Exploring Flutter’s Neural Networks: Image Recognition and AI
Neural networks are a subset of machine learning designed to recognize patterns and make predictions based on data. In image recognition, neural networks are trained to identify and categorize objects within images, enabling applications to interpret visual information accurately. Integrating neural networks into mobile apps can significantly enhance their functionality, offering features such as real-time image classification and object detection.
Using Flutter for Neural Networks and Image Recognition
Flutter, Google’s UI toolkit for building natively compiled applications, can be effectively utilized to incorporate neural networks for image recognition tasks. With Flutter’s rich ecosystem of packages and support for integrating with TensorFlow Lite and other machine learning libraries, developers can build powerful AI-driven mobile applications. Below are some key aspects and code examples demonstrating how Flutter can be employed for neural networks and image recognition.
1. Integrating TensorFlow Lite with Flutter
TensorFlow Lite (TFLite) is a lightweight version of TensorFlow designed for mobile and embedded devices. Flutter supports TFLite through the `tflite_flutter` package, enabling developers to use pre-trained models for image classification and other AI tasks.
Example: Using TensorFlow Lite for Image Classification
Assume you have a pre-trained TFLite model for image classification. You can use the `tflite_flutter` package to load and utilize this model in your Flutter app.
```dart import 'package:flutter/material.dart'; import 'package:tflite_flutter/tflite_flutter.dart'; class ImageClassifier extends StatefulWidget { @override _ImageClassifierState createState() => _ImageClassifierState(); } class _ImageClassifierState extends State<ImageClassifier> { late Interpreter _interpreter; @override void initState() { super.initState(); _loadModel(); } Future<void> _loadModel() async { _interpreter = await Interpreter.fromAsset('model.tflite'); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Image Classifier')), body: Center( child: Text('Model Loaded Successfully'), ), ); } } ```
2. Processing and Preprocessing Images
Before feeding images into a neural network, they need to be preprocessed into the correct format. Flutter provides various packages to handle image processing tasks, including resizing and normalization.
Example: Preprocessing an Image for Classification
Here’s how you might preprocess an image using the `image` package before passing it to your TFLite model.
```dart import 'dart:io'; import 'package:image/image.dart' as img; Future<img.Image> preprocessImage(File imageFile) async { img.Image image = img.decodeImage(imageFile.readAsBytesSync())!; img.Image resizedImage = img.copyResize(image, width: 224, height: 224); return resizedImage; } ```
3. Real-Time Object Detection
For real-time object detection, you can integrate models that support bounding boxes and class predictions. Flutter’s `camera` package can be used to capture images from the camera feed and process them with your neural network model.
Example: Real-Time Object Detection
Here’s a simplified example of how you might set up real-time object detection using the camera feed.
```dart import 'package:flutter/material.dart'; import 'package:camera/camera.dart'; import 'package:tflite_flutter/tflite_flutter.dart'; class ObjectDetection extends StatefulWidget { @override _ObjectDetectionState createState() => _ObjectDetectionState(); } class _ObjectDetectionState extends State<ObjectDetection> { late CameraController _cameraController; late Interpreter _interpreter; @override void initState() { super.initState(); _initializeCamera(); _loadModel(); } Future<void> _initializeCamera() async { final cameras = await availableCameras(); _cameraController = CameraController(cameras[0], ResolutionPreset.high); await _cameraController.initialize(); } Future<void> _loadModel() async { _interpreter = await Interpreter.fromAsset('object_detection_model.tflite'); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Object Detection')), body: CameraPreview(_cameraController), ); } } ```
4. Visualizing Image Recognition Results
Visualizing the results of image recognition can help in understanding and interpreting the output of neural networks. Flutter supports various visualization libraries to help display results effectively.
Example: Displaying Classification Results
Here’s how you might display the classification results on the screen.
```dart import 'package:flutter/material.dart'; class ClassificationResult extends StatelessWidget { final String label; final double confidence; ClassificationResult({required this.label, required this.confidence}); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Classification Result')), body: Center( child: Text( 'Label: $label\nConfidence: ${confidence.toStringAsFixed(2)}', style: TextStyle(fontSize: 20), ), ), ); } } ```
Conclusion
Flutter provides a robust framework for integrating neural networks and performing image recognition tasks in mobile applications. By leveraging packages like `tflite_flutter` and tools for image preprocessing, developers can build advanced AI-driven features into their apps. Whether for real-time object detection or image classification, Flutter’s capabilities and ecosystem make it an excellent choice for developing intelligent mobile applications.
Further Reading:
Table of Contents
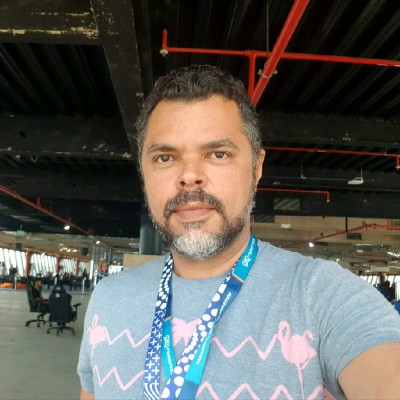
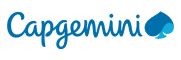