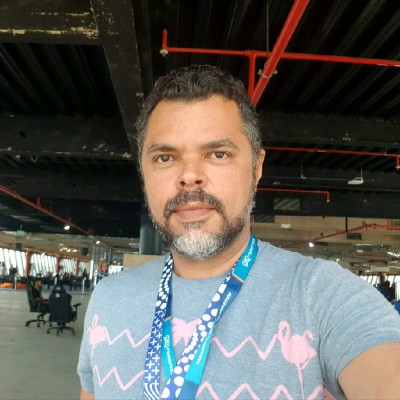
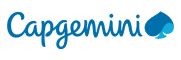