Handling User Input in Flutter: Forms and Validation
User input is a fundamental aspect of mobile app development. Whether you’re building a login screen, a registration form, or a settings page, you need to collect and process data entered by users. In Flutter, a powerful and versatile framework for building natively compiled applications, handling user input is made efficient and straightforward through forms and validation.
Table of Contents
In this comprehensive guide, we’ll dive into the world of Flutter forms and explore best practices for input validation. We’ll cover everything from creating simple input fields to implementing complex forms with custom validation logic. By the end of this tutorial, you’ll have the skills to build user-friendly and error-resistant Flutter apps.
1. Getting Started with Forms in Flutter
1.1. Creating a Basic Form
Forms in Flutter are created using the Form widget, which acts as a container for various form fields. Let’s start with a simple example of a login form that collects a username and password.
dart import 'package:flutter/material.dart'; class LoginForm extends StatefulWidget { @override _LoginFormState createState() => _LoginFormState(); } class _LoginFormState extends State<LoginForm> { final _formKey = GlobalKey<FormState>(); @override Widget build(BuildContext context) { return Form( key: _formKey, child: Column( children: [ // Username Field TextFormField( decoration: InputDecoration(labelText: 'Username'), validator: (value) { if (value.isEmpty) { return 'Please enter your username.'; } return null; }, ), // Password Field TextFormField( decoration: InputDecoration(labelText: 'Password'), obscureText: true, validator: (value) { if (value.isEmpty) { return 'Please enter your password.'; } return null; }, ), // Login Button ElevatedButton( onPressed: () { if (_formKey.currentState.validate()) { // Form is valid, proceed with login } }, child: Text('Login'), ), ], ), ); } }
In this example, we’ve created a LoginForm widget that includes a Form widget containing two TextFormField widgets for the username and password. We’ve also added basic validation for these fields using the validator property.
1.2. Adding Text Input Fields
The TextFormField widget is an essential element in collecting user input. It provides various properties to customize the input field’s appearance and behavior. In our login form example, we used the decoration property to add labels and the obscureText property to hide the password’s characters.
Now that we’ve created a basic form, let’s move on to form validation.
2. Form Validation in Flutter
2.1. Built-in Validators
Flutter provides a set of built-in validators that can be used with TextFormField. These validators can check for common input requirements like non-empty fields, valid email addresses, and numeric input. Here’s an example of using a built-in validator to require a valid email address:
dart TextFormField( decoration: InputDecoration(labelText: 'Email'), validator: (value) { if (value.isEmpty) { return 'Please enter your email address.'; } if (!RegExp(r'^[\w-]+(\.[\w-]+)*@([\w-]+\.)+[a-zA-Z]{2,7}
In the above code, we use the RegExp class to define a regular expression pattern for a valid email address. If the input value is empty or doesn’t match the pattern, the validator returns an error message.
2.2. Custom Validation Logic
While built-in validators cover many cases, you’ll often need custom validation logic to enforce specific rules or validate against external data sources. For instance, you might want to check if a username is already taken during registration. Here’s how you can implement custom validation:
dart TextFormField( decoration: InputDecoration(labelText: 'Username'), validator: (value) { if (value.isEmpty) { return 'Please enter your username.'; } if (/* Check if username is already taken */) { return 'Username is already taken.'; } return null; }, )
In this example, you would replace the comment with code to check if the username is already in use, potentially by making an API call or querying a database.
2.3. Displaying Validation Errors
To display validation errors to the user, you can utilize the Text widget positioned beneath the corresponding TextFormField. Here’s how to display validation errors for our username field:
dart TextFormField( decoration: InputDecoration(labelText: 'Username'), validator: (value) { if (value.isEmpty) { return 'Please enter your username.'; } if (/* Check if username is already taken */) { return 'Username is already taken.'; } return null; }, ), Text( // Display the validation error message here style: TextStyle(color: Colors.red), )
The Text widget displays the validation error message in red text if the validator returns an error message (non-null value).
Now that you’ve learned the basics of form validation, let’s explore some advanced form handling techniques.
3. Advanced Form Handling Techniques
3.1. Form Submission
Once the form is validated, you’ll want to handle the form submission, which typically involves sending the user’s input to a server or performing some action based on the input. You can do this by adding an onPressed callback to a button within the form:
dart ElevatedButton( onPressed: () { if (_formKey.currentState.validate()) { // Form is valid, proceed with submission final username = /* Get the username from the form */; final password = /* Get the password from the form */; // Perform submission logic here } }, child: Text('Submit'), )
In the onPressed callback, you can access the validated form data and proceed with the submission logic.
3.2. Resetting a Form
Sometimes, you may need to allow users to reset the form to its initial state. To achieve this, you can create a function that resets the form’s state and call it when needed:
dart void _resetForm() { _formKey.currentState.reset(); }
You can then call _resetForm in response to a button press or any other user action.
3.3. Handling Form State
Understanding and managing the state of a form is crucial for a smooth user experience. The Form widget automatically manages the state of its form fields and validators. However, if you need to access or manipulate the form state programmatically, you can use the FormState object obtained through the GlobalKey<FormState>.
dart final _formKey = GlobalKey<FormState>(); ... Form( key: _formKey, child: ... ) ... void _submitForm() { if (_formKey.currentState.validate()) { // Access and manipulate the form state _formKey.currentState.save(); } }
In this example, _formKey.currentState allows you to save the form data, which can be useful if you want to persist or display it elsewhere in your app.
