What is the purpose of the setState() method in Flutter?
The `setState()` method in Flutter is a crucial component for managing the state of a widget and triggering a rebuild of the user interface. In Flutter, the UI is constructed based on the current state of the widgets, and when that state changes, the UI needs to reflect those changes. This is where `setState()` comes into play.
When you call `setState()`, you provide a callback function that modifies the state of the widget. Flutter then schedules a rebuild of the widget subtree, making the necessary updates to the UI. This asynchronous rebuilding process is efficient, as it doesn’t block the main thread and ensures a smooth user experience.
Example:
```dart import 'package:flutter/material.dart'; class CounterApp extends StatefulWidget { @override _CounterAppState createState() => _CounterAppState(); } class _CounterAppState extends State<CounterApp> { int _counter = 0; void _incrementCounter() { setState(() { _counter++; }); } @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Counter App'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text('Counter Value: $_counter'), ElevatedButton( onPressed: _incrementCounter, child: Text('Increment'), ), ], ), ), ), ); } } void main() { runApp(CounterApp()); } ```
In this example, the `setState()` method is used within the `_incrementCounter` method. When the button is pressed, `_counter` is incremented within the `setState()` callback. This triggers a rebuild of the widget, updating the displayed counter value.
Key points:
- `setState()` is used to modify the state of a widget in Flutter.
- It triggers an asynchronous rebuild of the widget subtree.
- The UI is updated based on the modified state, ensuring a responsive user interface.
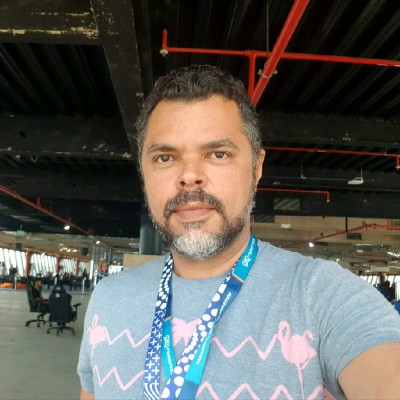
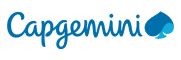