Flutter for Machine Learning: Integrating TensorFlow in Apps
In the ever-evolving landscape of mobile app development, one trend stands out prominently: the fusion of machine learning with app functionalities. This convergence opens up exciting possibilities, from real-time image recognition to natural language processing. Flutter, Google’s open-source UI software development toolkit, offers a powerful platform for crafting visually appealing cross-platform apps. When combined with TensorFlow, an open-source machine learning framework, Flutter becomes a force to be reckoned with in the realm of mobile machine learning.
1. Why Integrate Machine Learning?
Machine learning has transformed the way we interact with technology. It’s no longer just about following programmed instructions; applications can now learn and adapt from data, offering personalized experiences that cater to individual user preferences. Integrating machine learning in your Flutter app can enable:
1.1. Enhanced User Experiences:
Imagine an app that can identify objects in real-time through the smartphone camera or provide recommendations based on user behavior. These features not only make the app more engaging but also add a touch of personalization that users love.
1.2. Efficient Data Processing:
Machine learning can handle vast amounts of data and extract meaningful insights from it. Whether it’s analyzing user behavior or predicting trends, these capabilities can significantly enhance the app’s efficiency.
1.3. Automation and Assistance:
Chatbots, virtual assistants, and predictive text are all powered by machine learning. By integrating these features, you can create apps that proactively assist users, making their interactions smoother and more efficient.
2. TensorFlow: Powering the Machine Learning
At the heart of many machine learning endeavors lies TensorFlow, an open-source framework developed by the Google Brain team. TensorFlow provides a comprehensive suite of tools and libraries for building and deploying machine learning models across different platforms and devices. Its versatility and robustness have made it a staple in the AI and machine learning community.
2.1. Integrating TensorFlow with Flutter
Integrating TensorFlow into your Flutter app might sound like a complex endeavor, but the combination of these two technologies can yield remarkable results. Here’s a step-by-step guide to help you get started:
Step 1: Set Up Your Flutter Project
If you haven’t already, install Flutter and set up a new project:
bash flutter create your_ml_app cd your_ml_app
Step 2: Add TensorFlow Dependency
In your pubspec.yaml file, add the TensorFlow package to your dependencies:
yaml dependencies: flutter: sdk: flutter tensorflow: ^2.5.0 # Use the latest version
Then, run flutter packages get to fetch the new dependency.
Step 3: Design Your App Interface
Design the UI of your app as you would for any other Flutter app. TensorFlow will mainly come into play when you start integrating machine learning features.
Step 4: Load Your Pretrained Model
TensorFlow allows you to train and export machine learning models in various formats. For this example, let’s assume you have a pretrained image classification model in the TensorFlow SavedModel format (.pb file). Place the model file inside a directory in your Flutter project.
Step 5: Install the path_provider Package
You’ll need the path_provider package to access the path to your model file. Add it to your pubspec.yaml:
yaml dependencies: path_provider: ^2.0.5
As always, run flutter packages get.
Step 6: Load the Model in Your Flutter Code
Import the necessary packages at the beginning of your Dart file:
dart import 'package:flutter/material.dart'; import 'package:path_provider/path_provider.dart'; import 'package:tensorflow/tensorflow.dart' as tf;
Now, load the model in your widget’s code:
dart class MLPage extends StatefulWidget { @override _MLPageState createState() => _MLPageState(); } class _MLPageState extends State<MLPage> { tf.Graph model; @override void initState() { super.initState(); loadModel(); } Future<void> loadModel() async { Directory appDir = await getApplicationDocumentsDirectory(); String modelPath = '${appDir.path}/your_model_directory/your_model.pb'; model = await tf.Graph.fromSavedModel(modelPath); setState(() {}); } @override Widget build(BuildContext context) { // Build your UI here and utilize the loaded model } }
Replace your_model_directory and your_model.pb with the appropriate paths and filenames.
Step 7: Make Predictions
With your model loaded, you can now make predictions. For example, if you have an image classification model, you can use it to classify images:
dart var input = <String, tf.Tensor>{ 'input': tf.Tensor.from(<List>/* Your input data */), }; var outputs = model.run(input, <String>['output']); var predictions = outputs['output']; // Process the predictions as needed
Remember to replace ‘input’ and ‘output’ with the actual names of your input and output nodes in the model.
3. Potential Applications
The integration of TensorFlow with Flutter opens up a plethora of applications:
3.1. Image and Video Analysis:
Build apps that can recognize objects, scenes, or even emotions from images and videos captured by the device’s camera.
3.2. Natural Language Processing (NLP):
Integrate language models to create applications that can understand and generate human-like text. This could be used for chatbots, sentiment analysis, and more.
3.3. Anomaly Detection:
Develop apps that can detect anomalies in various data streams, such as identifying unusual patterns in user behavior for fraud detection.
3.4. Gesture Recognition:
Create apps that can understand and respond to gestures, opening up opportunities for interactive and immersive user experiences.
Conclusion
The integration of TensorFlow with Flutter presents an exciting avenue for developers to create intelligent and engaging mobile applications. By leveraging TensorFlow’s powerful machine learning capabilities within the Flutter framework, you can build applications that learn, adapt, and offer unprecedented user experiences. Whether you’re a seasoned developer or just starting, exploring this fusion of technologies can propel your app development skills to new heights. So why wait? Dive into the world of TensorFlow and Flutter, and unlock a realm of possibilities for your next mobile app project.
In a nutshell, combining TensorFlow’s machine learning prowess with Flutter’s versatile app development framework can lead to groundbreaking applications that understand, learn, and evolve alongside their users. The journey might have its challenges, but the destination is well worth the effort.
With this guide, you’re now equipped to embark on your journey of integrating TensorFlow with Flutter. Remember, the possibilities are virtually limitless, and the skills you acquire will position you at the forefront of innovation in the mobile app development landscape. Happy coding!
Table of Contents
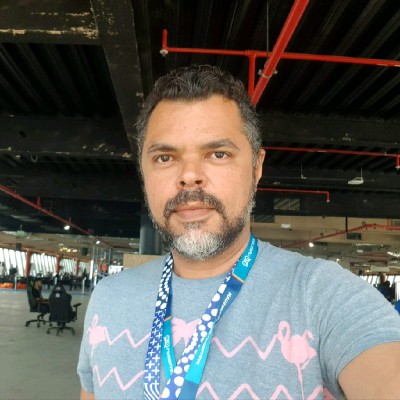
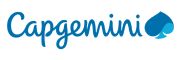