Implementing Push Notifications in Flutter: Engaging Users
Push notifications are a powerful tool for engaging users in your Flutter app. They allow you to send timely and relevant information to users, keeping them informed and engaged with your app. Whether it’s a news update, a special offer, or a reminder, push notifications can help you re-engage users and bring them back to your app.
Table of Contents
In this blog post, we will explore how to implement push notifications in your Flutter app and use them effectively to engage your users. We will cover the following topics:
1. Why Push Notifications Matter
Push notifications are an essential part of any modern mobile app strategy. Here’s why they matter:
1.1. User Engagement
Push notifications can significantly boost user engagement. They allow you to reach out to users even when your app is not open, reminding them about your app’s value and encouraging them to return.
1.2. Personalization
Push notifications can be personalized based on user behavior, preferences, and location. Personalized messages are more likely to resonate with users, leading to higher engagement rates.
1.3. Retention
Sending relevant push notifications can help improve user retention. By reminding users of your app’s existence and value, you can reduce churn and keep users coming back.
Now that we understand the importance of push notifications, let’s dive into how to implement them in your Flutter app.
2. Setting Up Push Notifications
To implement push notifications in your Flutter app, you need to follow these steps:
2.1. Configure Firebase
Firebase Cloud Messaging (FCM) is a popular choice for sending push notifications in Flutter apps. Start by setting up a Firebase project and enabling FCM. Follow the Firebase documentation for detailed instructions.
2.2. Add Dependencies
In your pubspec.yaml file, add the necessary dependencies:
yaml dependencies: flutter: sdk: flutter firebase_core: ^latest_version firebase_messaging: ^latest_version
Run flutter pub get to install the dependencies.
2.3. Initialize Firebase
Initialize Firebase in your Flutter app by adding the following code in your main.dart:
dart import 'package:firebase_core/firebase_core.dart'; void main() async { WidgetsFlutterBinding.ensureInitialized(); await Firebase.initializeApp(); runApp(MyApp()); }
2.4. Request Permissions
Ask users for permission to send push notifications. You can use the firebase_messaging plugin to request permissions and handle user responses:
dart import 'package:firebase_messaging/firebase_messaging.dart'; FirebaseMessaging _firebaseMessaging = FirebaseMessaging.instance; void requestNotificationPermissions() async { NotificationSettings settings = await _firebaseMessaging.requestPermission( alert: true, badge: true, sound: true, ); if (settings.authorizationStatus == AuthorizationStatus.authorized) { print('User granted permission'); } else { print('User declined permission'); } }
2.5. Handle Notifications
To handle incoming notifications, add the following code to your main.dart:
dart void main() async { WidgetsFlutterBinding.ensureInitialized(); await Firebase.initializeApp(); requestNotificationPermissions(); // Request permissions FirebaseMessaging.onMessage.listen((RemoteMessage message) { print('Got a new message! Message data: ${message.data}'); }); runApp(MyApp()); }
With these steps, your Flutter app is now set up to receive and handle push notifications.
3. Sending Push Notifications
Now that your app is ready to receive push notifications, let’s explore how to send them from your server or Firebase console.
3.1. Sending from Firebase Console
The Firebase console provides an easy way to send notifications manually. Follow these steps:
- Go to the Firebase console.
- Select your project.
- Navigate to Cloud Messaging.
- Click on “Send your first message.”
- Compose your message, choose the target audience, and click “Next.”
3.2. Sending from Server
To send push notifications from your server, you can use the Firebase Admin SDK or a third-party push notification service. Here’s an example using the Firebase Admin SDK in Node.js:
javascript const admin = require('firebase-admin'); admin.initializeApp({ credential: admin.credential.applicationDefault(), databaseURL: 'https://your-project-id.firebaseio.com', }); const message = { notification: { title: 'Hello, Flutter!', body: 'Don't forget to check out our latest features.', }, topic: 'all', // Send to all users }; admin.messaging().send(message) .then((response) => { console.log('Successfully sent message:', response); }) .catch((error) => { console.log('Error sending message:', error); });
4. Best Practices for Engaging Users with Push Notifications
Implementing push notifications is just the beginning. To engage users effectively, you need to follow some best practices:
4.1. Personalization
Personalize your notifications based on user behavior and preferences. Send relevant content to each user to increase engagement.
4.2. Timing
Send notifications at the right time. Avoid sending them late at night or during busy hours when users are less likely to interact with them.
4.3. A/B Testing
Experiment with different notification messages, titles, and images to see what resonates best with your audience. A/B testing can help you optimize your notification strategy.
4.4. Frequency
Don’t overwhelm users with too many notifications. Find the right balance between staying top-of-mind and avoiding annoyance.
4.5. Deep Linking
Include deep links in your notifications to take users directly to a specific screen or feature within your app. This improves the user experience and encourages interaction.
5. Handling Notification Data
Push notifications can include additional data that you may want to handle in your Flutter app. For example, you might want to open a specific screen when a notification is tapped. Here’s how you can handle notification data:
dart FirebaseMessaging.onMessageOpenedApp.listen((RemoteMessage message) { // Handle when the app is already open print('A new onMessageOpenedApp event was published with data: ${message.data}'); }); dart void main() async { WidgetsFlutterBinding.ensureInitialized(); await Firebase.initializeApp(); requestNotificationPermissions(); FirebaseMessaging.onMessage.listen((RemoteMessage message) { // Handle when the app is in the foreground print('Got a new message while the app is in the foreground! Message data: ${message.data}'); }); FirebaseMessaging.onMessageOpenedApp.listen((RemoteMessage message) { // Handle when the app is opened from a terminated state print('Opened the app from a terminated state! Message data: ${message.data}'); }); runApp(MyApp()); }
Conclusion
Push notifications are a valuable tool for engaging users in your Flutter app. By implementing them effectively and following best practices, you can boost user engagement, retention, and overall satisfaction. Remember to personalize your notifications, send them at the right time, and continually test and optimize your strategy. With the right approach, push notifications can be a game-changer for your app’s success.
Incorporate push notifications into your Flutter app today and watch your user engagement soar!
Table of Contents
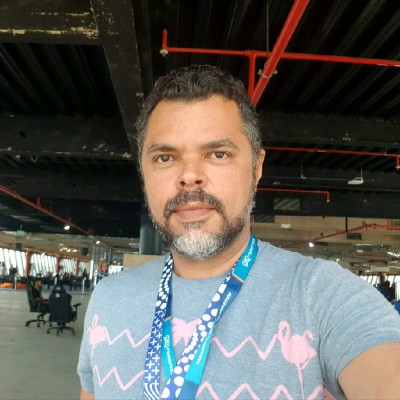
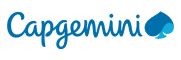