Flutter Q & A
Can you use native modules in a Flutter project?
In Flutter development, the ability to integrate native modules is crucial for accessing platform-specific functionalities. While Flutter provides a rich set of widgets and plugins for cross-platform development, there are instances where developers need to leverage existing native code written in languages like Java or Kotlin for Android, and Objective-C or Swift for iOS.
- Platform Channels: Flutter’s platform channels facilitate communication between Dart code and native code. Developers can create and use platform channels to invoke native methods from Dart and vice versa.
- Method Channels: Method channels enable the invocation of specific methods in native code from Dart, passing data between the Flutter and native layers seamlessly.
- Example Scenario: Suppose a Flutter app needs to access a device’s camera functionalities. Instead of implementing the camera-related features entirely in Dart, developers can use platform channels to invoke native camera APIs. This allows for a more efficient utilization of existing, well-optimized code.
- Implementation: In the Flutter project, developers define a platform channel and create method channels for specific functionalities. In native code, they implement corresponding methods that can be called from Flutter.
Example Code:
Flutter Dart Code: ```dart import 'package:flutter/services.dart'; const platform = MethodChannel('com.example.camera'); Future<void> captureImage() async { try { await platform.invokeMethod('captureImage'); } on PlatformException catch (e) { print("Failed to invoke native method: ${e.message}"); } } ``` Native Android Code (Java/Kotlin): ```java // Inside MainActivity.java or MainActivity.kt public class MainActivity extends FlutterActivity { private static final String CHANNEL = "com.example.camera"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); new MethodChannel(getFlutterEngine().getDartExecutor().getBinaryMessenger(), CHANNEL) .setMethodCallHandler( (call, result) -> { if (call.method.equals("captureImage")) { // Implement native camera functionality captureImage(); } else { result.notImplemented(); } } ); } private void captureImage() { // Native camera implementation } } ```
By utilizing native modules through platform channels in Flutter, developers can seamlessly integrate platform-specific features, ensuring a harmonious blend of cross-platform development and native capabilities.
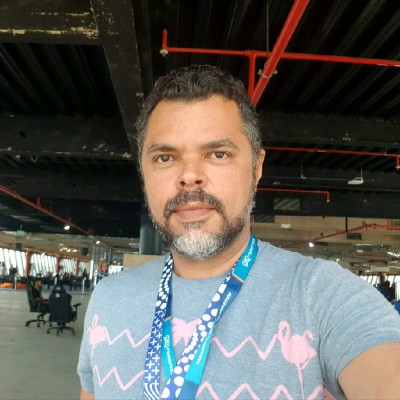
Previously at
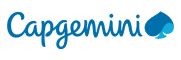
Full Stack Systems Analyst with a strong focus on Flutter development. Over 5 years of expertise in Flutter, creating mobile applications with a user-centric approach.