Using Flutter with SQLite: Offline Data Storage in Apps
In today’s fast-paced world, mobile applications have become an integral part of our lives. From social media platforms to productivity tools, the demand for feature-rich and responsive apps is ever-growing. However, the reliance on an uninterrupted internet connection can be a limiting factor for users, especially in areas with unstable connectivity. This is where offline data storage comes to the rescue. By seamlessly integrating offline capabilities into your app, you can enhance user experience and provide uninterrupted access to critical information. One of the most popular technologies for achieving this is by using Flutter with SQLite.
Table of Contents
1. Why Offline Data Storage Matters
Imagine you’re using a travel app to keep track of your itinerary and make bookings while on vacation. Suddenly, you find yourself in a location with poor internet connectivity, and the app becomes virtually unusable. This frustrating experience highlights the importance of offline data storage. Offline capabilities not only ensure uninterrupted access to data but also improve the overall reliability and performance of your app.
2. Introducing Flutter and SQLite
Flutter, developed by Google, is a powerful open-source UI framework that allows developers to create natively compiled applications for mobile, web, and desktop from a single codebase. SQLite, on the other hand, is a self-contained, serverless, and zero-configuration SQL database engine. It’s widely used for embedded database solutions due to its lightweight nature and efficient storage mechanisms.
The combination of Flutter and SQLite offers a robust solution for implementing offline data storage in your apps. Flutter’s rich widget library enables you to create intuitive user interfaces, while SQLite handles the storage and retrieval of data in offline mode.
3. Benefits of Using Flutter with SQLite for Offline Data Storage
- Efficient Data Management: SQLite databases are known for their efficiency in managing structured data. They provide indexing, querying, and sorting capabilities that allow your app to quickly retrieve and manipulate data, even when offline.
- Cross-Platform Consistency: Flutter’s “write once, run anywhere” philosophy ensures that your offline data storage solution works consistently across various platforms, including iOS, Android, and the web.
- Reduced Network Dependency: By storing essential data offline, your app can continue to function seamlessly even when there’s no internet connection. This is crucial for applications that involve real-time interactions or critical information access.
- Improved User Experience: Apps that can function offline offer a smoother and more enjoyable user experience. Users can continue browsing, performing actions, and accessing information without interruptions.
4. Implementing Offline Data Storage with Flutter and SQLite
Now, let’s dive into the practical steps of implementing offline data storage in your Flutter app using SQLite.
Step 1: Adding Dependencies
To get started, you need to include the necessary dependencies in your pubspec.yaml file:
yaml dependencies: flutter: sdk: flutter sqflite: ^2.0.0 path: ^2.0.0
The sqflite package provides the SQLite database support, while the path package helps with handling file paths.
Step 2: Creating the Database
Next, create a database helper class that manages the database connection and operations. Here’s a simplified example:
dart import 'package:sqflite/sqflite.dart'; import 'package:path/path.dart'; class DBHelper { static Database? _database; static Future<Database> get database async { if (_database != null) return _database!; _database = await initDatabase(); return _database!; } static Future<Database> initDatabase() async { final databasesPath = await getDatabasesPath(); final path = join(databasesPath, 'app_database.db'); return await openDatabase( path, version: 1, onCreate: (db, version) { db.execute(''' CREATE TABLE tasks ( id INTEGER PRIMARY KEY, title TEXT, description TEXT ) '''); }, ); } }
In this code, the DBHelper class handles database initialization and provides access to the database instance.
Step 3: Performing Database Operations
With the database set up, you can now perform various operations, such as adding, retrieving, updating, and deleting data.
dart class Task { final int id; final String title; final String description; Task({required this.id, required this.title, required this.description}); } class TaskDAO { final dbHelper = DBHelper(); Future<void> insertTask(Task task) async { final db = await dbHelper.database; await db.insert( 'tasks', task.toMap(), conflictAlgorithm: ConflictAlgorithm.replace, ); } Future<List<Task>> getAllTasks() async { final db = await dbHelper.database; final List<Map<String, dynamic>> maps = await db.query('tasks'); return List.generate(maps.length, (i) { return Task( id: maps[i]['id'], title: maps[i]['title'], description: maps[i]['description'], ); }); } }
In this example, the TaskDAO class provides methods for inserting and retrieving tasks from the database.
Step 4: Using the Offline Data
Now that you have the data stored offline, you can seamlessly integrate it into your app’s user interface, allowing users to access their data regardless of their internet connection status.
dart class TaskListScreen extends StatefulWidget { @override _TaskListScreenState createState() => _TaskListScreenState(); } class _TaskListScreenState extends State<TaskListScreen> { final taskDAO = TaskDAO(); late Future<List<Task>> tasks; @override void initState() { super.initState(); tasks = taskDAO.getAllTasks(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Task List')), body: FutureBuilder<List<Task>>( future: tasks, builder: (context, snapshot) { if (snapshot.connectionState == ConnectionState.waiting) { return CircularProgressIndicator(); } else if (snapshot.hasError) { return Text('Error: ${snapshot.error}'); } else { final taskList = snapshot.data ?? []; return ListView.builder( itemCount: taskList.length, itemBuilder: (context, index) { return ListTile( title: Text(taskList[index].title), subtitle: Text(taskList[index].description), ); }, ); } }, ), ); } }
This code demonstrates a basic Flutter screen that displays a list of tasks retrieved from the SQLite database. The FutureBuilder widget ensures that the data is loaded asynchronously.
5. Best Practices for Offline Data Storage
- Data Syncing: Implement a synchronization mechanism to update the local database with the server’s data whenever a network connection is available. This keeps the local data up-to-date and ensures consistency.
- Offline Indicator: Provide a clear visual indicator to users when the app is in offline mode. This helps manage user expectations and prevents confusion.
- Error Handling: Handle scenarios where network requests fail or there’s an issue with the local database. Inform users about the error gracefully and provide guidance on how to resolve it.
- Data Caching: Cache frequently accessed data locally to minimize the need for frequent network requests, even when the app is online. This enhances performance and reduces data consumption.
Conclusion
Integrating offline data storage into your Flutter app using SQLite opens up a world of possibilities for creating robust and user-friendly applications. By allowing users to access critical information and perform essential tasks without an active internet connection, you significantly enhance the value and reliability of your app. The combination of Flutter’s UI capabilities and SQLite’s efficient data storage mechanisms empowers developers to build apps that excel in both online and offline scenarios. So, whether your users are exploring remote areas or facing intermittent connectivity, your app remains a dependable companion.
Table of Contents
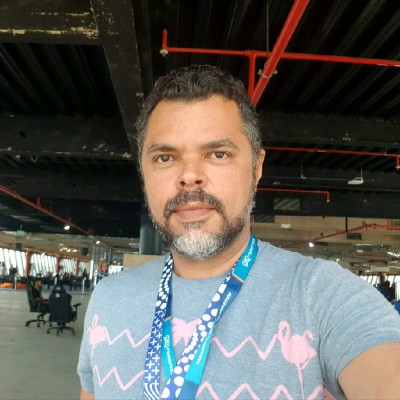
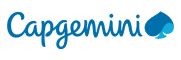