How does Flutter handle assets like images and fonts?
In Flutter, efficient asset management, including images and fonts, is crucial for creating visually appealing and responsive applications. Here’s a comprehensive explanation of how Flutter handles assets:
- Asset Folder Structure:
– Flutter uses a designated folder structure to organize assets, typically named `assets`.
– Within this folder, you can create subdirectories for images (`images/`) and fonts (`fonts/`), maintaining a structured approach.
- Declaring Assets in `pubspec.yaml`:
– The `pubspec.yaml` file is where you declare your project dependencies and assets.
– For images, you specify the path to the image file:
```yaml flutter: assets: - assets/images/my_image.png ```
– For fonts, you define the font family and its weight/style:
```yaml flutter: fonts: - family: myFont fonts: - asset: assets/fonts/my_font.ttf ```
- Image Rendering with `Image.asset()`:
– To display an image, you can use the `Image.asset()` widget, referencing the asset path:
```dart Image.asset('assets/images/my_image.png'); ```
- Font Integration:
– Flutter allows you to integrate custom fonts seamlessly. After declaring the font in `pubspec.yaml`, you can use it in your text widgets:
```dart Text( 'Hello, Flutter!', style: TextStyle(fontFamily: 'myFont'), ); ```
- Pre-caching Assets:
– To optimize performance, Flutter provides the `precacheImage()` function to preload images before they are displayed, reducing latency.
```dart precacheImage(AssetImage('assets/images/my_image.png'), context); ```
- Asset Bundling:
– During the build process, Flutter bundles assets, making them accessible at runtime without the need for additional configuration.
Efficiently managing assets is integral to Flutter development. By adhering to the folder structure and utilizing `pubspec.yaml` declarations, developers can seamlessly integrate images and fonts into their applications, ensuring a visually engaging user experience.
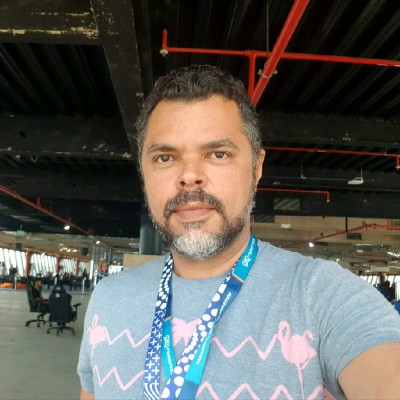
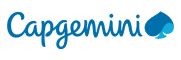