How does Flutter handle navigation between screens?
Flutter handles navigation between screens using a robust and flexible navigation system, primarily based on a widget called `Navigator`. The `Navigator` manages a stack of routes, where each route corresponds to a screen or page in the app. Developers can push and pop routes onto and off the stack to navigate between screens seamlessly.
To navigate to a new screen, developers typically use the `Navigator.push()` method, providing a new route to be added to the stack. Here’s a simple example:
```dart // Inside a widget's onPressed or onTap event handler onPressed: () { Navigator.push( context, MaterialPageRoute(builder: (context) => SecondScreen()), ); } ```
In this example, when the button is pressed, the `Navigator.push()` method is called with a new `MaterialPageRoute`. This MaterialPageRoute specifies the widget (`SecondScreen()`) to be displayed as the next route. The `context` parameter provides the necessary context for navigation.
To go back to the previous screen, developers use the `Navigator.pop()` method:
```dart // Inside the SecondScreen widget, for example, in a button's onPressed event handler onPressed: () { Navigator.pop(context); } ```
This pops the current route off the stack, returning to the previous screen.
Key points:
- Navigator Stack: Represents the navigation history of the app.
- Push and Pop: `Navigator.push()` adds a new route, while `Navigator.pop()` removes the current route.
- PageRoute: Often used to define transitions and animations between screens.
- Context: Necessary for navigation operations, providing information about the widget tree.
Flutter’s navigation system is intuitive and versatile, allowing developers to manage the flow between screens effortlessly.
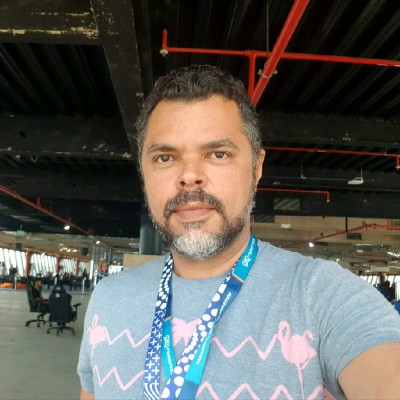
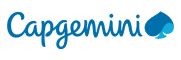