Exploring Flutter’s Gesture Recognition: Interacting with Users
In the realm of mobile app development, creating engaging and interactive user interfaces is essential. Flutter, Google’s UI toolkit, provides a robust gesture recognition system that allows developers to make apps respond to user actions seamlessly. Whether it’s a simple tap, a swipe, or a pinch, Flutter’s gesture recognition system empowers developers to enhance the user experience.
Table of Contents
In this blog post, we’ll take a deep dive into Flutter’s gesture recognition capabilities, exploring various types of gestures, touch events, and providing code samples to illustrate how to implement them effectively. By the end of this article, you’ll have a solid understanding of how to leverage Flutter’s gesture recognition to create interactive and user-friendly applications.
1. Understanding Gesture Recognition
Before we delve into the code and implementation details, let’s start by understanding what gesture recognition is and why it’s crucial in app development.
1.1. What Are Gestures?
Gestures are touch-based actions performed by users on a touchscreen device. These actions can include tapping, swiping, pinching, zooming, and more. Recognizing and responding to these gestures is essential for creating intuitive and responsive user interfaces.
1.2. Why Gesture Recognition Matters
Gesture recognition is essential for several reasons:
- Intuitive User Experience: Gestures mimic real-world actions, making your app more intuitive and user-friendly. Users can interact with your app in a way that feels natural to them.
- Efficiency: Gestures often allow users to perform actions more quickly than traditional button clicks. For example, swiping to delete an item is faster than selecting it and then tapping a delete button.
- Enhanced Interactivity: Gestures can add an extra layer of interactivity to your app, making it more engaging and enjoyable to use.
Now that we understand the importance of gesture recognition, let’s explore how to implement it in Flutter.
2. Types of Gestures in Flutter
Flutter provides various types of gestures, each serving a specific purpose. Let’s discuss some of the most common ones:
2.1. Tap Gesture
The GestureDetector widget in Flutter allows you to detect a simple tap gesture. This gesture is triggered when the user taps on a widget.
dart GestureDetector( onTap: () { // Handle the tap gesture }, child: Container( color: Colors.blue, width: 100, height: 100, child: Center( child: Text( 'Tap Me', style: TextStyle(color: Colors.white), ), ), ), )
In this example, the onTap callback is triggered when the user taps the Container.
2.2. Long Press Gesture
The long press gesture is triggered when the user presses and holds a widget for a specified duration. You can use the onLongPress property of GestureDetector to handle this gesture.
dart GestureDetector( onLongPress: () { // Handle the long press gesture }, child: Container( color: Colors.red, width: 100, height: 100, child: Center( child: Text( 'Long Press Me', style: TextStyle(color: Colors.white), ), ), ), )
In this example, the onLongPress callback is triggered when the user long-presses the Container.
2.3. Double Tap Gesture
Double tap gestures are triggered when the user quickly taps a widget twice. You can handle this gesture using the onDoubleTap property of GestureDetector.
dart GestureDetector( onDoubleTap: () { // Handle the double tap gesture }, child: Container( color: Colors.green, width: 100, height: 100, child: Center( child: Text( 'Double Tap Me', style: TextStyle(color: Colors.white), ), ), ), )
In this example, the onDoubleTap callback is triggered when the user double-taps the Container.
2.4. Swipe Gesture
Swiping gestures are commonly used for actions like scrolling through a list or navigating between pages. Flutter provides the onHorizontalDrag and onVerticalDrag properties of GestureDetector to handle swipe gestures.
dart GestureDetector( onHorizontalDragUpdate: (details) { // Handle horizontal swipe gesture }, onVerticalDragUpdate: (details) { // Handle vertical swipe gesture }, child: Container( color: Colors.yellow, width: 100, height: 100, child: Center( child: Text( 'Swipe Me', style: TextStyle(color: Colors.black), ), ), ), )
In this example, you can handle horizontal and vertical swipe gestures separately using the onHorizontalDragUpdate and onVerticalDragUpdate callbacks.
2.5. Pinch and Zoom Gesture
Pinch and zoom gestures are used for scaling and zooming in on content. Flutter provides the onScaleUpdate property of GestureDetector to handle these gestures.
dart GestureDetector( onScaleUpdate: (details) { // Handle pinch and zoom gestures // Use details.scale to determine the scale factor }, child: Container( color: Colors.orange, width: 200, height: 200, child: Center( child: Text( 'Pinch Me', style: TextStyle(color: Colors.white), ), ), ), )
In this example, you can use the details.scale property to determine the scale factor of the pinch and zoom gesture.
3. Handling Gesture Events
Now that we’ve discussed various types of gestures in Flutter, let’s dive into handling gesture events. When a gesture is detected, Flutter provides gesture event details that you can use to respond to the user’s action.
3.1. Gesture Event Properties
Here are some common properties available in gesture event details:
- globalPosition: The global position of the pointer when the gesture event occurred.
- localPosition: The local position of the pointer within the widget where the gesture occurred.
- delta: The difference in position from the previous gesture event. This is often used to calculate the velocity of a swipe gesture.
