Flutter for Machine Learning: Integrating TensorFlow in Apps
In the ever-evolving landscape of mobile app development, staying ahead of the curve is crucial. One way to achieve this is by incorporating machine learning capabilities into your Flutter apps. Machine learning can empower your applications with intelligent features, from image recognition to natural language processing. And when it comes to machine learning in Flutter, TensorFlow is the go-to framework.
Table of Contents
In this comprehensive guide, we’ll delve into the exciting world of Flutter and TensorFlow integration. You’ll discover how to harness the full potential of machine learning within your Flutter apps through step-by-step instructions and code samples.
1. Why TensorFlow for Machine Learning?
Before we dive into the nitty-gritty details of integrating TensorFlow into your Flutter apps, let’s understand why TensorFlow is the preferred choice for many machine learning tasks.
1.1. Versatility and Flexibility
TensorFlow, an open-source machine learning framework developed by Google, is renowned for its versatility and flexibility. It supports a wide range of machine learning models, including neural networks, and is compatible with multiple programming languages, making it an excellent choice for Flutter developers.
1.2. Pre-Trained Models
One of TensorFlow’s significant advantages is its library of pre-trained machine learning models. These models cover various use cases, such as image recognition, object detection, and natural language understanding. Leveraging pre-trained models can save you a considerable amount of time and effort in building ML-powered apps.
1.3. Active Community
TensorFlow boasts a vast and active community of developers, which means you’ll have access to extensive documentation, tutorials, and support. This can be invaluable when you run into issues or need guidance on complex machine learning tasks.
Now that we’ve highlighted the advantages of TensorFlow, let’s get started with integrating it into your Flutter apps.
2. Setting Up Your Development Environment
Before you can begin integrating TensorFlow into your Flutter app, you’ll need to set up your development environment. Ensure you have the following prerequisites in place:
2.1. Flutter Installed
If you haven’t already, install Flutter on your development machine. You can find detailed installation instructions on the official Flutter website.
2.2. Android Studio or Xcode
Depending on whether you’re targeting Android or iOS, make sure you have either Android Studio or Xcode installed.
2.3. TensorFlow Flutter Package
To use TensorFlow in your Flutter app, you’ll need to add the TensorFlow Flutter package to your project. Open your pubspec.yaml file and add the following dependency:
yaml dependencies: flutter: sdk: flutter tensorflow_lite_flutter: ^0.7.0 # Check for the latest version on pub.dev
Save the file and run ‘flutter pub get’ to fetch the package.
3. Integrating TensorFlow in Your Flutter App
With your development environment set up, it’s time to integrate TensorFlow into your Flutter app. We’ll walk through a simple example of using TensorFlow Lite to perform image classification.
3.1. Prepare Your Model
Before you can use TensorFlow for image classification, you need a pre-trained model. You can find various pre-trained models for image classification on TensorFlow’s official website. Once you have your model, convert it to TensorFlow Lite format using the TensorFlow Converter.
python import tensorflow as tf # Load your pre-trained model model = tf.keras.models.load_model('your_model.h5') # Convert the model to TensorFlow Lite format converter = tf.lite.TFLiteConverter.from_keras_model(model) tflite_model = converter.convert() # Save the TensorFlow Lite model with open('model.tflite', 'wb') as f: f.write(tflite_model)
3.2. Add TensorFlow Lite to Your Flutter App
Now that you have your TensorFlow Lite model, add it to your Flutter app. Place the model.tflite file in your Flutter project’s assets folder.
3.3. Load and Run the Model in Flutter
Next, you’ll need to load and run the TensorFlow Lite model in your Flutter app. Here’s a basic example of how to do this:
dart import 'package:flutter/material.dart'; import 'package:tflite_flutter/tflite_flutter.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { Interpreter? interpreter; @override void initState() { super.initState(); loadModel(); } Future<void> loadModel() async { interpreter = await Interpreter.fromAsset('model.tflite'); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Image Classification'), ), body: Center( child: Text('Image Classification Result Will Appear Here'), ), ); } }
In this code snippet, we’ve created a simple Flutter app with an empty container to display the image classification result. We load the TensorFlow Lite model using the Interpreter.fromAsset method from the ‘tflite_flutter package’.
