Responsive Design in Flutter: Adapting to Different Screen Sizes
In today’s dynamic digital landscape, users interact with applications across a wide range of devices and screen sizes. Ensuring a consistent and visually appealing user experience across these different dimensions is paramount for any successful app. This is where responsive design comes into play. Flutter, Google’s UI toolkit, offers a powerful framework for building natively compiled applications for mobile, web, and desktop from a single codebase. In this blog post, we will delve into the world of responsive design in Flutter, exploring techniques, best practices, and code examples that enable your app to seamlessly adapt to various screen sizes.
1. Understanding Responsive Design
Responsive design is an approach that allows web and app interfaces to respond and adapt to the user’s environment and behavior based on the screen size, platform, and orientation. In the context of Flutter, responsive design involves creating layouts that automatically adjust their appearance and behavior according to the available screen real estate. This ensures that users receive an optimal experience regardless of whether they’re using a small smartphone, a tablet, or a large desktop monitor.
2. MediaQuery and LayoutBuilder Widgets
Flutter provides two essential widgets for implementing responsive design: MediaQuery and LayoutBuilder.
2.1 MediaQuery
The MediaQuery widget enables you to retrieve information about the current app’s dimensions, orientation, and more. By accessing this information, you can dynamically adapt your app’s UI components.
dart Widget build(BuildContext context) { final screenWidth = MediaQuery.of(context).size.width; final screenHeight = MediaQuery.of(context).size.height; // Use screenWidth and screenHeight to adjust your UI elements // based on the available space. }
2.2 LayoutBuilder
The LayoutBuilder widget gives you the ability to respond to changes in layout constraints. It provides a callback with constraints that define the maximum available width and height for a widget. This is particularly useful when you want to create responsive layouts that adapt to different screen sizes.
dart Widget build(BuildContext context) { return LayoutBuilder( builder: (BuildContext context, BoxConstraints constraints) { if (constraints.maxWidth < 600) { // Return a layout suitable for small screens } else { // Return a layout suitable for larger screens } }, ); }
3. Flex and Expanded Widgets
Flutter offers the Flex and Expanded widgets that are incredibly useful for creating flexible layouts that adjust to available space.
3.1 Flex
The Flex widget is the foundation of many flexible layouts. It allows you to divide space within a container among its children based on their flex factors.
dart Flex( direction: Axis.horizontal, children: <Widget>[ Flexible( flex: 2, child: Container(color: Colors.blue), ), Flexible( flex: 3, child: Container(color: Colors.green), ), ], )
3.2 Expanded
The Expanded widget, when used as a child of a Row, Column, or Flex, takes up the available space along the main axis. This is particularly useful for ensuring that certain UI elements take up proportionate space on different screen sizes.
dart Column( children: <Widget>[ Expanded( flex: 2, child: Container(color: Colors.red), ), Expanded( flex: 3, child: Container(color: Colors.yellow), ), ], )
4. Using OrientationBuilder
Device orientation is another factor to consider when designing responsive layouts. Flutter provides the OrientationBuilder widget, allowing you to adjust your UI based on whether the device is in portrait or landscape mode.
dart OrientationBuilder( builder: (BuildContext context, Orientation orientation) { return orientation == Orientation.portrait ? // Return portrait layout : // Return landscape layout; }, )
5. Breakpoints and Adaptive Design
In responsive design, breakpoints are specific screen widths at which the layout of your app might change to better accommodate the available space. Flutter doesn’t have a predefined concept of breakpoints, but you can define your own based on your app’s design and target devices.
dart Widget build(BuildContext context) { final screenWidth = MediaQuery.of(context).size.width; if (screenWidth < 600) { // Return layout for small screens } else if (screenWidth < 1200) { // Return layout for medium screens } else { // Return layout for large screens } }
6. Testing Across Different Screen Sizes
To ensure the effectiveness of your responsive design, testing across various screen sizes and orientations is crucial. Flutter provides a robust set of tools for emulating different devices and orientations, such as the –device-id flag and the Flutter Device Preview package.
bash flutter run --device-id <device_id>
Conclusion
Creating a responsive Flutter app that caters to different screen sizes involves understanding the available tools and techniques at your disposal. By using widgets like MediaQuery, LayoutBuilder, Flex, and Expanded, and considering factors like orientation and breakpoints, you can design a UI that adapts gracefully to a diverse range of devices. Remember that the key to a successful responsive design lies in providing a seamless and enjoyable user experience across all platforms and screen sizes. Happy coding!
Incorporating responsive design principles into your Flutter app not only ensures a delightful user experience but also expands your app’s reach to a wider audience. By leveraging the power of Flutter’s layout and widget system, you can create adaptable interfaces that look and function flawlessly on devices of all shapes and sizes. Whether your users are on mobile phones, tablets, or desktops, a responsive design approach is your ticket to a successful and user-friendly app.
Table of Contents
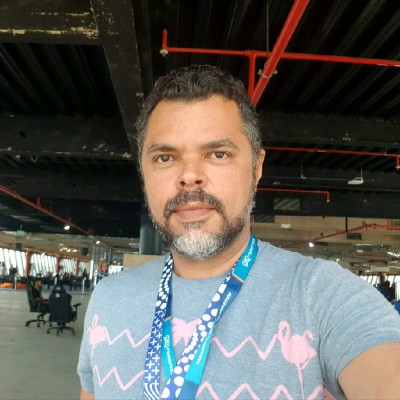
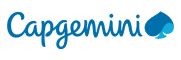