Exploring Flutter’s Material Design: Styling Your App
In the world of app development, aesthetics play a crucial role in capturing users’ attention and providing them with a delightful experience. Google’s Material Design is a design language that offers a comprehensive set of guidelines and components for creating visually appealing and consistent user interfaces. Flutter, Google’s UI toolkit, makes it exceptionally easy to implement Material Design principles in your app.
Table of Contents
In this blog post, we will explore the key aspects of styling your Flutter app using Material Design. We’ll cover essential topics, provide code samples, and share best practices to help you create a beautiful and user-friendly application.
1. Understanding Material Design
1.1. What is Material Design?
Material Design is a design language developed by Google that aims to provide a consistent and visually appealing user experience across different platforms and devices. It is characterized by clean and responsive layouts, bold colors, smooth animations, and intuitive navigation. Material Design principles focus on creating realistic digital interfaces that mimic the physical world, with elements like cards, shadows, and meaningful motion.
1.2. Why Use Material Design in Flutter?
Integrating Material Design into your Flutter app offers several advantages:
- Consistency: Material Design provides a set of guidelines and components that ensure a consistent look and feel across your app, regardless of the platform it runs on.
- Familiarity: Users are already accustomed to Material Design, as many popular apps, including Google’s own, use this design language. This familiarity can enhance user comfort and usability.
- Efficiency: Flutter’s extensive library of Material Design widgets and tools streamlines the development process, saving you time and effort.
Now that you understand the importance of Material Design in Flutter, let’s proceed to set up your development environment.
2. Setting Up Your Flutter Environment
2.1. Install Flutter and Dart
Before you can start building your Material Design app with Flutter, you need to install the Flutter SDK and Dart programming language. Follow these steps:
Visit the official Flutter website to find installation instructions for your operating system.
Download and install Flutter by following the provided instructions.
After installation, open a terminal window and run the following command to verify your installation:
bash flutter --version
Install Dart by running:
bash flutter pub global activate dart
2.2. Create a New Flutter Project
Now that you have Flutter and Dart installed, it’s time to create a new Flutter project:
Open a terminal window.
Navigate to your desired project directory:
bash cd path/to/your/project/directory
Create a new Flutter project using the following command:
bash flutter create my_material_app
Change into the project directory:
bash cd my_material_app
With your Flutter project set up, let’s move on to applying Material Design styles.
3. Applying Material Design Styles
3.1. Material Colors and Typography
Material Design provides a predefined color palette and typography guidelines to maintain a consistent visual identity. To use these styles, open the lib/main.dart file in your Flutter project and update the MaterialApp widget:
dart import 'package:flutter/material.dart'; void main() { runApp(MaterialApp( title: 'My Material App', theme: ThemeData( primarySwatch: Colors.blue, // Change the primary color fontFamily: 'Roboto', // Set a custom font ), home: MyHomePage(), )); }
Here, we set the primary color to blue and specify the ‘Roboto’ font for the entire app. You can adjust these values to match your app’s branding.
3.2. Buttons and Icons
Material Design offers a wide range of buttons and icons that you can easily integrate into your Flutter app. For example, to create a raised button, use the ElevatedButton widget:
dart ElevatedButton( onPressed: () { // Handle button press }, child: Text('Click Me'), )
To add an icon, use the Icon widget:
dart Icon( Icons.star, color: Colors.yellow, )
You can explore the full list of available icons in the Material Icons library.
3.3. Cards and Surfaces
Material Design emphasizes the use of cards and surfaces to organize content and provide a sense of depth. To create a card, use the Card widget:
dart Card( child: ListTile( leading: Icon(Icons.android), title: Text('Material Card'), subtitle: Text('This is a sample card.'), ), )
This code creates a simple card with an icon, title, and subtitle. You can customize the card’s appearance further to match your app’s style.
3.4. Navigation and App Bars
Navigation is a fundamental aspect of any app, and Material Design offers efficient solutions for it. To implement a basic app bar, use the AppBar widget:
dart AppBar( title: Text('My App'), actions: [ IconButton( icon: Icon(Icons.search), onPressed: () { // Handle search action }, ), ], )
This code creates an app bar with a title and a search icon in the top-right corner. You can add more customization options and navigation features as needed.
4. Creating a Material Design UI
4.1. Scaffold and AppBar
The Scaffold widget is a key component for creating Material Design layouts. It provides a basic structure for your app, including an app bar, body, and optional floating action button (FAB). Here’s an example of how to use Scaffold:
dart Scaffold( appBar: AppBar( title: Text('My App'), ), body: Center( child: Text('Welcome to my Material Design app!'), ), )
In this code, we define an app bar and set the body content to a centered text widget. You can further enhance your app’s structure by adding navigation elements, tabs, and drawers.
