Explain the concept of StatelessWidget in Flutter.
In Flutter, a StatelessWidget is a fundamental building block of the user interface that, once instantiated, cannot be modified. Its primary purpose is to represent parts of the user interface that remain static and do not change over time. As the name implies, a StatelessWidget does not maintain any internal state, making it immutable.
One key characteristic of a StatelessWidget is that its appearance is solely determined by its configuration and any external information provided to it. This makes it an ideal choice for UI elements that don’t need to change dynamically based on user interactions or other factors.
Let’s take a simple example to illustrate the concept. Consider a Flutter application with a static text widget displaying a welcome message. This text widget would be implemented as a StatelessWidget since the message doesn’t change during the app’s runtime:
```dart import 'package:flutter/material.dart'; class WelcomeMessage extends StatelessWidget { @override Widget build(BuildContext context) { return Container( child: Text( 'Welcome to Flutter!', style: TextStyle(fontSize: 20), ), ); } } void main() { runApp(MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Flutter StatelessWidget Example'), ), body: Center( child: WelcomeMessage(), ), ), )); } ```
In this example, the `WelcomeMessage` class extends `StatelessWidget`, and its `build` method returns a static text widget. Since the message doesn’t change, there’s no need for internal state or mutable properties.
A StatelessWidget is a crucial element in Flutter for creating static and unchanging parts of the user interface, promoting a clean and efficient separation of concerns in the application’s architecture.
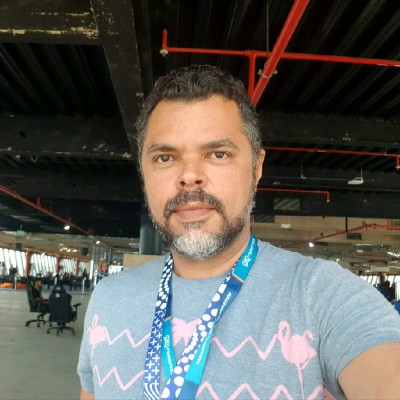
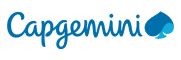