Navigating the Expedition of Hiring Flutter Developers: A Flutter Developer Interview Questions Guide
Flutter, the versatile UI toolkit, has emerged as a powerful solution for creating natively compiled applications across various platforms. To build stunning cross-platform apps, hiring skilled Flutter developers is essential. This guide acts as your compass through the hiring voyage, empowering you to evaluate candidates’ technical proficiency, problem-solving abilities, and Flutter expertise. Let’s set sail on this exploration, uncovering vital interview questions and strategies to identify the perfect Flutter developer for your team.
Table of Contents
1. How to Hire Flutter Developers
Embarking on the quest to hire Flutter developers? Follow these steps for a successful journey:
- Job Requirements: Define specific job prerequisites, outlining the skills and experience you’re seeking.
- Search Channels: Utilize job postings, online platforms, and tech communities to discover potential candidates.
- Screening: Scrutinize candidates’ Flutter proficiency, relevant experience, and additional skills.
- Technical Assessment: Develop a comprehensive technical assessment to evaluate coding abilities and problem-solving aptitude.
2. Core Skills of Flutter Developers to Look For
When evaluating Flutter developers, be on the lookout for these core skills:
- Flutter Proficiency: A strong understanding of Flutter’s architecture, widgets, and state management.
- Dart Language: Expertise in the Dart programming language, including its features and syntax.
- UI/UX Design: Familiarity with UI/UX design principles to create visually appealing and user-friendly interfaces.
- Cross-Platform Development: Ability to develop consistent and performant apps for multiple platforms, such as iOS and Android.
- API Integration: Experience integrating with APIs to fetch and display data within the app.
- State Management: Knowledge of various state management solutions like Provider or Bloc.
- Problem-Solving Abilities: Aptitude for identifying and resolving technical challenges efficiently.
3. Overview of the Flutter Developer Hiring Process
Here’s an overview of the Flutter developer hiring process:
3.1 Defining Job Requirements and Skillsets
Lay the foundation by outlining clear job prerequisites, specifying the skills and knowledge you’re seeking.
3.2 Crafting Compelling Job Descriptions
Create captivating job descriptions that accurately convey the role, attracting the right candidates.
3.3 Crafting Flutter Developer Interview Questions
Develop a comprehensive set of interview questions covering Flutter intricacies, problem-solving aptitude, and relevant technologies.
4. Sample Flutter Developer Interview Questions and Answers
Explore these sample questions with detailed answers to assess candidates’ Flutter skills:
Q1. Explain the concept of “widgets” in Flutter and differentiate between “StatelessWidget” and “StatefulWidget.”
A: Widgets are the building blocks of Flutter applications. StatelessWidget
is used for UI components that do not change based on user interaction, while StatefulWidget
is used for components that can change dynamically over time.
Q2. Build a simple Flutter app that displays “Hello, Flutter” on the screen.
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar(title: Text('Flutter App')), body: Center(child: Text('Hello, Flutter')), ), ); } }
Q3. Explain the concept of “hot reload” in Flutter and its benefits for development.
A: Hot reload allows developers to instantly see the effects of code changes without restarting the entire app. It speeds up development by preserving app state and avoiding time-consuming rebuilds.
Q4. Build a Flutter app that fetches data from a JSON API and displays it in a ListView.
import 'package:flutter/material.dart'; import 'dart:convert'; import 'package:http/http.dart' as http; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { Future<List<dynamic>> fetchData() async { final response = await http.get(Uri.parse('https://api.example.com/data')); if (response.statusCode == 200) { return json.decode(response.body); } else { throw Exception('Failed to load data'); } } @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar(title: Text('Flutter API App')), body: FutureBuilder<List<dynamic>>( future: fetchData(), builder: (context, snapshot) { if (snapshot.hasData) { return ListView.builder( itemCount: snapshot.data.length, itemBuilder: (context, index) { return ListTile(title: Text(snapshot.data[index]['title'])); }, ); } else if (snapshot.hasError) { return Text('Error loading data'); } return CircularProgressIndicator(); }, ), ), ); } }
Q5. Explain the purpose of the “async” and “await” keywords in Dart and how they facilitate asynchronous programming.
