Can you integrate Firebase with Flutter?
Integrating Firebase with Flutter is a seamless process that enhances the functionality and features of your mobile applications. Firebase, a comprehensive mobile and web app development platform by Google, offers a variety of services like real-time database, authentication, cloud storage, and more. Here’s a detailed explanation:
- Dependency Setup:
– Begin by adding the necessary Firebase dependencies to your `pubspec.yaml` file.
```yaml dependencies: firebase_core: ^latest_version firebase_auth: ^latest_version cloud_firestore: ^latest_version // Add other Firebase services as needed ```
- Initialization:
– Initialize Firebase in your Flutter app using `Firebase.initializeApp()` in the `main()` function or the `initState()` method.
```dart void main() async { WidgetsFlutterBinding.ensureInitialized(); await Firebase.initializeApp(); runApp(MyApp()); } ```
- Authentication:
– Implement user authentication using `FirebaseAuth` for features like email/password login, Google Sign-In, etc.
```dart final FirebaseAuth _auth = FirebaseAuth.instance; Future<void> signInWithGoogle() async { // Implement Google Sign-In using Firebase } ```
- Real-time Database:
– Utilize Firebase’s Cloud Firestore for real-time data synchronization. Here’s an example of reading and writing data:
```dart final FirebaseFirestore _firestore = FirebaseFirestore.instance; Future<void> addData() { return _firestore.collection('example').add({'key': 'value'}); } StreamBuilder( stream: _firestore.collection('example').snapshots(), builder: (context, snapshot) { // Handle real-time updates }, ); ```
- Cloud Storage:
– For storing and retrieving files, Firebase Cloud Storage can be integrated easily:
```dart final FirebaseStorage _storage = FirebaseStorage.instance; Future<void> uploadFile() { // Upload a file to Cloud Storage } Future<void> downloadFile() { // Download a file from Cloud Storage } ```
Integrating Firebase with Flutter empowers your app with a robust backend, real-time capabilities, and efficient data storage. Whether it’s user authentication, database management, or file storage, Firebase provides a comprehensive set of tools for building modern and feature-rich applications.
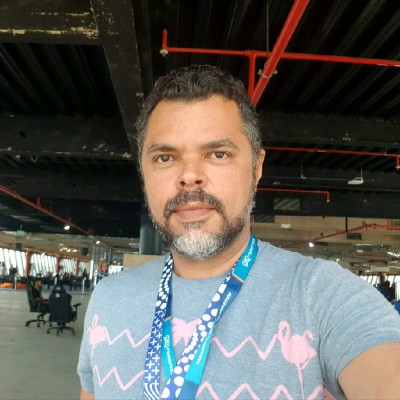
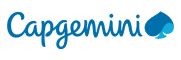