What is the purpose of the GlobalKey in Flutter?
The `GlobalKey` in Flutter serves as a unique identifier for widgets across the widget tree, allowing developers to access and manipulate the state of a specific widget from anywhere in the application. It is particularly useful when you need to reference a widget and its associated state outside the widget tree where it is declared. Here are key points and an example to illustrate its purpose:
- Uniqueness: GlobalKey ensures the uniqueness of a widget within the widget tree, preventing conflicts when trying to access or update its state.
- Global Accessibility: It provides a way to access a widget from different parts of the application, such as from a parent widget or even a different screen.
- State Preservation: GlobalKey is often used to preserve and restore the state of a widget when rebuilding the widget tree. This is especially valuable when dealing with forms or complex UI elements.
- Key for Widgets: While it can be used with various Flutter classes, GlobalKey is commonly associated with StatefulWidgets, enabling the retrieval of the corresponding State object.
Example:
Suppose you have a `Form` widget in your Flutter application, and you want to reset the form from a button placed outside the widget tree. You can use `GlobalKey<FormState>` to achieve this:
```dart class MyForm extends StatefulWidget { // Creating a GlobalKey for the Form widget static final GlobalKey<FormState> formKey = GlobalKey<FormState>(); @override _MyFormState createState() => _MyFormState(); } class _MyFormState extends State<MyForm> { @override Widget build(BuildContext context) { return Form( key: MyForm.formKey, // Associating GlobalKey with Form widget child: Column( children: [ // Form fields go here // ... ], ), ); } } // Outside the widget tree, you can reset the form using the GlobalKey void resetForm() { if (MyForm.formKey.currentState != null) { MyForm.formKey.currentState!.reset(); } } ```
In this example, the GlobalKey allows the `resetForm` function to access and reset the state of the `Form` widget, even though it is located outside the widget tree where the form is defined.
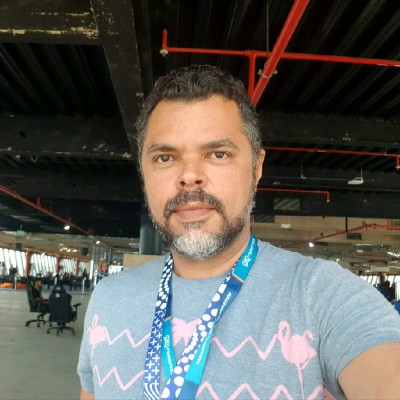
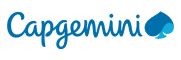