4. Best Practices for User-Friendly Forms
4.1. Input Field Styling
A well-designed form not only functions correctly but also looks appealing. Flutter provides extensive customization options for input fields through the InputDecoration class. You can style text input fields, labels, and error messages to match your app’s design language.
dart TextFormField( decoration: InputDecoration( labelText: 'Email', hintText: 'Enter your email', prefixIcon: Icon(Icons.email), border: OutlineInputBorder(), errorStyle: TextStyle(fontSize: 14), ), validator: (value) { if (value.isEmpty) { return 'Please enter your email address.'; } // Additional validation logic return null; }, )
4.2. Keyboard and Focus Management
For a smoother user experience, consider managing the keyboard and focus. You can programmatically show or hide the keyboard and control which input field has focus using the FocusNode and TextFormField properties like autofocus.
dart FocusNode _emailFocusNode = FocusNode(); ... TextFormField( decoration: InputDecoration(labelText: 'Email'), focusNode: _emailFocusNode, validator: (value) { if (value.isEmpty) { return 'Please enter your email address.'; } return null; }, )
You can use _emailFocusNode.requestFocus() to programmatically focus on the email field, making the user experience more intuitive.
4.3. Error Messaging
Clear and concise error messages are essential for helping users understand and correct their input errors. Ensure that your error messages are informative and displayed prominently. Consider using Flutter’s SnackBar or a dedicated error message area to convey validation errors to the user.
dart ScaffoldMessenger.of(context).showSnackBar( SnackBar( content: Text('Please correct the form errors.'), ), );
Conclusion
Handling user input in Flutter forms and implementing validation logic is a crucial skill for building robust and user-friendly apps. With the knowledge you’ve gained in this guide, you can create forms that collect data efficiently, validate it effectively, and provide a seamless user experience.
Remember that user input can vary, so always adapt your forms and validation to match the specific requirements of your app. By following best practices and making good use of Flutter’s features, you’ll be well-equipped to create delightful user interfaces and ensure data accuracy in your Flutter applications. Start building your next great app today!
).hasMatch(value)) { return 'Please enter a valid email address.'; } return null; }, )
In the above code, we use the RegExp class to define a regular expression pattern for a valid email address. If the input value is empty or doesn’t match the pattern, the validator returns an error message.
2.2. Custom Validation Logic
While built-in validators cover many cases, you’ll often need custom validation logic to enforce specific rules or validate against external data sources. For instance, you might want to check if a username is already taken during registration. Here’s how you can implement custom validation:
In this example, you would replace the comment with code to check if the username is already in use, potentially by making an API call or querying a database.
2.3. Displaying Validation Errors
To display validation errors to the user, you can utilize the Text widget positioned beneath the corresponding TextFormField. Here’s how to display validation errors for our username field:
The Text widget displays the validation error message in red text if the validator returns an error message (non-null value).
Now that you’ve learned the basics of form validation, let’s explore some advanced form handling techniques.
3. Advanced Form Handling Techniques
3.1. Form Submission
Once the form is validated, you’ll want to handle the form submission, which typically involves sending the user’s input to a server or performing some action based on the input. You can do this by adding an onPressed callback to a button within the form:
In the onPressed callback, you can access the validated form data and proceed with the submission logic.
3.2. Resetting a Form
Sometimes, you may need to allow users to reset the form to its initial state. To achieve this, you can create a function that resets the form’s state and call it when needed:
You can then call _resetForm in response to a button press or any other user action.
3.3. Handling Form State
Understanding and managing the state of a form is crucial for a smooth user experience. The Form widget automatically manages the state of its form fields and validators. However, if you need to access or manipulate the form state programmatically, you can use the FormState object obtained through the GlobalKey<FormState>.
In this example, _formKey.currentState allows you to save the form data, which can be useful if you want to persist or display it elsewhere in your app.
4. Best Practices for User-Friendly Forms
4.1. Input Field Styling
A well-designed form not only functions correctly but also looks appealing. Flutter provides extensive customization options for input fields through the InputDecoration class. You can style text input fields, labels, and error messages to match your app’s design language.
4.2. Keyboard and Focus Management
For a smoother user experience, consider managing the keyboard and focus. You can programmatically show or hide the keyboard and control which input field has focus using the FocusNode and TextFormField properties like autofocus.
You can use _emailFocusNode.requestFocus() to programmatically focus on the email field, making the user experience more intuitive.
4.3. Error Messaging
Clear and concise error messages are essential for helping users understand and correct their input errors. Ensure that your error messages are informative and displayed prominently. Consider using Flutter’s SnackBar or a dedicated error message area to convey validation errors to the user.
Conclusion
Handling user input in Flutter forms and implementing validation logic is a crucial skill for building robust and user-friendly apps. With the knowledge you’ve gained in this guide, you can create forms that collect data efficiently, validate it effectively, and provide a seamless user experience.
Remember that user input can vary, so always adapt your forms and validation to match the specific requirements of your app. By following best practices and making good use of Flutter’s features, you’ll be well-equipped to create delightful user interfaces and ensure data accuracy in your Flutter applications. Start building your next great app today!
Table of Contents
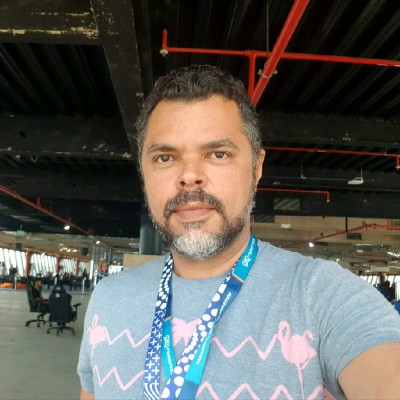
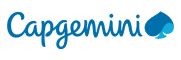