4.2. Bottom Navigation
Material Design encourages the use of a bottom navigation bar for easy access to different sections of your app. To implement it, use the BottomNavigationBar widget:
dart BottomNavigationBar( items: [ BottomNavigationBarItem( icon: Icon(Icons.home), label: 'Home', ), BottomNavigationBarItem( icon: Icon(Icons.notifications), label: 'Notifications', ), BottomNavigationBarItem( icon: Icon(Icons.person), label: 'Profile', ), ], currentIndex: _selectedIndex, onTap: _onItemTapped, )
This code defines a bottom navigation bar with three items: Home, Notifications, and Profile. You can handle item taps by specifying the _onItemTapped function.
4.3. Tabs and TabBar
Tabs are a great way to organize content in your app. Material Design provides the TabBar widget for this purpose:
dart TabBar( tabs: [ Tab(text: 'Tab 1'), Tab(text: 'Tab 2'), Tab(text: 'Tab 3'), ], controller: _tabController, )
To use tabs, you also need to set up a TabController and associate it with your TabBar.
4.4. Drawer Navigation
Drawer navigation allows users to access additional app functionality and settings. To implement a drawer, use the Drawer widget:
dart Drawer( child: ListView( padding: EdgeInsets.zero, children: <Widget>[ UserAccountsDrawerHeader( accountName: Text('John Doe'), accountEmail: Text('john@example.com'), currentAccountPicture: CircleAvatar( backgroundImage: AssetImage('assets/avatar.png'), ), ), ListTile( leading: Icon(Icons.settings), title: Text('Settings'), onTap: () { // Handle settings navigation }, ), ListTile( leading: Icon(Icons.exit_to_app), title: Text('Log Out'), onTap: () { // Handle log out }, ), ], ), )
This code creates a simple drawer with a user account header, settings option, and a log out button. You can customize the content and actions according to your app’s requirements.
5. Customizing Material Design
5.1. Theming Your App
Material Design allows you to customize your app’s visual style by creating and applying themes. Themes define colors, typography, and other visual properties. To create a custom theme, define a ThemeData object and set it in your MaterialApp:
dart final ThemeData myTheme = ThemeData( primarySwatch: Colors.indigo, accentColor: Colors.pink, fontFamily: 'Roboto', ); void main() { runApp(MaterialApp( title: 'My Material App', theme: myTheme, home: MyHomePage(), )); }
In this example, we define a custom theme with an indigo primary color and pink as the accent color. You can create more extensive themes by specifying text styles, button styles, and other visual elements.
5.2. Creating Custom Themes
If you need to create multiple custom themes for your app, you can use Theme widgets at a widget level. Wrap the parts of your app that should use a specific theme in a Theme widget:
dart Theme( data: ThemeData(primarySwatch: Colors.green), child: MyAppContent(), )
This approach allows you to apply different themes to different parts of your app.
5.3. Adding Splash Screens
A splash screen is the initial screen that appears when your app is launched. You can customize it to match your app’s Material Design style. To create a splash screen, follow these steps:
- Create a new Dart file for your splash screen, e.g., splash_screen.dart.
- Design your splash screen using Material Design elements, such as a logo and background color.
- In your app’s main entry point (usually main.dart), set the splashScreen property in the MaterialApp widget:
dart MaterialApp( title: 'My Material App', theme: myTheme, home: SplashScreen(), // ... )
This code directs the app to start with your custom splash screen.
6. Testing Your Material Design App
Testing is a crucial part of app development, ensuring that your app works correctly and looks as intended. Flutter provides various testing tools and techniques for your Material Design app:
6.1. Widgets Testing
Widgets testing allows you to test individual widgets in isolation. You can use the flutter_test package to write unit tests for your widgets. For example, you can test the behavior of a button widget when it’s pressed.
6.2. UI Testing
UI testing, also known as integration testing, tests the interactions between widgets and screens. Flutter’s flutter_driver package lets you write UI tests to verify that your Material Design app’s UI components work together correctly.
6.3. Device-Specific Testing
To ensure your Material Design app functions properly on different devices and screen sizes, perform device-specific testing. Use Flutter’s built-in device emulators and test on real devices to catch issues related to responsiveness and layout.
Conclusion
In this blog post, we’ve explored the world of Material Design and how to implement it in your Flutter app. We covered everything from setting up your development environment to creating custom themes and testing your app. By following these guidelines and best practices, you can create a visually stunning and user-friendly app that adheres to Google’s Material Design principles.
Material Design not only enhances the aesthetic appeal of your app but also contributes to a consistent and intuitive user experience. So, go ahead, dive into Flutter, and start styling your app with Material Design today!
Table of Contents
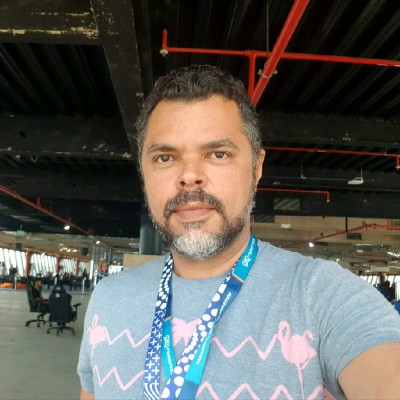
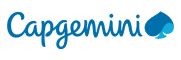