How does Flutter handle platform-specific code?
In Flutter, handling platform-specific code is crucial for achieving true cross-platform development while still allowing customization for each platform. Flutter uses a platform channel mechanism to communicate between Dart, the language in which Flutter apps are written, and native code written in languages like Java (for Android) or Swift/Objective-C (for iOS).
The platform channel is a bridge that enables communication between the Dart code and native code. It allows developers to encapsulate platform-specific functionality in separate files, known as method channels, which act as intermediaries. For example, if you need to access a device’s camera, you might have a method channel for camera-related operations.
Example:
```dart // Dart code import 'package:flutter/services.dart'; class CameraService { static const MethodChannel _channel = MethodChannel('camera_channel'); static Future<void> takePicture() async { try { await _channel.invokeMethod('takePicture'); } on PlatformException catch (e) { print("Error: ${e.message}"); } } } ```
On the native side, you would implement the ‘camera_channel’ method channel in Java or Swift, providing the necessary functionality for taking a picture. This way, you encapsulate the platform-specific code in native files, ensuring that your Flutter app interacts seamlessly with the underlying operating system.
Key points to remember include the necessity of creating method channels for each platform-specific task, maintaining a consistent communication protocol between Dart and native code, and encapsulating platform-specific logic to ensure code readability and maintainability. This approach empowers Flutter developers to harness the strengths of each platform while building a unified user experience across diverse devices.
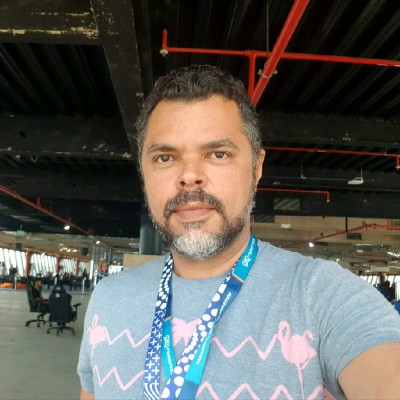
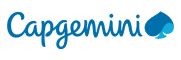