What is the purpose of the ListView widget in Flutter?
The `ListView` widget in Flutter serves as a fundamental building block for creating scrollable lists of widgets. Its primary purpose is to display a scrolling, linear list of children. This widget is crucial for handling scenarios where you need to present a collection of items that may extend beyond the device’s screen, allowing users to scroll through the content seamlessly.
Scrollable Lists: `ListView` provides a scrollable viewport for a list of widgets, ensuring efficient memory usage by loading and unloading items dynamically as the user scrolls.
Versatility: It supports a variety of children, allowing developers to include a mix of different widgets such as text, images, or even custom widgets within the list.
Builder Pattern: To optimize performance, Flutter uses the builder pattern with `ListView.builder()`. This constructs items on-demand as they become visible during scrolling, preventing unnecessary widget creation and improving efficiency.
Infinite Scrolling: With `ListView.builder()`, you can easily implement infinite scrolling by dynamically loading additional items as the user reaches the end of the current list.
Example:
```dart ListView.builder( itemCount: myData.length, itemBuilder: (context, index) { return ListTile( title: Text(myData[index]['title']), subtitle: Text(myData[index]['subtitle']), leading: CircleAvatar( backgroundImage: NetworkImage(myData[index]['avatarUrl']), ), onTap: () { // Handle item tap }, ); }, ) ```
In this example, `ListView.builder()` efficiently builds a list of `ListTile` widgets based on the data in the `myData` list. The builder pattern ensures that only the items visible on the screen are created, optimizing performance for long lists.
The `ListView` widget in Flutter is a fundamental tool for creating scrollable lists, offering versatility and efficient memory usage, especially when dealing with large datasets or dynamic content.
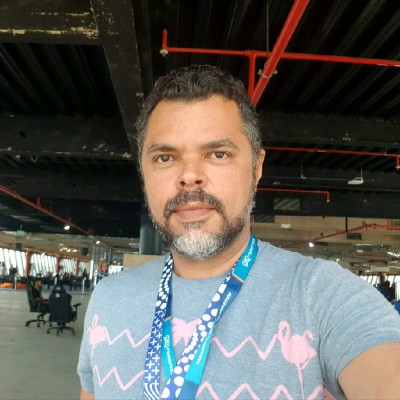
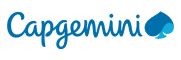