Testing and Debugging in Flutter: Best Practices and Tools
Building a Flutter application is an exciting journey that involves creating captivating user interfaces and seamless user experiences. However, with great power comes great responsibility, and ensuring that your Flutter app functions correctly requires rigorous testing and effective debugging. In this guide, we will delve into the world of testing and debugging in Flutter, uncovering the best practices and tools that can help you create robust and reliable applications.
1. Why Testing and Debugging Matter
Before we dive into the best practices and tools, let’s establish why testing and debugging are crucial aspects of Flutter app development.
1.1. Reliable Functionality
Flutter apps can run on various devices and platforms, each with its unique set of characteristics. Ensuring that your app works consistently across these platforms is essential to provide a reliable user experience. Comprehensive testing helps identify and fix issues that might arise on specific devices or operating systems.
1.2. User Satisfaction
Imagine a scenario where your app crashes or exhibits unexpected behavior in front of users. Such situations can lead to frustration and negative reviews, ultimately driving users away. By thoroughly testing your app and diligently debugging issues, you can deliver a polished and satisfying user experience.
2. Testing Best Practices
2.1. Unit Testing
Unit testing involves testing individual components or functions in isolation to ensure they behave as expected. In Flutter, the test package offers a robust framework for writing unit tests.
dart // Example of a simple unit test in Flutter import 'package:flutter_test/flutter_test.dart'; int add(int a, int b) { return a + b; } void main() { test('Adding two numbers', () { expect(add(2, 3), equals(5)); }); }
2.2. Widget Testing
Flutter’s widget testing allows you to test UI components by simulating user interactions and verifying the resulting changes. This ensures that your widgets render correctly and respond appropriately to user actions.
dart // Example of a widget test in Flutter import 'package:flutter/material.dart'; import 'package:flutter_test/flutter_test.dart'; void main() { testWidgets('Counter increments', (WidgetTester tester) async { await tester.pumpWidget(MyApp()); expect(find.text('0'), findsOneWidget); expect(find.text('1'), findsNothing); await tester.tap(find.byIcon(Icons.add)); await tester.pump(); expect(find.text('0'), findsNothing); expect(find.text('1'), findsOneWidget); }); }
2.3. Integration Testing
Integration tests assess how different parts of your app work together. These tests ensure that various components integrate seamlessly, from UI widgets to backend logic.
dart // Example of an integration test in Flutter import 'package:flutter_test/flutter_test.dart'; import 'package:my_app/main.dart' as app; void main() { testWidgets('App loads and displays text', (WidgetTester tester) async { // Build our app and trigger a frame. await tester.pumpWidget(app.MyApp()); // Verify that the initial text is displayed. expect(find.text('Hello, Flutter!'), findsOneWidget); }); }
3. Debugging Strategies
3.1. Logging
Strategically placed log statements can provide valuable insights into your app’s behavior. Use print() statements to output information about variable values, function calls, and control flow.
dart void calculateTotalPrice(List<double> prices) { double total = 0; for (double price in prices) { total += price; print('Adding $price to total. Total: $total'); } }
3.2. Debugging Tools
Flutter offers a powerful set of debugging tools that can help you diagnose issues more effectively. The Flutter DevTools suite provides insights into widget trees, performance profiles, and memory usage.
3.3. Hot Reload and Hot Restart
Leverage Flutter’s hot reload and hot restart features to quickly see the impact of your code changes. Hot reload applies changes to your running app, maintaining its state. Hot restart rebuilds the entire app, which can help resolve more complex issues.
4. Effective Debugging Tools
4.1. Flutter DevTools
Flutter DevTools is a comprehensive suite of debugging tools that offers insights into various aspects of your app’s performance and behavior. It includes:
- Widget Inspector: Visualize your widget tree and identify UI issues.
- Timeline: Analyze your app’s rendering and frame behavior.
- CPU Profiler: Identify performance bottlenecks in your code.
- Memory Profiler: Detect and resolve memory leaks.
4.2. Dart Observatory
Dart Observatory is a debugging tool that provides insights into your Dart code’s runtime behavior. It helps you monitor variables, view stack traces, and understand memory allocation.
5. Continuous Integration and Delivery (CI/CD)
5.1. Automated Testing
Integrate automated testing into your CI/CD pipeline to ensure that your app is tested thoroughly before deployment. Tools like Jenkins, Travis CI, and CircleCI can help automate your testing process.
5.2. Continuous Delivery
Automate the deployment process to ensure smooth and consistent updates to your app. Continuous delivery tools like Fastlane can help streamline app distribution.
Conclusion
Testing and debugging are fundamental aspects of creating successful Flutter applications. By following best practices and leveraging powerful debugging tools, you can identify and address issues before they reach your users, providing a seamless and delightful user experience. Remember, investing time in testing and debugging now can save you from headaches down the road. So, keep testing, keep debugging, and keep building amazing Flutter apps!
In this guide, we’ve only scratched the surface of testing and debugging in Flutter. As you continue your Flutter journey, don’t hesitate to explore more advanced testing techniques and dive deeper into debugging tools to become a true Flutter debugging and testing expert. Happy coding!
Table of Contents
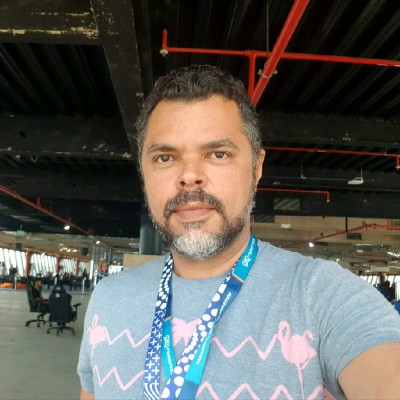
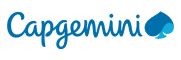