Exploring Flutter’s Image and Video Processing: Filters and Effects
Flutter, Google’s UI toolkit for building natively compiled applications, offers powerful capabilities for image and video processing. This article explores how you can leverage Flutter’s features to apply filters, effects, and more to enhance your media content, making your applications visually appealing and engaging.
Understanding Image and Video Processing in Flutter
Image and video processing in Flutter involves manipulating media content to apply effects, filters, and enhancements. By using Flutter’s plugins and packages, developers can achieve a variety of media transformations that improve user experience and visual appeal.
Using Flutter for Advanced Image and Video Processing
Flutter provides several plugins and libraries that make it easy to work with images and videos. Here are key aspects and examples demonstrating how Flutter can be employed for image and video processing.
1. Applying Filters to Images
Flutter’s image processing capabilities allow you to apply various filters to images, such as color adjustments, blurring, and sharpening.
Example: Using the `image` Package
- Add the `image` Package
Add the `image` package to your `pubspec.yaml` file:
```yaml dependencies: image: ^3.0.1 ```
- Load and Process an Image
Use the `image` package to load and apply filters to an image:
```dart import 'package:image/image.dart' as img; // Load an image img.Image image = img.decodeImage(File('path_to_image').readAsBytesSync())!; // Apply a blur filter img.Image blurredImage = img.gaussianBlur(image, 10); // Save the processed image File('path_to_processed_image').writeAsBytesSync(img.encodeJpg(blurredImage)); ```
- Display the Processed Image
Use the `Image.file` widget to display the processed image in your Flutter app:
```dart Image.file(File('path_to_processed_image')); ```
2. Applying Effects to Videos
Flutter allows for video processing and effects using various packages and techniques.
Example: Using the `video_player` and `flutter_ffmpeg` Packages
- Add the Packages
Include `video_player` and `flutter_ffmpeg` in your `pubspec.yaml` file:
```yaml dependencies: video_player: ^2.5.0 flutter_ffmpeg: ^0.4.0 ```
- Play and Process a Video
Use `flutter_ffmpeg` to apply effects to a video:
```dart import 'package:flutter_ffmpeg/flutter_ffmpeg.dart'; final FlutterFFmpeg _flutterFFmpeg = FlutterFFmpeg(); // Apply a video effect _flutterFFmpeg.execute('-i input.mp4 -vf "scale=1280:720,eq=brightness=0.1" output.mp4'); ```
- Play the Processed Video
Use the `video_player` package to play the processed video:
```dart import 'package:video_player/video_player.dart'; VideoPlayerController _controller = VideoPlayerController.file(File('path_to_processed_video')) ..initialize().then((_) { setState(() {}); }); @override Widget build(BuildContext context) { return AspectRatio( aspectRatio: _controller.value.aspectRatio, child: VideoPlayer(_controller), ); } ```
3. Creating Custom Filters and Effects
For advanced custom filters and effects, Flutter allows you to create your own using custom shaders and image manipulation techniques.
Example: Using `flutter_shaders` for Custom Filters
- Add the `flutter_shaders` Package
Add the `flutter_shaders` package to your `pubspec.yaml` file:
```yaml dependencies: flutter_shaders: ^0.1.0 ```
- Create and Apply a Custom Shader
Implement a custom shader to apply a filter:
```dart import 'package:flutter_shaders/flutter_shaders.dart'; final shader = Shader.fromAsset('path_to_shader_file.frag'); // Apply the shader to an image Widget build(BuildContext context) { return ShaderMask( shaderCallback: (Rect bounds) { return shader.createShader(bounds); }, child: Image.asset('path_to_image'), ); } ```
Conclusion
Flutter’s capabilities for image and video processing open up a wide range of possibilities for enhancing your media content. By applying filters, effects, and creating custom shaders, you can significantly improve the visual appeal of your applications.
Further Reading:
Table of Contents
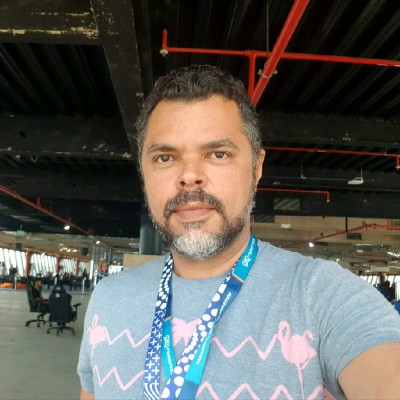
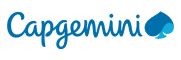