3.4. Perform Image Classification
Now that you have your TensorFlow Lite model loaded, you can perform image classification. You’ll need to preprocess the input image and pass it to the model for inference. Here’s a basic example:
dart import 'dart:typed_data'; Future<void> classifyImage(Uint8List imageBytes) async { // Preprocess the image (resize, normalize, etc.) // ... // Perform inference final input = interpreter.getInputTensors()[0]; final output = interpreter.getOutputTensors()[0]; interpreter.run( input: input, output: output, ); // Process the output (get class probabilities, labels, etc.) // ... }
This code snippet demonstrates the image classification process. You’ll need to preprocess the input image to match the model’s input requirements and then run inference using the interpreter.run method.
4. Real-World Use Cases
Now that you have a basic understanding of how to integrate TensorFlow into your Flutter app let’s explore some real-world use cases where machine learning can add significant value:
4.1. Image Recognition
Imagine building a Flutter app that can recognize objects in photos taken by the user. With TensorFlow, you can train your model to identify various objects, from animals to everyday objects. Users can simply point their phone’s camera at an object, and the app can provide information about it.
4.2. Language Translation
Integrating machine learning can also enhance your Flutter app’s language translation capabilities. You can create a translation app that not only translates text but also understands context to provide more accurate translations.
4.3. Personalized Recommendations
Machine learning can power personalized recommendation systems in your app. Whether you’re developing an e-commerce app or a content platform, TensorFlow can analyze user behavior and preferences to recommend products or content tailored to each user.
4.4. Natural Language Processing
For apps involving text input and output, such as chatbots or virtual assistants, TensorFlow can be a game-changer. You can use NLP models to understand and generate human-like text responses, improving user engagement and interaction.
5. Best Practices for TensorFlow in Flutter
As you embark on your journey to integrate TensorFlow into your Flutter apps, keep these best practices in mind:
5.1. Model Size Optimization
TensorFlow Lite allows you to optimize your models for mobile devices, reducing their size while maintaining performance. Consider optimizing your models to ensure they don’t consume excessive storage space on users’ devices.
5.2. Test on Real Devices
Always test your TensorFlow-powered Flutter app on real devices to ensure optimal performance. Emulators can provide some insight, but real-world testing is essential to identify any issues.
5.3. Error Handling
Machine learning models can produce unexpected results or errors. Implement robust error handling in your app to gracefully handle issues that may arise during inference.
5.4. Keep Models Updated
If your app relies on machine learning models for critical functionality, be prepared to update the models regularly. This ensures your app remains accurate and up-to-date in its predictions.
Conclusion
Integrating TensorFlow into your Flutter apps opens up a world of possibilities for machine learning-powered features. Whether you’re developing image recognition, language translation, or recommendation systems, TensorFlow provides the tools and flexibility to make it happen. With the steps and best practices outlined in this guide, you’re well on your way to building intelligent and engaging Flutter applications.
Now, it’s time to roll up your sleeves, start experimenting, and unlock the potential of machine learning in your Flutter projects. Happy coding!
Table of Contents
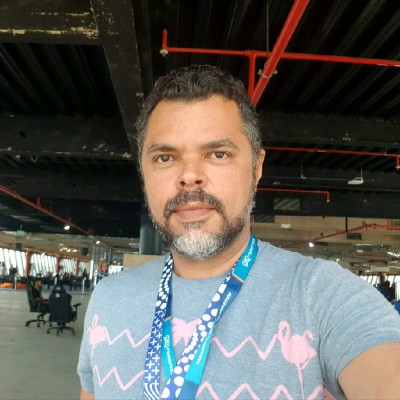
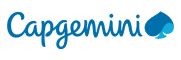