Exploring Flutter’s Testing Frameworks: Ensuring App Quality
Ensuring the quality of mobile applications is paramount, especially in today’s competitive app market. Flutter, Google’s UI toolkit for building natively compiled applications, offers robust testing frameworks to help developers maintain high standards. This article explores how to use Flutter’s testing tools to create reliable and performant apps, providing practical examples of unit testing, widget testing, and integration testing.
Why Testing is Crucial in Flutter Development
Testing is an integral part of the software development lifecycle, ensuring that apps function as expected across different devices and scenarios. In Flutter, testing is particularly important due to the framework’s ability to create cross-platform applications with a single codebase. This means that a single issue could affect multiple platforms if not caught early. Flutter’s testing tools help mitigate this risk by providing thorough and efficient ways to test your app at various levels.
Flutter Testing Frameworks Overview
Flutter offers three primary types of testing: unit testing, widget testing, and integration testing. Each serves a distinct purpose and can be used in combination to ensure a well-rounded testing strategy.
1. Unit Testing
Unit testing in Flutter focuses on testing individual functions, methods, or classes in isolation. This type of testing is essential for verifying the correctness of your business logic and ensuring that each component behaves as expected.
Example: Testing a Dart Function
Consider a simple function that calculates the sum of two integers. Here’s how you might write a unit test for it.
```dart import 'package:flutter_test/flutter_test.dart'; int add(int a, int b) => a + b; void main() { test('add two numbers', () { expect(add(2, 3), 5); }); } ```
This basic test ensures that the `add` function behaves correctly by checking if the sum of 2 and 3 equals 5.
2. Widget Testing
Widget testing (sometimes referred to as component testing) is used to verify that individual widgets function as intended. In Flutter, widgets are the building blocks of your UI, so it’s crucial to ensure they render and interact correctly.
Example: Testing a Flutter Widget
Here’s how you might write a widget test to verify that a button triggers a specific action.
```dart import 'package:flutter/material.dart'; import 'package:flutter_test/flutter_test.dart'; void main() { testWidgets('MyWidget has a title and button', (WidgetTester tester) async { // Build the widget await tester.pumpWidget(MaterialApp( home: Scaffold( appBar: AppBar(title: const Text('My Widget')), body: Column( children: [ const Text('Hello, World!'), ElevatedButton( onPressed: () {}, child: const Text('Press me'), ), ], ), ), )); // Verify the title is displayed expect(find.text('My Widget'), findsOneWidget); // Verify the button is displayed and works expect(find.text('Press me'), findsOneWidget); await tester.tap(find.byType(ElevatedButton)); await tester.pump(); // Rebuilds the widget after the button is pressed }); } ```
This test checks if the widget renders correctly with the expected text and if the button can be tapped.
3. Integration Testing
Integration testing in Flutter involves testing a complete app or a large part of it, ensuring that different components work together as expected. This type of testing is particularly useful for simulating real-world scenarios.
Example: Writing an Integration Test
Here’s an example of a simple integration test that verifies the app’s navigation flow.
```dart import 'package:flutter_test/flutter_test.dart'; import 'package:integration_test/integration_test.dart'; import 'package:my_app/main.dart' as app; void main() { IntegrationTestWidgetsFlutterBinding.ensureInitialized(); testWidgets('App navigates to second screen', (WidgetTester tester) async { app.main(); await tester.pumpAndSettle(); // Verify the first screen is shown expect(find.text('Home Screen'), findsOneWidget); // Tap the button to navigate to the second screen await tester.tap(find.text('Go to Second Screen')); await tester.pumpAndSettle(); // Verify the second screen is shown expect(find.text('Second Screen'), findsOneWidget); }); } ```
This integration test ensures that the app successfully navigates from the home screen to the second screen when the button is tapped.
Combining Testing Strategies
By combining unit, widget, and integration tests, you can cover all aspects of your Flutter application, ensuring both the individual components and the entire app function as expected. This comprehensive testing approach minimizes the risk of bugs and improves the overall reliability of your app.
Conclusion
Flutter’s testing frameworks provide developers with the tools needed to ensure their apps are of the highest quality. Whether you’re performing unit tests to verify your business logic, widget tests to check UI components, or integration tests to simulate real-world usage, Flutter has you covered. By leveraging these tools, you can build more robust, reliable, and user-friendly applications.
Further Reading
Table of Contents
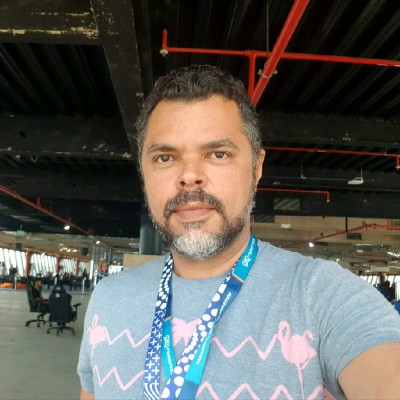
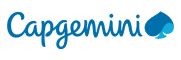