Creating a Wallpaper App with Flutter: Image APIs and Grid Layouts
Flutter has become a popular choice for building cross-platform apps thanks to its fast development capabilities and expressive UI. When it comes to creating a wallpaper app, Flutter’s rich widget library and robust support for HTTP requests make it an excellent choice. This blog will guide you through using Flutter to build a wallpaper app, focusing on integrating image APIs and implementing a grid layout to showcase images beautifully.
Understanding the Basics of Wallpaper Apps
Wallpaper apps are designed to provide users with a variety of images that they can set as backgrounds on their devices. These apps typically involve fetching images from a server, displaying them in a grid or list, and allowing users to select and apply their favorite wallpapers. Flutter simplifies the process with its powerful widgets and seamless integration with various APIs.
Using Flutter to Create a Wallpaper App
Flutter provides an array of tools and libraries that make building a wallpaper app straightforward. Below are key aspects and code examples to help you get started.
1. Fetching Images from an API
The first step in creating a wallpaper app is to fetch images from an API. For this example, we’ll use the Unsplash API, which offers high-quality images for free.
Example: Fetching Images from Unsplash API
Add the `http` package to your `pubspec.yaml` file:
```yaml dependencies: flutter: sdk: flutter http: ^0.14.0 ```
Then, create a function to fetch images:
```dart import 'dart:convert'; import 'package:http/http.dart' as http; Future<List<String>> fetchImages() async { final response = await http.get(Uri.parse('https://api.unsplash.com/photos/?client_id=YOUR_ACCESS_KEY')); if (response.statusCode == 200) { List<dynamic> data = jsonDecode(response.body); return data.map((image) => image['urls']['regular']).toList(); } else { throw Exception('Failed to load images'); } } ```
2. Displaying Images in a Grid Layout
A grid layout is ideal for displaying a collection of images. Flutter’s `GridView` widget is perfect for this purpose.
Example: Creating a Grid Layout
Here’s how you might use `GridView` to display images:
```dart import 'package:flutter/material.dart'; class WallpaperApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar(title: Text('Wallpaper App')), body: FutureBuilder<List<String>>( future: fetchImages(), builder: (context, snapshot) { if (snapshot.connectionState == ConnectionState.waiting) { return Center(child: CircularProgressIndicator()); } else if (snapshot.hasError) { return Center(child: Text('Error: ${snapshot.error}')); } else { List<String> images = snapshot.data!; return GridView.builder( gridDelegate: SliverGridDelegateWithFixedCrossAxisCount( crossAxisCount: 2, crossAxisSpacing: 4.0, mainAxisSpacing: 4.0, ), itemCount: images.length, itemBuilder: (context, index) { return Image.network(images[index], fit: BoxFit.cover); }, ); } }, ), ), ); } } ```
3. Implementing Image Selection and Setting Wallpaper
To allow users to select an image and set it as their wallpaper, you’ll need to handle image selection and possibly integrate with platform-specific APIs to set the wallpaper.
Example: Handling Image Selection
Here’s a simple way to handle image selection:
```dart import 'package:flutter/material.dart'; import 'package:fluttertoast/fluttertoast.dart'; class WallpaperApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar(title: Text('Wallpaper App')), body: FutureBuilder<List<String>>( future: fetchImages(), builder: (context, snapshot) { if (snapshot.connectionState == ConnectionState.waiting) { return Center(child: CircularProgressIndicator()); } else if (snapshot.hasError) { return Center(child: Text('Error: ${snapshot.error}')); } else { List<String> images = snapshot.data!; return GridView.builder( gridDelegate: SliverGridDelegateWithFixedCrossAxisCount( crossAxisCount: 2, crossAxisSpacing: 4.0, mainAxisSpacing: 4.0, ), itemCount: images.length, itemBuilder: (context, index) { return GestureDetector( onTap: () { Fluttertoast.showToast( msg: 'Selected image: ${images[index]}', toastLength: Toast.LENGTH_SHORT, ); // Implement wallpaper setting functionality here }, child: Image.network(images[index], fit: BoxFit.cover), ); }, ); } }, ), ), ); } } ```
Conclusion
Flutter provides a powerful platform for building a wallpaper app with its easy-to-use widgets and robust support for API integrations. By leveraging Flutter’s `GridView` for layout and handling image data with HTTP requests, you can create a visually appealing and functional wallpaper app. With these capabilities, you can deliver a seamless and engaging experience for your users.
Further Reading:
Table of Contents
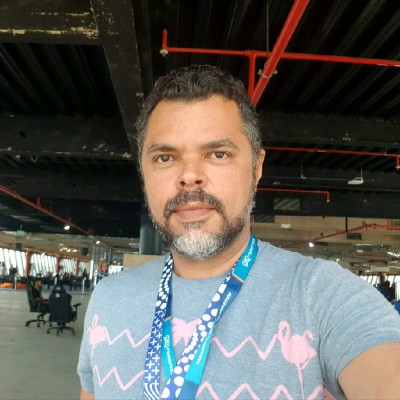
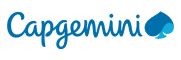