A: The async
keyword is used to mark a function as asynchronous, allowing the use of await
to pause execution until an asynchronous operation completes. This facilitates non-blocking code execution.
Q6. Build a Flutter app with a button that increments a counter when clicked.
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { int _counter = 0; void _incrementCounter() { setState(() { _counter++; }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Counter App')), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text('Counter:'), Text('$_counter', style: Theme.of(context).textTheme.headline4), ], ), ), floatingActionButton: FloatingActionButton( onPressed: _incrementCounter, tooltip: 'Increment', child: Icon(Icons.add), ), ); } }
Q7. Explain the concept of “routes” in Flutter navigation and demonstrate how to navigate between two screens.
A: Routes define the navigation paths within a Flutter app. To navigate between screens, use the Navigator
class and push a route onto the navigation stack.
Q8. Build a Flutter app that includes user authentication using Firebase Authentication.
// Implementing Firebase authentication requires a comprehensive code sample. Here's a high-level overview. import 'package:flutter/material.dart'; import 'package:firebase_auth/firebase_auth.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: AuthenticationWrapper(), ); } } class AuthenticationWrapper extends StatelessWidget { final FirebaseAuth _auth = FirebaseAuth.instance; @override Widget build(BuildContext context) { if (_auth.currentUser != null) { return HomeScreen(); } else { return LoginScreen(); } } } class HomeScreen extends StatelessWidget { final FirebaseAuth _auth = FirebaseAuth.instance; void _signOut() async { await _auth.signOut(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Home')), body: Center(child: Text('Welcome!')), floatingActionButton: FloatingActionButton( onPressed: _signOut, child: Icon(Icons.logout), ), ); } } class LoginScreen extends StatelessWidget { // Implement Firebase login using FirebaseAuth }
Q9. Explain the concept of “State Management” in Flutter and discuss different state management approaches.
A: State management is the process of handling and sharing data across different parts of an app. Approaches like Provider, Bloc, and MobX offer solutions to manage app state effectively.
Q10. What are “Flutter Widgets”? Differentiate between “StatelessWidget” and “StatefulWidget.”
A: Widgets are building blocks of the Flutter UI. A StatelessWidget
is immutable and doesn’t store dynamic data, while a StatefulWidget
can change over time, preserving its state.
5. Hiring Flutter Developers through CloudDevs
Step 1: Connect with CloudDevs: Initiate a conversation with a CloudDevs consultant to discuss your project’s requirements, preferred skills, and expected experience.
Step 2: Find Your Ideal Match: Within 24 hours, CloudDevs presents you with carefully selected Flutter developers from their pool of pre-vetted professionals. Review their profiles and select the candidate who aligns with your project’s vision.
Step 3: Embark on a Risk-Free Trial: Engage in discussions with your chosen developer to ensure a smooth onboarding process. Once satisfied, formalize the collaboration and commence a week-long free trial.
By leveraging the expertise of CloudDevs, you can effortlessly identify and hire exceptional Flutter developers, ensuring your team possesses the skills required to build remarkable cross-platform applications.
6. Conclusion
With these additional technical questions and insights at your disposal, you’re now well-prepared to assess Flutter developers comprehensively. Whether you’re creating captivating UIs or developing cross-platform apps, securing the right Flutter developers for your team is a pivotal step toward app excellence.
Table of Contents
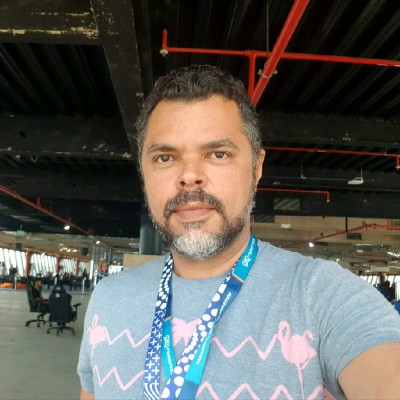
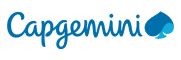