- scale: The scale factor for pinch and zoom gestures.
- rotation: The rotation angle for rotation gestures.
3.2. Handling Tap Gesture Events
To handle tap gestures, you can use the onTap property of GestureDetector. The onTap callback is called when the user taps the widget. You can access the gesture event details, such as the globalPosition and localPosition, inside the callback.
dart GestureDetector( onTap: (TapUpDetails details) { final globalPosition = details.globalPosition; final localPosition = details.localPosition; // Handle tap gesture using gesture event details }, child: // Your widget here )
3.3. Handling Swipe Gesture Events
To handle swipe gestures, you can use the onHorizontalDragUpdate and onVerticalDragUpdate properties of GestureDetector. These callbacks are called when the user swipes horizontally or vertically. You can access the gesture event details, such as the delta, to determine the direction and speed of the swipe.
dart GestureDetector( onHorizontalDragUpdate: (DragUpdateDetails details) { final delta = details.delta; // Handle horizontal swipe gesture using gesture event details }, onVerticalDragUpdate: (DragUpdateDetails details) { final delta = details.delta; // Handle vertical swipe gesture using gesture event details }, child: // Your widget here )
3.4. Handling Pinch and Zoom Gesture Events
To handle pinch and zoom gestures, you can use the onScaleUpdate property of GestureDetector. This callback is called when the user performs a pinch or zoom gesture. You can access the gesture event details, such as the scale, to determine the scale factor of the gesture.
dart GestureDetector( onScaleUpdate: (ScaleUpdateDetails details) { final scale = details.scale; // Handle pinch and zoom gesture using gesture event details }, child: // Your widget here )
4. Implementing Complex Gesture Interactions
In addition to handling individual gesture events, Flutter allows you to implement complex gesture interactions by combining multiple gestures. Here are some examples of complex interactions:
4.1. Drag-and-Drop
Implementing drag-and-drop functionality requires handling both the drag gesture and the drop gesture. You can use the onHorizontalDragDown, onHorizontalDragUpdate, and onHorizontalDragEnd properties to track the drag, and then use the onDrop property to handle the drop event.
dart GestureDetector( onHorizontalDragDown: (DragDownDetails details) { // Handle drag-down event }, onHorizontalDragUpdate: (DragUpdateDetails details) { // Handle drag-update event }, onHorizontalDragEnd: (DragEndDetails details) { // Handle drag-end event }, onDrop: () { // Handle drop event }, child: // Your draggable widget here )
4.2. Gesture Sequences
You can also implement gesture sequences, where a specific sequence of gestures triggers an action. For example, you might want to perform an action when the user swipes left, then right in quick succession. You can use a combination of gesture properties and state management to achieve this.
dart bool swipeLeftDetected = false; GestureDetector( onHorizontalDragUpdate: (DragUpdateDetails details) { final delta = details.delta; if (delta.dx > 0 && !swipeLeftDetected) { // User is swiping right swipeLeftDetected = true; } else if (delta.dx < 0 && swipeLeftDetected) { // User is swiping left after swiping right // Handle the desired action } }, onHorizontalDragEnd: (DragEndDetails details) { // Reset the state after the gesture is complete swipeLeftDetected = false; }, child: // Your widget here )
4.3. Combining Gestures
In some cases, you might want to combine multiple gestures to trigger an action. For example, you can require the user to pinch and zoom with two fingers while long-pressing to activate a specific feature.
dart bool pinchAndLongPressDetected = false; GestureDetector( onScaleUpdate: (ScaleUpdateDetails details) { final scale = details.scale; // Handle pinch and zoom gesture }, onLongPress: () { if (pinchAndLongPressDetected) { // Activate the feature } }, child: // Your widget here )
By combining gestures, you can create unique and interactive user experiences in your Flutter app.
Conclusion
Flutter’s gesture recognition system empowers developers to create highly interactive and user-friendly applications. Whether you need to handle simple tap gestures or complex multi-step interactions, Flutter provides the tools and flexibility to implement a wide range of gestures.
In this blog post, we explored various types of gestures, discussed how to handle gesture events, and even delved into implementing complex interactions by combining gestures. Armed with this knowledge, you can now take your Flutter app development skills to the next level and create engaging user experiences.
Remember that the key to successful gesture recognition is to make your app respond naturally to user actions, enhancing the overall user experience. So, go ahead, experiment with gestures, and create apps that users will love to interact with!
Table of Contents
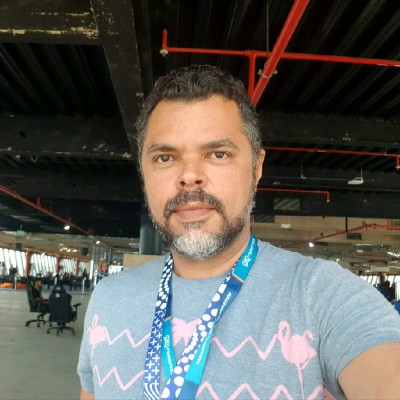
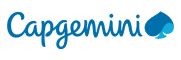