Continuous Integration and Deployment for Flutter Apps: Best Practices
In the fast-paced world of mobile app development, staying ahead of the competition means not only creating feature-rich applications but also ensuring quick and reliable updates. This is where Continuous Integration and Deployment (CI/CD) comes into play. For Flutter developers, CI/CD can be a game-changer, allowing for seamless integration of changes and automatic deployment of new app versions. In this comprehensive guide, we’ll explore the best practices for implementing CI/CD in your Flutter app development process, along with code samples and actionable insights.
Table of Contents
1. What is Continuous Integration and Deployment (CI/CD)?
Continuous Integration and Deployment, commonly referred to as CI/CD, is a set of practices and tools that enable developers to automate the building, testing, and deployment of their applications. This approach helps streamline the development process, reduce manual errors, and accelerate the delivery of new features or bug fixes to end-users. CI/CD consists of two main phases:
1.1. Continuous Integration (CI)
Continuous Integration (CI) focuses on the automation of code integration and testing. Developers regularly push their code changes to a shared repository, triggering automated build and test processes. If any issues arise, they are identified and fixed early in the development cycle, reducing the chances of defects reaching the production environment.
1.2. Continuous Deployment (CD)
Continuous Deployment (CD) extends CI by automating the deployment process. After successfully passing all tests in the CI phase, the application is automatically deployed to the production environment. This ensures that the latest, thoroughly tested version of the app is always available to end-users.
2. Why CI/CD for Flutter Apps?
Implementing CI/CD for Flutter apps offers numerous advantages:
2.1. Faster Development Cycles
CI/CD streamlines the development process by automating repetitive tasks, such as building, testing, and deployment. This results in faster development cycles, allowing you to release new features and updates more frequently.
2.2. Improved Code Quality
Automated testing in the CI phase helps identify and fix bugs early in the development process. This leads to improved code quality and reduces the likelihood of issues in the production environment.
2.3. Consistency Across Environments
CI/CD ensures that your app behaves consistently across different environments, from development to production. This minimizes the “it works on my machine” problem and provides a more reliable user experience.
2.4. Rollback Capability
In case an issue arises after deployment, CI/CD allows for easy rollback to a previous version, mitigating the impact of unexpected problems on end-users.
Now that we understand the importance of CI/CD for Flutter apps, let’s delve into the best practices to implement it effectively.
3. Best Practices for CI/CD in Flutter Apps
3.1. Version Control with Git
Version control is the foundation of CI/CD. Use Git as your version control system to track changes, collaborate with team members, and maintain a history of your codebase. Popular platforms like GitHub, GitLab, and Bitbucket provide hosting for Git repositories.
Code Sample:
bash # Initialize a Git repository git init # Add your code to the repository git add . # Commit your changes git commit -m "Initial commit" # Create a remote repository on GitHub (replace 'username' and 'repo' with your details) git remote add origin https://github.com/username/repo.git # Push your code to the remote repository git push -u origin master
3.2. Automated Testing
Implement automated tests for your Flutter app to ensure code quality and catch regressions early. Utilize Flutter’s built-in testing framework or third-party packages like flutter_test and mockito for unit, widget, and integration testing.
Code Sample (Running Flutter Tests):
bash # Run unit tests flutter test # Run widget tests flutter test test/widget_test.dart # Run integration tests flutter drive --target=test_driver/app.dart
3.3. Continuous Integration (CI) Servers
Choose a CI server to automate your build and test processes. Some popular options for Flutter CI/CD pipelines include:
- Travis CI: A cloud-based CI service that integrates seamlessly with GitHub repositories.
- CircleCI: Offers scalable and customizable CI/CD pipelines for your Flutter projects.
- Jenkins: An open-source automation server that provides robust CI/CD capabilities.
Configuration Example (Travis CI):
yaml # .travis.yml language: dart dart: - stable script: - flutter test
3.4. Versioning and Dependency Management
Maintain consistent and reproducible builds by specifying Flutter and Dart versions in your project. Additionally, use a pubspec.yaml file to manage dependencies, ensuring that all team members work with the same library versions.
Code Sample (pubspec.yaml):
yaml dependencies: flutter: sdk: flutter http: ^2.0.0
3.5. Code Signing and Security
Secure your Flutter app by implementing code signing for iOS and Android. Store sensitive information, such as API keys and credentials, securely using environment variables or a secrets manager.
Code Sample (iOS Code Signing):
bash # Generate a signing certificate (replace 'MyCertificate' and 'MyKey' with your details) security create-keychain -p mypassword MyKeychain.keychain security import Certificate.p12 -k ~/Library/Keychains/MyKeychain.keychain -P mypassword security set-key-partition-list -S apple-tool:,apple: -s -k mypassword MyKeychain.keychain
3.6. Artifact Management
Store and manage build artifacts, such as APKs and IPA files, for future reference and distribution. Artifact repositories like JFrog Artifactory and Sonatype Nexus can help you achieve this.
3.7. Continuous Deployment (CD)
Implement CD by automating the deployment process of your Flutter app to various environments, including staging and production. Popular CD tools like Fastlane and Codemagic can simplify this task.
Example Fastlane Configuration (iOS Deployment):
ruby lane :beta do build_app upload_to_testflight end lane :release do build_app upload_to_app_store end
3.8. Monitoring and Alerts
Integrate monitoring tools like Firebase Crashlytics, Sentry, or New Relic to track app performance and user-reported issues. Set up alerts to proactively respond to any critical incidents.
3.9. Rollback Plan
Always have a rollback plan in place in case of unexpected issues after deployment. This might involve reverting to a previous version of the app or applying hotfixes.
3.10. Documentation and Collaboration
Document your CI/CD processes and collaborate with your team to ensure everyone understands the workflow. Use tools like Confluence or GitHub Wiki for documentation.
Conclusion
Continuous Integration and Deployment (CI/CD) is a crucial practice for Flutter app development, enabling faster development cycles, improved code quality, and consistent deployments. By following the best practices outlined in this guide, you can streamline your development process and deliver high-quality Flutter apps to your users with confidence. Embrace automation, leverage the right tools, and prioritize code quality, and you’ll be well on your way to mobile app development success. Happy coding!
Table of Contents
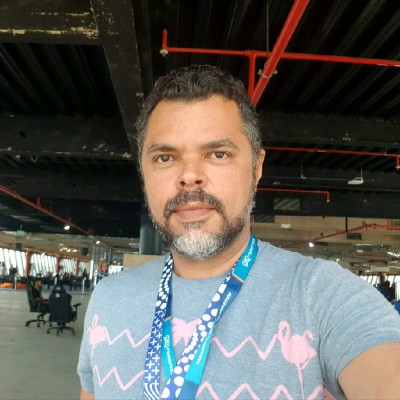
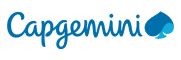