Flutter for IoT: Creating Smart Apps for Connected Devices
In a world driven by connectivity and automation, the Internet of Things (IoT) has taken center stage. IoT devices are seamlessly integrating into our lives, from smart thermostats and wearable fitness trackers to connected cars and home security systems. As these devices become more prevalent, the demand for intuitive and user-friendly applications to control them is on the rise. This is where Flutter, Google’s open-source UI software development toolkit, comes into play. Flutter’s versatility and rapid development capabilities make it an ideal choice for crafting smart apps that interact with a diverse range of IoT devices. In this article, we’ll delve into the exciting realm of Flutter for IoT and explore how to create intelligent applications for connected devices.
1. Understanding the Power of Flutter for IoT
Flutter has gained immense popularity among developers for its ability to create cross-platform applications with a single codebase. Whether you’re targeting Android, iOS, web, or even desktop platforms, Flutter ensures a consistent and visually appealing experience across all devices. This is a crucial advantage when it comes to IoT applications, as users expect seamless interaction regardless of the device they’re using.
2. Benefits of Using Flutter for IoT Applications
Rapid Development: Flutter’s hot reload feature enables developers to instantly see changes in the app’s UI as they code. This real-time feedback greatly speeds up the development process, allowing for quicker iterations and bug fixes.
dart // Example of using hot reload in Flutter void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar(title: Text('Flutter IoT App')), body: Center(child: Text('Hello, IoT World!')), ), ); } }
Beautiful UIs: Flutter’s rich set of customizable widgets makes it easy to design stunning user interfaces that match the branding and aesthetics of your IoT devices.
Cross-Platform Compatibility: With a single codebase, you can deploy your IoT app on multiple platforms, reducing development time and maintenance efforts.
Native Performance: Flutter’s architecture allows it to compile to native ARM code, ensuring excellent performance on resource-constrained IoT devices.
3. Building an IoT App with Flutter
Let’s walk through the process of building a simple IoT app using Flutter. In this example, we’ll create a basic interface to control the lighting of a fictional smart home.
3.1 Setting Up the Project
Before getting started, ensure you have Flutter installed. You can check by running flutter doctor in your terminal. If not installed, follow the official installation guide on the Flutter website.
Create a New Flutter Project:
bash flutter create SmartHomeApp cd SmartHomeApp
Add Dependencies:
Open the pubspec.yaml file in your project directory and add the following dependency for HTTP requests:
yaml dependencies: flutter: sdk: flutter http: ^0.13.3
Save the file and run flutter pub get to fetch the new dependency.
3.2 Designing the User Interface
In this step, we’ll design a simple UI for our smart home app. We’ll use Flutter’s widgets to create a sleek and intuitive interface.
Replace the Contents of lib/main.dart with the Following Code:
dart import 'package:flutter/material.dart'; import 'package:http/http.dart' as http; void main() { runApp(SmartHomeApp()); } class SmartHomeApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Smart Home App', theme: ThemeData(primarySwatch: Colors.blue), home: SmartHomeScreen(), ); } } class SmartHomeScreen extends StatefulWidget { @override _SmartHomeScreenState createState() => _SmartHomeScreenState(); } class _SmartHomeScreenState extends State<SmartHomeScreen> { bool _isLightOn = false; void _toggleLight() async { final response = await http.post( Uri.parse('https://api.example.com/control_light'), body: {'action': _isLightOn ? 'turn_off' : 'turn_on'}, ); if (response.statusCode == 200) { setState(() { _isLightOn = !_isLightOn; }); } } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Smart Home')), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Icon( _isLightOn ? Icons.lightbulb : Icons.lightbulb_outline, size: 100, color: _isLightOn ? Colors.yellow : Colors.grey, ), SizedBox(height: 20), ElevatedButton( onPressed: _toggleLight, child: Text(_isLightOn ? 'Turn Off Light' : 'Turn On Light'), ), ], ), ), ); } }
In this example code, we’ve created a simple app with a toggle button to control the light. The app uses the http package to make HTTP requests to a fictional API (https://api.example.com/control_light). Depending on the response, the light’s status is updated.
4. Connecting Flutter with IoT Devices
Now that we have a basic app, let’s explore how to connect it with real IoT devices. The process may vary depending on the specific device and communication protocol you’re using. For this example, we’ll assume our smart home’s lighting system provides a RESTful API for control.
Replace the URL in _toggleLight with Your Device’s API URL:
dart final response = await http.post( Uri.parse('https://your-device-api-url/control_light'), body: {'action': _isLightOn ? 'turn_off' : 'turn_on'}, );
Ensure Your Device’s API is Configured:
Consult your device’s documentation to understand its API endpoints and required parameters. Make sure your device is accessible from the network your Flutter app is running on.
5. Security Considerations
When developing IoT applications, security is of paramount importance. Ensure that communication between your Flutter app and IoT devices is encrypted and authenticated to prevent unauthorized access.
- Use HTTPS: Always communicate with your IoT devices over HTTPS to encrypt data transmitted between the app and the device.
- Authentication: Implement secure authentication mechanisms to prevent unauthorized control of IoT devices.
- Authorization: Set up role-based access control to define who can perform actions on IoT devices.
Conclusion
Flutter’s capabilities extend beyond mobile and web app development—it’s a powerful tool for creating smart applications that control IoT devices seamlessly. Its cross-platform compatibility, rapid development cycle, and beautiful UI capabilities make it an excellent choice for building user-friendly IoT experiences. As you venture into the world of IoT app development, remember to prioritize security and maintainability to ensure your applications provide reliable and secure interactions with connected devices. So go ahead, start experimenting with Flutter for IoT, and unlock a realm of possibilities in the world of connected technology.
In this article, we’ve only scratched the surface of what’s possible with Flutter for IoT. By exploring more advanced features, integrating with different IoT protocols, and diving into real-world use cases, you can build truly remarkable applications that redefine the way users interact with their smart devices. So, what are you waiting for? Start building your own Flutter-powered IoT apps and be part of the revolution in connected experiences.
Table of Contents
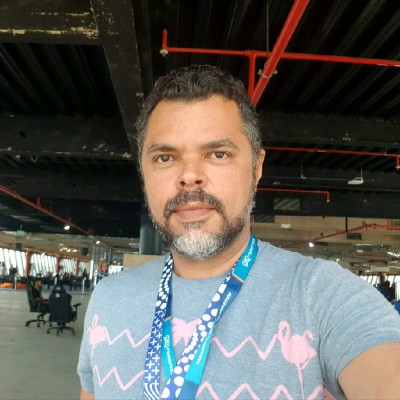
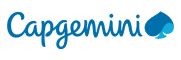